Model deployment#
One of the main goals of PyMC-Marketing is to facilitate the deployment of its models.
This is achieved by building our models on top of ModelBuilder, a brand new PyMC experimental feature that offers a scikit-learn-like API and makes PyMC models easy to deploy.
PyMC-marketing models inherit 2 easy-to-use methods: save
and load
that can be used after the model has been fitted. All models can be configured with two standard dictionaries: model_config
and sampler_config
that are serialized during save
and persisted after load
, allowing model reuse across workflows.
We will illustrate this functionality with the example model described in the MMM Example Notebook. For sake of generality, we ommit most technical details here.
import arviz as az
import numpy as np
import pandas as pd
from pymc_marketing.mmm import DelayedSaturatedMMM
seed = sum(map(ord, "mmm"))
rng = np.random.default_rng(seed=seed)
Let’s load the dataset:
url = "https://raw.githubusercontent.com/pymc-labs/pymc-marketing/main/data/mmm_example.csv"
df = pd.read_csv(url)
columns_to_keep = [
"date_week",
"y",
"x1",
"x2",
"event_1",
"event_2",
"dayofyear",
]
data = df[columns_to_keep].copy()
data["t"] = np.arange(df.shape[0])
data.head()
date_week | y | x1 | x2 | event_1 | event_2 | dayofyear | t | |
---|---|---|---|---|---|---|---|---|
0 | 2018-04-02 | 3984.662237 | 0.318580 | 0.0 | 0.0 | 0.0 | 92 | 0 |
1 | 2018-04-09 | 3762.871794 | 0.112388 | 0.0 | 0.0 | 0.0 | 99 | 1 |
2 | 2018-04-16 | 4466.967388 | 0.292400 | 0.0 | 0.0 | 0.0 | 106 | 2 |
3 | 2018-04-23 | 3864.219373 | 0.071399 | 0.0 | 0.0 | 0.0 | 113 | 3 |
4 | 2018-04-30 | 4441.625278 | 0.386745 | 0.0 | 0.0 | 0.0 | 120 | 4 |
But for our model we need much smaller dataset, many of the previous features were contributing to generation of others, now as our target variable is computed we can filter out not needed columns:
Model and sampling configuration#
Model configuration#
We first illustrate the use of model_config
to define custom priors within the model.
Because there are potentially many variables that can be configured, each model provides a default_model_config
attribute. This will allow you to see which settings are available by default and only define the ones you need to change.
We need to create a dummy model to be able to see the configuration dictionary.
dummy_model = DelayedSaturatedMMM(
date_column="date_week",
channel_columns=["x1", "x2"],
control_columns=[
"event_1",
"event_2",
"t",
],
adstock_max_lag=8,
yearly_seasonality=2,
)
dummy_model.default_model_config
{'intercept': {'mu': 0, 'sigma': 2},
'beta_channel': {'sigma': 2, 'dims': ('channel',)},
'alpha': {'alpha': 1, 'beta': 3, 'dims': ('channel',)},
'lam': {'alpha': 3, 'beta': 1, 'dims': ('channel',)},
'sigma': {'sigma': 2},
'gamma_control': {'mu': 0, 'sigma': 2, 'dims': ('control',)},
'mu': {'dims': ('date',)},
'likelihood': {'dims': ('date',)},
'gamma_fourier': {'mu': 0, 'b': 1, 'dims': 'fourier_mode'}}
We can change the parameters that go into the distribution of each term.
In this case we’ll just simply replace the sigma
for beta_channel
with a custom one:
n_channels = 2
total_spend_per_channel = data[["x1", "x2"]].sum(axis=0)
spend_share = total_spend_per_channel / total_spend_per_channel.sum()
spend_share
# The scale necessary to make a HalfNormal distribution have unit variance
HALFNORMAL_SCALE = 1 / np.sqrt(1 - 2 / np.pi)
prior_sigma = HALFNORMAL_SCALE * n_channels * spend_share.to_numpy()
prior_sigma
array([2.1775326 , 1.14026088])
my_model_config = {'beta_channel': {'sigma': prior_sigma, 'dims': ('channel',)}}
As mentioned in the original notebook: “For the prior specification there is no right or wrong answer. It all depends on the data, the context and the assumptions you are willing to make. It is always recommended to do some prior predictive sampling and sensitivity analysis to check the impact of the priors on the posterior. We skip this here for the sake of simplicity. If you are not sure about specific priors, the DelayedSaturatedMMM
class has some default priors that you can use as a starting point.”
Sampling configuration#
The second feature we can customize is sampler_config
. Similar to model_config
, it’s a dictionary that gets saved and contains things you would usually pass to the fit()
kwargs. It’s not mandatory to create your own sampler_config
. The default DelayedSaturatedMMM.sampler_config
is empty because the default sampling parameters usually prove sufficient for a start.
dummy_model.default_sampler_config
{}
my_sampler_config = {
'tune':1000,
'draws':1000,
'chains':4,
'target_accept':0.95,
}
Let’s finally assemble our model!
mmm = DelayedSaturatedMMM(
model_config = my_model_config,
sampler_config = my_sampler_config,
date_column="date_week",
channel_columns=["x1", "x2"],
control_columns=[
"event_1",
"event_2",
"t",
],
adstock_max_lag=8,
yearly_seasonality=2,
)
We can confirm our settings are being used
mmm.model_config["beta_channel"]
{'sigma': array([2.1775326 , 1.14026088]), 'dims': ('channel',)}
mmm.sampler_config
{'tune': 1000, 'draws': 1000, 'chains': 4, 'target_accept': 0.95}
Model Fitting#
Note that we didn’t pass the dataset to the class constructor itself. This is done to mimick the scikit-learn
API, and make it easier to get started on PyMC-Marketing models.
# Split X, and y
X = data.drop('y',axis=1)
y = data['y']
All that’s left now is to finally fit the model:
As you can see below, you can still pass the sampler kwargs directly to fit()
method. However, only those kwargs passed using sampler_config
will be saved and reused after loading the model.
mmm.fit(X=X, y=y, random_seed=rng)
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
Multiprocess sampling (4 chains in 4 jobs)
NUTS: [intercept, beta_channel, alpha, lam, sigma, gamma_control, gamma_fourier]
Sampling 4 chains for 1_000 tune and 1_000 draw iterations (4_000 + 4_000 draws total) took 71 seconds.
Sampling: [alpha, beta_channel, gamma_control, gamma_fourier, intercept, lam, likelihood, sigma]
Sampling: [likelihood]
-
- chain: 4
- draw: 1000
- control: 3
- fourier_mode: 4
- channel: 2
- date: 179
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- control(control)<U7'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype='<U7')
- fourier_mode(fourier_mode)<U11'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='<U11')
- channel(channel)<U2'x1' 'x2'
array(['x1', 'x2'], dtype='<U2')
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- intercept(chain, draw)float640.3852 0.3278 ... 0.3526 0.311
array([[0.3851927 , 0.3278435 , 0.36165071, ..., 0.34388561, 0.35443253, 0.35253033], [0.34981775, 0.34584928, 0.35525882, ..., 0.35523169, 0.35908122, 0.34575935], [0.35563579, 0.3451598 , 0.35325558, ..., 0.34084824, 0.3358194 , 0.34582752], [0.35233725, 0.34990925, 0.33671441, ..., 0.33613902, 0.35255676, 0.31100003]])
- gamma_control(chain, draw, control)float640.2353 0.335 ... 0.3376 0.0006672
array([[[0.23532676, 0.33500538, 0.0005746 ], [0.25416368, 0.32078771, 0.00061704], [0.25503385, 0.34825216, 0.00063763], ..., [0.27940618, 0.36824443, 0.00063042], [0.20458129, 0.29476204, 0.0005926 ], [0.20935657, 0.30461187, 0.00064154]], [[0.24481066, 0.38185725, 0.00065845], [0.22396253, 0.31905371, 0.00063923], [0.2644513 , 0.29687398, 0.00059563], ..., [0.29669276, 0.32514522, 0.00065721], [0.28999838, 0.34466694, 0.00065541], [0.23563995, 0.32485093, 0.00053246]], [[0.25429729, 0.34677501, 0.00058459], [0.22986879, 0.33982102, 0.00061778], [0.22342951, 0.29270982, 0.00066354], ..., [0.25601694, 0.34311046, 0.00065063], [0.25895209, 0.29189666, 0.00062944], [0.22955645, 0.32483543, 0.00064999]], [[0.24109677, 0.31853461, 0.00058352], [0.29313553, 0.34149225, 0.00061645], [0.22163006, 0.31875355, 0.00068093], ..., [0.24132451, 0.354876 , 0.00061514], [0.25669935, 0.33493294, 0.0006427 ], [0.25828094, 0.33763074, 0.00066721]]])
- gamma_fourier(chain, draw, fourier_mode)float640.004958 0.06203 ... 0.002304
array([[[ 4.95809628e-03, 6.20279726e-02, -5.71308839e-02, -3.61014002e-04], [-1.91843410e-03, 6.48588368e-02, -5.87826187e-02, 3.60838917e-03], [ 4.35995936e-03, 6.47872116e-02, -5.65168053e-02, 4.71093336e-03], ..., [ 7.94672426e-03, 6.75807164e-02, -5.63313282e-02, -1.47784829e-04], [-1.31529224e-03, 5.92130264e-02, -5.81009382e-02, 3.96237167e-03], [ 5.55080638e-03, 6.23952161e-02, -5.76088140e-02, -3.76878678e-03]], [[ 4.13643501e-03, 5.47443780e-02, -5.97690662e-02, -3.06882849e-03], [ 3.19892639e-03, 6.29689960e-02, -6.04330480e-02, 4.04539302e-03], [ 7.62099127e-03, 6.33083802e-02, -5.69458811e-02, 3.34054624e-03], ... [ 5.11807677e-03, 6.28919145e-02, -5.29486257e-02, -6.17973039e-05], [ 1.68063824e-04, 6.49121813e-02, -6.20664321e-02, 2.88811104e-03], [-2.74165987e-03, 6.18243942e-02, -5.72046509e-02, 2.77271404e-03]], [[ 4.66838199e-03, 6.50293320e-02, -5.53405183e-02, 4.70843165e-03], [ 3.58246737e-03, 5.88003555e-02, -5.74237099e-02, -2.67996208e-03], [-1.17035656e-03, 6.63343357e-02, -5.81246770e-02, 2.34420384e-03], ..., [ 7.64189080e-03, 6.75806276e-02, -5.22560665e-02, 2.83681101e-03], [-3.01968156e-03, 6.03621191e-02, -6.62141618e-02, -1.15545314e-03], [ 2.54997426e-03, 6.67754216e-02, -5.51418500e-02, 2.30388176e-03]]])
- beta_channel(chain, draw, channel)float640.3975 0.2199 ... 0.3708 0.3032
array([[[0.39747966, 0.21988804], [0.34515166, 0.25144203], [0.38923905, 0.27966718], ..., [0.36613939, 0.22342244], [0.36084128, 0.21863902], [0.35797294, 0.29516133]], [[0.35420288, 0.22517493], [0.38252049, 0.24562958], [0.36322659, 0.26906047], ..., [0.354326 , 0.23809452], [0.34863784, 0.23125968], [0.32616385, 0.26794348]], [[0.35108301, 0.25902999], [0.36757113, 0.24140852], [0.37625117, 0.25125183], ..., [0.35758592, 0.23179333], [0.37958889, 0.26137228], [0.36196509, 0.2821071 ]], [[0.33416923, 0.23833189], [0.35337092, 0.26069343], [0.38017521, 0.42298183], ..., [0.36616889, 0.37683898], [0.37366401, 0.23341168], [0.37077652, 0.30324672]]])
- alpha(chain, draw, channel)float640.4327 0.1476 ... 0.4033 0.2364
array([[[0.43272042, 0.14763962], [0.37628828, 0.21490056], [0.44952034, 0.19114875], ..., [0.3918313 , 0.2143133 ], [0.39587832, 0.18641637], [0.39023918, 0.22904142]], [[0.40099726, 0.18609191], [0.41830397, 0.19922713], [0.40902319, 0.19465306], ..., [0.38225161, 0.18357016], [0.37474468, 0.22267201], [0.35744327, 0.20237434]], [[0.37937136, 0.1810354 ], [0.39701279, 0.22641711], [0.40693796, 0.20299626], ..., [0.43417953, 0.21038369], [0.38818336, 0.22937401], [0.40052059, 0.1968152 ]], [[0.34478513, 0.20297456], [0.39019533, 0.20159526], [0.45334093, 0.19681061], ..., [0.41071744, 0.25705175], [0.43922073, 0.17603632], [0.40334685, 0.23643458]]])
- lam(chain, draw, channel)float642.864 3.748 5.017 ... 4.928 2.357
array([[[2.86425215, 3.7483983 ], [5.01728387, 3.22465384], [3.38145664, 2.46475436], ..., [4.03547871, 4.2791672 ], [3.99487994, 4.09929228], [4.01818239, 2.10356613]], [[4.20189298, 3.23864771], [3.78380769, 3.06663053], [3.85040114, 2.72983397], ..., [3.82269294, 3.73977378], [3.80472013, 3.48590908], [5.33750927, 2.37939828]], [[4.16650807, 2.84458786], [3.97025846, 3.38015519], [3.69400167, 2.86456307], ..., [4.18250848, 3.68758412], [4.08004477, 2.7849874 ], [4.01796744, 2.42955159]], [[4.52726296, 3.73440388], [4.12171083, 2.8086678 ], [3.98776329, 1.37034525], ..., [4.3643265 , 1.68699974], [3.68499589, 3.46548145], [4.92799961, 2.35656969]]])
- sigma(chain, draw)float640.0297 0.02959 ... 0.03126 0.02854
array([[0.02970057, 0.02959437, 0.03038651, ..., 0.02992881, 0.02988514, 0.03156086], [0.02910947, 0.03040008, 0.03222584, ..., 0.02925145, 0.02916137, 0.02987333], [0.03256473, 0.03259282, 0.03395681, ..., 0.02995864, 0.02846489, 0.02906484], [0.03165629, 0.03038313, 0.02800252, ..., 0.0307474 , 0.03125682, 0.0285407 ]])
- channel_adstock(chain, draw, date, channel)float640.1816 0.0 ... 0.3552 0.008762
array([[[[0.1815531 , 0. ], [0.14260994, 0. ], [0.22834396, 0. ], ..., [0.1499905 , 0.10926844], [0.22447859, 0.01613235], [0.34698014, 0.0023816 ]], [[0.19944852, 0. ], [0.14541148, 0. ], [0.2377752 , 0. ], ..., [0.15421733, 0.1465582 ], [0.23343663, 0.03149544], [0.3624971 , 0.00676512]], [[0.17625371, 0. ], [0.14140831, 0. ], [0.22533573, 0. ], ..., ... ..., [0.15162208, 0.16601091], [0.22801753, 0.04267339], [0.35316586, 0.01095633]], [[0.17950072, 0. ], [0.1421646 , 0. ], [0.22719164, 0. ], ..., [0.14951595, 0.12595762], [0.22342381, 0.02217312], [0.34511994, 0.00390258]], [[0.19085283, 0. ], [0.14430884, 0. ], [0.23337575, 0. ], ..., [0.15217504, 0.15686844], [0.22919074, 0.03708912], [0.35519933, 0.00876234]]]])
- channel_adstock_saturated(chain, draw, date, channel)float640.2543 0.0 0.2014 ... 0.704 0.01032
array([[[[0.25430202, 0. ], [0.2014423 , 0. ], [0.31583818, 0. ], ..., [0.21156136, 0.20197513], [0.31084602, 0.03022603], [0.45969091, 0.00446356]], [[0.46238838, 0. ], [0.3494221 , 0. ], [0.53454925, 0. ], ..., [0.36866399, 0.23199765], [0.52673016, 0.05073734], [0.72083234, 0.01090716]], [[0.28947865, 0. ], [0.23462945, 0. ], [0.36355954, 0. ], ..., ... ..., [0.31929703, 0.13912204], [0.46020511, 0.03597946], [0.6473163 , 0.0092414 ]], [[0.3191763 , 0. ], [0.2561073 , 0. ], [0.39575047, 0. ], ..., [0.26871921, 0.21485129], [0.38987949, 0.03840137], [0.56208967, 0.00676206]], [[0.43841044, 0. ], [0.34131216, 0. ], [0.51904945, 0. ], ..., [0.35832189, 0.18275915], [0.51147546, 0.04367375], [0.70401208, 0.01032416]]]])
- channel_contributions(chain, draw, date, channel)float640.1011 0.0 ... 0.261 0.003131
array([[[[0.10107988, 0. ], [0.08006922, 0. ], [0.12553925, 0. ], ..., [0.08409134, 0.04441191], [0.12355497, 0.00664634], [0.18271778, 0.00098148]], [[0.15959412, 0. ], [0.12060362, 0. ], [0.18450056, 0. ], ..., [0.12724499, 0.05833396], [0.18180179, 0.0127575 ], [0.24879648, 0.00274252]], [[0.1126764 , 0. ], [0.09132694, 0. ], [0.14151157, 0. ], ..., ... ..., [0.11691664, 0.05242661], [0.1685128 , 0.01355846], [0.23702709, 0.00348252]], [[0.1192647 , 0. ], [0.09569808, 0. ], [0.14787771, 0. ], ..., [0.1004107 , 0.0501488 ], [0.14568393, 0.00896333], [0.21003268, 0.00157834]], [[0.1625523 , 0. ], [0.12655054, 0. ], [0.19245135, 0. ], ..., [0.13285734, 0.05542111], [0.18964309, 0.01324392], [0.26103115, 0.00313077]]]])
- control_contributions(chain, draw, date, control)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.1188
array([[[[0. , 0. , 0. ], [0. , 0. , 0.0005746 ], [0. , 0. , 0.00114919], ..., [0. , 0. , 0.10112895], [0. , 0. , 0.10170355], [0. , 0. , 0.10227814]], [[0. , 0. , 0. ], [0. , 0. , 0.00061704], [0. , 0. , 0.00123407], ..., [0. , 0. , 0.10859852], [0. , 0. , 0.10921556], [0. , 0. , 0.1098326 ]], [[0. , 0. , 0. ], [0. , 0. , 0.00063763], [0. , 0. , 0.00127526], ..., ... ..., [0. , 0. , 0.10826448], [0. , 0. , 0.10887962], [0. , 0. , 0.10949475]], [[0. , 0. , 0. ], [0. , 0. , 0.0006427 ], [0. , 0. , 0.00128541], ..., [0. , 0. , 0.11311578], [0. , 0. , 0.11375849], [0. , 0. , 0.11440119]], [[0. , 0. , 0. ], [0. , 0. , 0.00066721], [0. , 0. , 0.00133443], ..., [0. , 0. , 0.11742978], [0. , 0. , 0.118097 ], [0. , 0. , 0.11876421]]]])
- fourier_contributions(chain, draw, date, fourier_mode)float640.004958 -0.0007336 ... -0.001047
array([[[[ 4.95774954e-03, -7.33567038e-04, 1.35120980e-03, 3.60913017e-04], [ 4.91480489e-03, -8.17891640e-03, 1.49348357e-02, 3.48460341e-04], [ 4.80068044e-03, -1.55058127e-02, 2.76564051e-02, 3.15894122e-04], ..., [-3.48890034e-03, -4.40720905e-02, -5.71282093e-02, -3.49324817e-06], [-3.88681934e-03, -3.85097195e-02, -5.56113077e-02, 8.27099173e-05], [-4.22844658e-03, -3.23896226e-02, -5.08844556e-02, 1.64138968e-04]], [[-1.91829994e-03, -7.67045944e-04, 1.39027520e-03, -3.60737980e-03], [-1.90168339e-03, -8.55218996e-03, 1.53666229e-02, -3.48291344e-03], [-1.85752526e-03, -1.62134749e-02, 2.84559910e-02, -3.15740919e-03], ... [ 2.12488169e-03, -4.28884689e-02, -6.62110620e-02, -1.11804100e-05], [ 2.36723049e-03, -3.74754837e-02, -6.44529872e-02, 2.64719466e-04], [ 2.57529532e-03, -3.15197511e-02, -5.89746097e-02, 5.25339421e-04]], [[ 2.54979593e-03, -7.89712225e-04, 1.30416691e-03, -2.30323730e-03], [ 2.52770928e-03, -8.80490798e-03, 1.44148736e-02, -2.22376811e-03], [ 2.46901449e-03, -1.66925846e-02, 2.66935367e-02, -2.01594039e-03], ..., [-1.79435928e-03, -4.74452461e-02, -5.51392685e-02, 2.22928493e-05], [-1.99901106e-03, -4.14571467e-02, -5.36751784e-02, -5.27829582e-04], [-2.17471169e-03, -3.48686345e-02, -4.91128936e-02, -1.04748507e-03]]]])
- mu(chain, draw, date)float640.4922 0.4779 ... 0.5343 0.6067
array([[[0.49220889, 0.4778557 , 0.52914831, ..., 0.51013221, 0.51917242, 0.58383173], [0.48253517, 0.45049399, 0.52080572, ..., 0.51854251, 0.53480922, 0.60298718], [0.47454763, 0.45962161, 0.51570048, ..., 0.51005826, 0.52307717, 0.58981955], ..., [0.48926008, 0.46240409, 0.52518171, ..., 0.52222545, 0.52673797, 0.59369961], [0.4825707 , 0.45873296, 0.52191945, ..., 0.52394809, 0.53235925, 0.59977951], [0.4959653 , 0.4705083 , 0.53256606, ..., 0.51358231, 0.53398808, 0.6033219 ]], [[0.49313542, 0.47023699, 0.53427596, ..., 0.52100609, 0.53929052, 0.60919536], [0.47507318, 0.45462685, 0.51739271, ..., 0.50882137, 0.52278221, 0.5927206 ], [0.48679539, 0.4649309 , 0.52513421, ..., 0.50546247, 0.51903428, 0.58642755], ... [0.4758345 , 0.45570457, 0.51577561, ..., 0.52293027, 0.53077526, 0.59669912], [0.47775355, 0.45060018, 0.51827874, ..., 0.50838255, 0.5256123 , 0.59737514], [0.47386164, 0.45007095, 0.5128358 , ..., 0.51514693, 0.53365051, 0.60339939]], [[0.50039882, 0.46556837, 0.53151247, ..., 0.52511132, 0.53388285, 0.59841661], [0.49167767, 0.4664165 , 0.52927345, ..., 0.51517028, 0.53146507, 0.60022269], [0.46134556, 0.44465078, 0.50618606, ..., 0.50121153, 0.52522484, 0.59785904], ..., [0.48405405, 0.45770919, 0.52178695, ..., 0.50812578, 0.52762602, 0.59650458], [0.47080929, 0.45636958, 0.51677125, ..., 0.50924621, 0.52166599, 0.59117525], [0.47431334, 0.44413168, 0.51523983, ..., 0.51235169, 0.53432487, 0.60672243]]])
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:52.592010
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
- sampling_time :
- 71.22048568725586
- tuning_steps :
- 1000
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000, control: 3, fourier_mode: 4, channel: 2, date: 179) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 ... 995 996 997 998 999 * control (control) <U7 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) <U11 'sin_order_1' ... 'cos_ord... * channel (channel) <U2 'x1' 'x2' * date (date) <U10 '2018-04-02' ... '2021-08-30' Data variables: (12/13) intercept (chain, draw) float64 0.3852 0.3278 ... 0.311 gamma_control (chain, draw, control) float64 0.2353 ... 0.00... gamma_fourier (chain, draw, fourier_mode) float64 0.004958 .... beta_channel (chain, draw, channel) float64 0.3975 ... 0.3032 alpha (chain, draw, channel) float64 0.4327 ... 0.2364 lam (chain, draw, channel) float64 2.864 ... 2.357 ... ... channel_adstock (chain, draw, date, channel) float64 0.1816 ..... channel_adstock_saturated (chain, draw, date, channel) float64 0.2543 ..... channel_contributions (chain, draw, date, channel) float64 0.1011 ..... control_contributions (chain, draw, date, control) float64 0.0 ... 0... fourier_contributions (chain, draw, date, fourier_mode) float64 0.00... mu (chain, draw, date) float64 0.4922 ... 0.6067 Attributes: created_at: 2023-08-03T12:44:52.592010 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0 sampling_time: 71.22048568725586 tuning_steps: 1000
xarray.Dataset -
- chain: 4
- draw: 1000
- date: 179
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- likelihood(chain, draw, date)float640.4729 0.4781 ... 0.5132 0.5917
array([[[0.47287942, 0.47813078, 0.51465129, ..., 0.52427118, 0.48808257, 0.64502033], [0.45271729, 0.43090349, 0.52388816, ..., 0.50669698, 0.56831357, 0.6213914 ], [0.42171949, 0.43005445, 0.51474497, ..., 0.47139577, 0.52505306, 0.5843854 ], ..., [0.48602618, 0.46158674, 0.48214092, ..., 0.52001007, 0.5588568 , 0.64542187], [0.47392491, 0.48522603, 0.57290291, ..., 0.5108139 , 0.51097266, 0.57536919], [0.52295468, 0.47749806, 0.5184336 , ..., 0.56059989, 0.50194709, 0.5448219 ]], [[0.44534133, 0.49686391, 0.57126524, ..., 0.51129144, 0.51708924, 0.61947504], [0.38988604, 0.48435177, 0.54436286, ..., 0.49925045, 0.52653 , 0.64186543], [0.54005746, 0.48779086, 0.59584443, ..., 0.49281092, 0.51468331, 0.56969741], ... [0.45774182, 0.4412412 , 0.50929802, ..., 0.52677074, 0.53297208, 0.57374545], [0.42837812, 0.44675722, 0.59275659, ..., 0.50487571, 0.50630096, 0.55256169], [0.47478711, 0.43370699, 0.5517417 , ..., 0.48946988, 0.55556668, 0.60029659]], [[0.50040094, 0.48237177, 0.48872577, ..., 0.57913077, 0.57688092, 0.63184387], [0.46921747, 0.50867525, 0.53736652, ..., 0.53987552, 0.54848383, 0.62268496], [0.47374027, 0.43857999, 0.52922524, ..., 0.42368666, 0.54641232, 0.60036874], ..., [0.51725923, 0.46773254, 0.54444294, ..., 0.50322911, 0.51189872, 0.59232263], [0.50870318, 0.40132392, 0.51047115, ..., 0.50286732, 0.5106579 , 0.5727394 ], [0.44793272, 0.41722096, 0.51152289, ..., 0.54099287, 0.51319513, 0.59169714]]])
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:55.266426
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000, date: 179) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 6 7 ... 992 993 994 995 996 997 998 999 * date (date) <U10 '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (chain, draw, date) float64 0.4729 0.4781 ... 0.5132 0.5917 Attributes: created_at: 2023-08-03T12:44:55.266426 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- chain: 4
- draw: 1000
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- process_time_diff(chain, draw)float640.02484 0.03027 ... 0.02287 0.04276
array([[0.02484465, 0.03026824, 0.0275498 , ..., 0.02033344, 0.0246395 , 0.01992772], [0.03949065, 0.01371447, 0.02974776, ..., 0.01909351, 0.01244942, 0.02200291], [0.02418727, 0.02139096, 0.02423729, ..., 0.02853269, 0.02975177, 0.01429801], [0.04396229, 0.04085172, 0.03761578, ..., 0.03177199, 0.02286654, 0.04275852]])
- lp(chain, draw)float64351.2 354.5 353.6 ... 353.0 353.4
array([[351.15588188, 354.49917291, 353.62608315, ..., 352.73204341, 355.68605299, 354.62048964], [348.97647245, 358.33147522, 356.24118053, ..., 355.5669823 , 353.41596179, 349.40172304], [355.33330248, 354.11476965, 353.02275297, ..., 356.37076335, 355.23282234, 357.27840173], [355.46829925, 356.75307698, 352.46180604, ..., 354.23307794, 352.95911501, 353.3783564 ]])
- max_energy_error(chain, draw)float64-0.03674 0.03495 ... 0.2547 0.1954
array([[-0.03674255, 0.03494822, 0.3392237 , ..., 0.18039873, 0.26088542, 0.49621841], [ 0.13823553, -0.18089455, 0.03477711, ..., -0.14652744, -0.09281548, 0.35230766], [ 0.03755447, -0.0662405 , 0.03674591, ..., 0.1285427 , 0.01519062, 0.13618743], [ 0.30428744, -0.2446454 , -0.02112695, ..., -0.02632085, 0.25474352, 0.19543155]])
- step_size(chain, draw)float640.07388 0.07388 ... 0.06563 0.06563
array([[0.07388311, 0.07388311, 0.07388311, ..., 0.07388311, 0.07388311, 0.07388311], [0.0714658 , 0.0714658 , 0.0714658 , ..., 0.0714658 , 0.0714658 , 0.0714658 ], [0.08111642, 0.08111642, 0.08111642, ..., 0.08111642, 0.08111642, 0.08111642], [0.06562828, 0.06562828, 0.06562828, ..., 0.06562828, 0.06562828, 0.06562828]])
- reached_max_treedepth(chain, draw)boolFalse False False ... False False
array([[False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False]])
- perf_counter_diff(chain, draw)float640.02507 0.03056 ... 0.02366 0.04421
array([[0.02507298, 0.03056349, 0.02787485, ..., 0.02106091, 0.02582147, 0.02055384], [0.04002584, 0.01379189, 0.03068306, ..., 0.01909887, 0.0124605 , 0.02201315], [0.02420191, 0.02140715, 0.02440291, ..., 0.03021452, 0.03137594, 0.01493147], [0.05295574, 0.0466779 , 0.03982227, ..., 0.03293008, 0.02366477, 0.04420793]])
- step_size_bar(chain, draw)float640.07122 0.07122 ... 0.07193 0.07193
array([[0.07121798, 0.07121798, 0.07121798, ..., 0.07121798, 0.07121798, 0.07121798], [0.07661654, 0.07661654, 0.07661654, ..., 0.07661654, 0.07661654, 0.07661654], [0.06641153, 0.06641153, 0.06641153, ..., 0.06641153, 0.06641153, 0.06641153], [0.071931 , 0.071931 , 0.071931 , ..., 0.071931 , 0.071931 , 0.071931 ]])
- smallest_eigval(chain, draw)float64nan nan nan nan ... nan nan nan nan
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]])
- acceptance_rate(chain, draw)float640.9998 0.9916 ... 0.8754 0.9391
array([[0.99981323, 0.99156617, 0.86087927, ..., 0.9423506 , 0.9186242 , 0.83478816], [0.9490059 , 0.99982621, 0.98453782, ..., 0.98754873, 0.97686967, 0.86907628], [0.98896688, 0.99065932, 0.99177597, ..., 0.95280949, 0.99488913, 0.91630383], [0.88759452, 0.990825 , 0.99666295, ..., 0.99622014, 0.87535508, 0.93914408]])
- perf_counter_start(chain, draw)float641.442e+04 1.442e+04 ... 1.444e+04
array([[14416.2873664 , 14416.31274647, 14416.34360081, ..., 14441.42891612, 14441.45019966, 14441.47626545], [14410.82677097, 14410.86706909, 14410.88139978, ..., 14436.66757347, 14436.68689378, 14436.69960516], [14409.35345573, 14409.37791282, 14409.39967388, ..., 14435.89103034, 14435.92153093, 14435.95342922], [14412.12049024, 14412.173873 , 14412.22084097, ..., 14437.55382856, 14437.58701967, 14437.61093389]])
- energy_error(chain, draw)float64-0.01344 0.02138 ... 0.09381 0.1034
array([[-0.01343999, 0.02138095, 0.19201605, ..., 0.10314587, -0.12546418, -0.01132211], [ 0.13080946, -0.18089455, 0.02378239, ..., -0.01192367, -0.03935264, 0.15098095], [ 0.01433681, -0.00304629, 0.00828812, ..., -0.0148316 , 0.01148575, 0.04128621], [ 0.11345492, -0.20100975, 0.00464202, ..., -0.0210118 , 0.09381406, 0.10337544]])
- index_in_trajectory(chain, draw)int64-31 33 32 -28 43 ... 44 -9 38 29
array([[-31, 33, 32, ..., -37, -41, -32], [-12, 18, -10, ..., 33, -11, -23], [-15, -59, -10, ..., -38, 35, 14], [ 48, -28, -34, ..., -9, 38, 29]])
- energy(chain, draw)float64-344.9 -345.2 ... -345.0 -346.9
array([[-344.93870218, -345.16252633, -346.5464796 , ..., -345.62667989, -345.34434866, -345.90163116], [-333.85438495, -345.87424529, -349.06552382, ..., -352.20633888, -349.62907148, -341.99915893], [-344.87714537, -350.74551005, -345.76644887, ..., -348.45764188, -347.33084329, -349.89438043], [-349.3319774 , -344.49423483, -346.52038173, ..., -348.01416215, -344.95524551, -346.92667977]])
- largest_eigval(chain, draw)float64nan nan nan nan ... nan nan nan nan
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]])
- n_steps(chain, draw)float6463.0 63.0 63.0 ... 63.0 63.0 127.0
array([[ 63., 63., 63., ..., 63., 63., 63.], [ 63., 31., 63., ..., 63., 31., 63.], [ 63., 63., 63., ..., 63., 63., 31.], [ 63., 63., 63., ..., 63., 63., 127.]])
- tree_depth(chain, draw)int646 6 6 6 6 6 6 6 ... 6 6 6 6 6 6 6 7
array([[6, 6, 6, ..., 6, 6, 6], [6, 5, 6, ..., 6, 5, 6], [6, 6, 6, ..., 6, 6, 5], [6, 6, 6, ..., 6, 6, 7]])
- diverging(chain, draw)boolFalse False False ... False False
array([[False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False]])
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- created_at :
- 2023-08-03T12:44:52.608151
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
- sampling_time :
- 71.22048568725586
- tuning_steps :
- 1000
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 ... 994 995 996 997 998 999 Data variables: (12/17) process_time_diff (chain, draw) float64 0.02484 0.03027 ... 0.04276 lp (chain, draw) float64 351.2 354.5 ... 353.0 353.4 max_energy_error (chain, draw) float64 -0.03674 0.03495 ... 0.1954 step_size (chain, draw) float64 0.07388 0.07388 ... 0.06563 reached_max_treedepth (chain, draw) bool False False False ... False False perf_counter_diff (chain, draw) float64 0.02507 0.03056 ... 0.04421 ... ... index_in_trajectory (chain, draw) int64 -31 33 32 -28 43 ... 44 -9 38 29 energy (chain, draw) float64 -344.9 -345.2 ... -345.0 -346.9 largest_eigval (chain, draw) float64 nan nan nan nan ... nan nan nan n_steps (chain, draw) float64 63.0 63.0 63.0 ... 63.0 127.0 tree_depth (chain, draw) int64 6 6 6 6 6 6 6 6 ... 6 6 6 6 6 6 7 diverging (chain, draw) bool False False False ... False False Attributes: created_at: 2023-08-03T12:44:52.608151 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0 sampling_time: 71.22048568725586 tuning_steps: 1000
xarray.Dataset -
- chain: 1
- draw: 500
- channel: 2
- control: 3
- fourier_mode: 4
- date: 179
- chain(chain)int640
array([0])
- draw(draw)int640 1 2 3 4 5 ... 495 496 497 498 499
array([ 0, 1, 2, ..., 497, 498, 499])
- channel(channel)<U2'x1' 'x2'
array(['x1', 'x2'], dtype='<U2')
- control(control)<U7'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype='<U7')
- fourier_mode(fourier_mode)<U11'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='<U11')
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- beta_channel(chain, draw, channel)float641.418 1.277 0.1118 ... 4.541 1.259
array([[[1.41771409e+00, 1.27651456e+00], [1.11759230e-01, 1.00391763e+00], [1.66078493e+00, 3.20596683e+00], [3.20419089e+00, 9.87694132e-01], [3.55738705e+00, 1.17779302e+00], [1.57480200e+00, 2.96252368e-01], [3.26279685e+00, 1.56043278e+00], [1.27876003e+00, 1.10874401e-01], [3.54175229e-01, 7.43369192e-04], [2.38913960e+00, 1.67625422e+00], [1.75669724e+00, 5.02332389e-02], [2.38221787e-01, 2.21589489e+00], [1.15402728e+00, 1.28912514e+00], [9.01387641e-01, 2.27254179e+00], [1.97424890e+00, 7.10213090e-01], [3.01153104e+00, 1.13393205e-01], [1.80193915e+00, 2.84006410e-01], [1.94642714e+00, 9.86562999e-01], [2.61711365e-01, 2.05874501e+00], [6.98596308e-01, 2.07954266e-01], ... [1.95652787e+00, 3.39117422e-01], [2.91587674e+00, 3.44216753e-02], [3.36193824e+00, 1.02603402e-01], [8.08479560e-02, 1.91336022e+00], [2.23524656e+00, 1.10874283e+00], [1.07506579e+00, 7.46518481e-03], [2.78408481e+00, 5.37883336e-02], [2.21400711e+00, 4.95268830e-01], [6.58985171e-01, 2.47089791e+00], [3.60840473e-01, 1.27136115e-01], [1.39541597e+00, 7.09987659e-01], [2.12174075e-01, 1.75399689e+00], [4.06291582e+00, 9.94070662e-01], [6.66311684e-01, 9.11884110e-01], [1.71819776e+00, 6.33663220e-01], [4.54245357e+00, 1.15071969e+00], [1.07630282e+00, 2.20479058e+00], [1.81437141e+00, 1.11845209e+00], [4.54819610e+00, 7.58313974e-01], [4.54092801e+00, 1.25927449e+00]]])
- gamma_control(chain, draw, control)float643.665 4.476 ... -0.5954 -1.201
array([[[ 3.66482459, 4.47564534, 3.04612384], [ 1.8484942 , 2.98944803, 0.45814775], [-1.28487187, 0.87898241, 0.0175121 ], ..., [-0.08253424, 1.42715996, -2.11053585], [-0.33669504, 1.06259901, 2.12543441], [ 0.84511814, -0.59539134, -1.20094649]]])
- gamma_fourier(chain, draw, fourier_mode)float640.08728 -0.1613 ... -0.1337 2.467
array([[[ 0.08727895, -0.16125849, -0.71021385, -0.22046244], [-0.98480157, -0.09054815, -0.17627153, -2.0710989 ], [ 0.90703613, -0.41613027, 4.51566395, -0.68117658], ..., [ 3.34541511, -2.06247692, 0.15811203, 0.74631286], [ 2.17838564, -0.08109065, 0.59851762, -0.07118254], [-0.32304809, -0.14205784, -0.13366498, 2.46669554]]])
- channel_contributions(chain, draw, date, channel)float640.2905 0.0 0.119 ... 3.186 0.1076
array([[[[2.90516504e-01, 0.00000000e+00], [1.19033514e-01, 0.00000000e+00], [2.73228572e-01, 0.00000000e+00], ..., [1.66297245e-01, 6.33972700e-03], [2.64799160e-01, 4.44643693e-05], [4.08043786e-01, 3.11853158e-07]], [[5.97122984e-02, 0.00000000e+00], [3.73626697e-02, 0.00000000e+00], [6.21567495e-02, 0.00000000e+00], ..., [4.23183280e-02, 2.28024037e-01], [6.13012219e-02, 5.21924459e-02], [8.33391527e-02, 1.17514998e-02]], [[6.69743994e-01, 0.00000000e+00], [2.91719758e-01, 0.00000000e+00], [6.35954640e-01, 0.00000000e+00], ..., ... ..., [2.32507655e-01, 1.30642798e-02], [3.74909454e-01, 1.20254676e-04], [5.75214477e-01, 1.10687536e-06]], [[1.19848505e+00, 0.00000000e+00], [1.03161115e+00, 0.00000000e+00], [1.58491311e+00, 0.00000000e+00], ..., [1.09437874e+00, 4.19086610e-02], [1.57037339e+00, 2.10121606e-03], [2.28147106e+00, 1.05243910e-04]], [[2.26777159e+00, 0.00000000e+00], [1.35144884e+00, 0.00000000e+00], [2.32981154e+00, 0.00000000e+00], ..., [1.56231581e+00, 2.35940757e-01], [2.29549604e+00, 1.78506809e-01], [3.18647033e+00, 1.07580873e-01]]]])
- intercept(chain, draw)float64-3.807 -1.606 ... 0.5065 -0.598
array([[-3.80664915e+00, -1.60632722e+00, -2.23287081e+00, 1.09787805e+00, 1.76016341e+00, -1.88967081e+00, 6.07935349e-01, -3.79703233e+00, -1.15201037e+00, -1.18584205e+00, -8.74344532e-01, 2.56337702e+00, 3.46090683e+00, -3.77754708e-01, 2.04130032e-01, 1.17706005e+00, 1.74583787e+00, 7.49616077e-01, -4.60983997e-01, -1.81504417e+00, 5.85722649e+00, 8.86564381e-01, -1.20215513e+00, 2.94730415e-01, 6.09652104e-01, 9.11875687e-01, -4.38947169e+00, 6.89550084e-01, 2.06504837e+00, 2.74654396e+00, 2.38194873e+00, -8.94219085e-01, 3.91248163e+00, -3.29180346e+00, -1.34649410e+00, 8.96551388e-02, -2.90473580e+00, 9.72659269e-02, -1.92257214e+00, -1.11137240e+00, -2.81659882e+00, -5.70027859e+00, 3.62964271e-01, -2.80605209e-01, -2.22468885e+00, 1.98732785e+00, 2.84508165e+00, 2.96739935e+00, -3.48394576e+00, -2.02887307e-01, -1.34791202e+00, -1.83266609e+00, -1.85205186e+00, 1.95568463e-01, -1.17267467e+00, -1.03010248e+00, 7.57360596e-01, -2.01141799e-01, 5.62960478e-01, 2.60324891e+00, ... 1.12283454e+00, -3.26569052e+00, 1.53617785e+00, -2.39620849e+00, 1.59846579e+00, -5.76747003e-01, -1.46739063e+00, -5.24181191e-02, 1.40837723e+00, -6.04833598e-01, 1.59690714e+00, 1.64638048e+00, -1.00528212e+00, 2.28411724e-01, -4.10244097e-01, 4.84134507e-01, 1.93437760e+00, 7.88297884e-01, -1.61553946e+00, 3.04686958e-01, -2.70082064e+00, -6.14513806e+00, -7.08004311e-01, -3.02819776e+00, 1.23298734e+00, -4.19611758e+00, 4.87400474e-01, 6.07163435e+00, 1.68453167e+00, 3.98394979e+00, 2.15590303e+00, 7.25736371e-01, -4.07869462e+00, 3.34821766e+00, 2.54238707e+00, -2.22477173e+00, -3.74008812e-01, 8.12533823e-01, 1.49192715e+00, 5.33468705e+00, 1.00686622e+00, 4.50202281e+00, -6.06565848e-01, -2.36020627e-02, 1.97849581e+00, 1.93642563e+00, -3.92982412e-01, 1.78024985e+00, -2.67678489e+00, 9.31340283e-01, 1.95256632e+00, -1.49401961e+00, 3.05449438e-01, 1.20708956e+00, -1.03227911e+00, -1.60946736e+00, -5.37938207e-01, 5.06498336e-01, -5.98010433e-01]])
- lam(chain, draw, channel)float641.372 1.643 4.847 ... 4.326 1.678
array([[[ 1.3720548 , 1.64270887], [ 4.846812 , 3.05089387], [ 2.85303097, 5.08265535], [ 3.90491712, 3.31338982], [ 4.45846384, 5.23646078], [ 1.69843734, 0.76837954], [ 8.02190759, 1.38004493], [ 3.27879988, 6.93637645], [ 3.28594503, 3.58567522], [ 1.98321149, 1.22753861], [ 9.93484412, 1.20314043], [ 1.62539436, 4.38944859], [ 5.96540237, 3.92421575], [ 8.17722329, 3.87321514], [ 5.12222528, 0.70012007], [ 3.37302323, 3.22603354], [ 2.19312691, 2.65267639], [ 2.86273852, 1.68693716], [ 1.64905395, 1.4875959 ], [ 4.58132884, 0.89593771], ... [ 1.51059352, 5.06906401], [ 3.8870985 , 0.71907266], [ 1.14851796, 2.68981586], [ 1.93168394, 8.08156595], [ 3.2294571 , 2.62666351], [ 4.51877697, 10.45226595], [ 2.69223401, 1.05351076], [ 1.32245143, 4.03977294], [ 1.46040357, 5.91052485], [ 1.99781008, 2.19104807], [ 3.46922155, 1.32888381], [ 5.46855487, 2.52903811], [ 1.56943531, 3.5625068 ], [ 0.58488156, 5.23058655], [ 0.67068795, 1.35500863], [ 3.02724692, 0.96446992], [ 4.70293848, 2.71689166], [ 1.49472697, 2.9505339 ], [ 3.38065588, 2.67843913], [ 4.32638843, 1.67784475]]])
- fourier_contributions(chain, draw, date, fourier_mode)float640.08727 0.001907 ... -0.1191 -1.122
array([[[[ 8.72728473e-02, 1.90710586e-03, 1.67973582e-02, 2.20400771e-01], [ 8.65168790e-02, 2.12633047e-02, 1.85660127e-01, 2.12796226e-01], [ 8.45079099e-02, 4.03115527e-02, 3.43806375e-01, 1.92908831e-01], ..., [-6.14162260e-02, 1.14577316e-01, -7.10180605e-01, -2.13324140e-03], [-6.84209213e-02, 1.00116429e-01, -6.91323474e-01, 5.05089279e-02], [-7.44346945e-02, 8.42055818e-02, -6.32562335e-01, 1.00235662e-01]], [[-9.84732695e-01, 1.07085783e-03, 4.16902030e-03, 2.07051955e+00], [-9.76202818e-01, 1.19395450e-02, 4.60799150e-02, 1.99907988e+00], [-9.53534856e-01, 2.26353150e-02, 8.53310226e-02, 1.81225095e+00], ... [-1.53288076e+00, 5.76164940e-02, 5.98489602e-01, -6.88777354e-04], [-1.70771017e+00, 5.03446739e-02, 5.82598156e-01, 1.63082367e-02], [-1.85780727e+00, 4.23437254e-02, 5.33078456e-01, 3.23639201e-02]], [[-3.23025497e-01, 1.68003148e-03, 3.16132753e-03, -2.46600554e+00], [-3.20227410e-01, 1.87315356e-02, 3.49419512e-02, -2.38092031e+00], [-3.12791555e-01, 3.55117558e-02, 6.47056842e-02, -2.15840554e+00], ..., [ 2.27321642e-01, 1.00934878e-01, -1.33658726e-01, 2.38682700e-02], [ 2.53248322e-01, 8.81958130e-02, -1.30109741e-01, -5.65130945e-01], [ 2.75507273e-01, 7.41794314e-02, -1.19050668e-01, -1.12151013e+00]]]])
- mu(chain, draw, date)float64-3.19 -0.1353 ... -211.0 -212.0
array([[[-3.18975456e+00, -1.35255259e-01, 3.22036177e+00, ..., 5.31824631e+02, 5.35012995e+02, 5.38288883e+02], [-4.55588189e-01, -2.99202865e-02, 3.38807451e-01, ..., 7.98590339e+01, 8.07304683e+01, 8.17108687e+01], [-7.70473012e-02, -1.49262202e+00, -2.16956752e+00, ..., 6.02611008e+00, 5.64451201e+00, 5.57311356e+00], ..., [ 2.50462802e+00, 3.32252116e-01, -1.34367874e+00, ..., -3.72470012e+02, -3.75086938e+02, -3.77612712e+02], [ 3.94118271e+00, 5.74584772e+00, 8.24432548e+00, ..., 3.74841779e+02, 3.77222405e+02, 3.79865379e+02], [-1.11442852e+00, -3.09498232e+00, -3.04107153e+00, ..., -2.09947870e+02, -2.11045333e+02, -2.11963308e+02]]])
- alpha(chain, draw, channel)float640.0521 0.007014 ... 0.2067 0.7527
array([[[5.21036437e-02, 7.01355180e-03], [2.30438776e-01, 2.25101248e-01], [6.23615608e-02, 9.70097144e-02], [1.32889237e-01, 5.00559550e-02], [2.86399158e-01, 4.86702102e-01], [1.12476609e-01, 1.38874353e-01], [4.38830006e-02, 2.27186361e-01], [2.17255274e-02, 1.14369354e-01], [3.13171807e-01, 3.25342124e-01], [8.69289147e-02, 3.13357452e-01], [4.80242501e-01, 5.57627343e-02], [2.99680856e-01, 3.66054083e-01], [4.38263867e-01, 4.03405202e-01], [3.77285663e-01, 3.77672564e-01], [6.12790820e-01, 1.64399192e-01], [6.03679618e-01, 2.86299055e-01], [4.38487606e-02, 2.33547181e-01], [5.70323120e-01, 4.12761879e-01], [3.35988660e-01, 8.92219801e-02], [2.01030313e-01, 3.47716797e-01], ... [3.96006546e-01, 2.27202810e-03], [2.61246602e-01, 3.87195477e-01], [1.65564394e-01, 1.71122640e-01], [1.46161941e-01, 5.36758595e-02], [3.77553319e-01, 5.30462189e-01], [5.67577800e-01, 5.01400248e-01], [2.31180070e-01, 2.93754129e-01], [1.48999380e-01, 2.05110752e-01], [2.66807613e-01, 1.60311428e-01], [6.70239481e-02, 2.47008160e-01], [2.38660236e-01, 4.32334615e-01], [3.91907264e-01, 5.43144348e-01], [3.86505130e-01, 5.99591575e-01], [1.16866568e-01, 2.04606808e-01], [4.63992803e-02, 3.68704752e-01], [6.13431324e-02, 5.71710076e-01], [2.74840902e-01, 3.33923134e-01], [6.20565186e-03, 9.20442678e-03], [5.02552224e-01, 5.00870321e-02], [2.06731998e-01, 7.52706120e-01]]])
- channel_adstock(chain, draw, date, channel)float640.303 0.0 0.1227 ... 0.4025 0.1021
array([[[[3.02993403e-01, 0.00000000e+00], [1.22676900e-01, 0.00000000e+00], [2.84486416e-01, 0.00000000e+00], ..., [1.71774527e-01, 6.04669065e-03], [2.75495702e-01, 4.24087781e-05], [4.31740283e-01, 2.97436162e-07]], [[2.45990848e-01, 0.00000000e+00], [1.43466340e-01, 0.00000000e+00], [2.58836419e-01, 0.00000000e+00], ..., [1.64433866e-01, 1.51539656e-01], [2.54290758e-01, 3.41117657e-02], [3.97458806e-01, 7.67393208e-03]], [[2.99714478e-01, 0.00000000e+00], [1.24423765e-01, 0.00000000e+00], [2.82844288e-01, 0.00000000e+00], ..., ... ..., [1.72414420e-01, 7.91803150e-03], [2.80521945e-01, 7.28809412e-05], [4.39336977e-01, 6.70827287e-07]], [[1.59657898e-01, 0.00000000e+00], [1.36560453e-01, 0.00000000e+00], [2.15166550e-01, 0.00000000e+00], ..., [1.45196794e-01, 4.13090949e-02], [2.13016631e-01, 2.06904996e-03], [3.26250262e-01, 1.03632537e-04]], [[2.53567568e-01, 0.00000000e+00], [1.41873951e-01, 0.00000000e+00], [2.62060189e-01, 0.00000000e+00], ..., [1.65811029e-01, 2.26006913e-01], [2.57343254e-01, 1.70116787e-01], [4.02499945e-01, 1.02082852e-01]]]])
- control_contributions(chain, draw, date, control)float640.0 0.0 0.0 0.0 ... 0.0 -0.0 -213.8
array([[[[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 3.04612384e+00], [ 0.00000000e+00, 0.00000000e+00, 6.09224768e+00], ..., [ 0.00000000e+00, 0.00000000e+00, 5.36117796e+02], [ 0.00000000e+00, 0.00000000e+00, 5.39163920e+02], [ 0.00000000e+00, 0.00000000e+00, 5.42210044e+02]], [[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 4.58147748e-01], [ 0.00000000e+00, 0.00000000e+00, 9.16295495e-01], ..., [ 0.00000000e+00, 0.00000000e+00, 8.06340036e+01], [ 0.00000000e+00, 0.00000000e+00, 8.10921513e+01], [ 0.00000000e+00, 0.00000000e+00, 8.15502991e+01]], [[-0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [-0.00000000e+00, 0.00000000e+00, 1.75121026e-02], [-0.00000000e+00, 0.00000000e+00, 3.50242052e-02], ..., ... ..., [-0.00000000e+00, 0.00000000e+00, -3.71454309e+02], [-0.00000000e+00, 0.00000000e+00, -3.73564845e+02], [-0.00000000e+00, 0.00000000e+00, -3.75675381e+02]], [[-0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [-0.00000000e+00, 0.00000000e+00, 2.12543441e+00], [-0.00000000e+00, 0.00000000e+00, 4.25086882e+00], ..., [-0.00000000e+00, 0.00000000e+00, 3.74076456e+02], [-0.00000000e+00, 0.00000000e+00, 3.76201891e+02], [-0.00000000e+00, 0.00000000e+00, 3.78327325e+02]], [[ 0.00000000e+00, -0.00000000e+00, -0.00000000e+00], [ 0.00000000e+00, -0.00000000e+00, -1.20094649e+00], [ 0.00000000e+00, -0.00000000e+00, -2.40189298e+00], ..., [ 0.00000000e+00, -0.00000000e+00, -2.11366582e+02], [ 0.00000000e+00, -0.00000000e+00, -2.12567529e+02], [ 0.00000000e+00, -0.00000000e+00, -2.13768475e+02]]]])
- channel_adstock_saturated(chain, draw, date, channel)float640.2049 0.0 ... 0.7017 0.08543
array([[[[2.04918965e-01, 0.00000000e+00], [8.39615789e-02, 0.00000000e+00], [1.92724734e-01, 0.00000000e+00], ..., [1.17299564e-01, 4.96643534e-03], [1.86778958e-01, 3.48326379e-05], [2.87818107e-01, 2.44300510e-07]], [[5.34294109e-01, 0.00000000e+00], [3.34313950e-01, 0.00000000e+00], [5.56166586e-01, 0.00000000e+00], ..., [3.78656223e-01, 2.27134210e-01], [5.48511490e-01, 5.19887733e-02], [7.45702637e-01, 1.17056415e-02]], [[4.03269552e-01, 0.00000000e+00], [1.75651737e-01, 0.00000000e+00], [3.82924140e-01, 0.00000000e+00], ..., ... ..., [1.28147773e-01, 1.16806789e-02], [2.06633247e-01, 1.07518843e-04], [3.17032376e-01, 9.89649326e-07]], [[2.63507779e-01, 0.00000000e+00], [2.26817650e-01, 0.00000000e+00], [3.48470707e-01, 0.00000000e+00], ..., [2.40618196e-01, 5.52655791e-02], [3.45273896e-01, 2.77090510e-03], [5.01621085e-01, 1.38786721e-04]], [[4.99407079e-01, 0.00000000e+00], [2.97615120e-01, 0.00000000e+00], [5.13069472e-01, 0.00000000e+00], ..., [3.44052098e-01, 1.87362453e-01], [5.05512537e-01, 1.41753693e-01], [7.01722274e-01, 8.54308364e-02]]]])
- sigma(chain, draw)float642.361 0.3904 3.818 ... 1.618 2.132
array([[2.36139233e+00, 3.90383666e-01, 3.81810433e+00, 1.59228477e+00, 2.43287675e+00, 5.48688025e-01, 1.56404487e+00, 1.31355316e+00, 1.28073124e+00, 4.40476811e-01, 1.78472661e+00, 3.15452436e-01, 1.27223844e+00, 2.75881580e+00, 1.84174204e+00, 1.04928691e+00, 8.87666065e-01, 2.76698391e+00, 3.93845643e-01, 1.63368287e+00, 7.61037943e-02, 4.12874596e+00, 5.21084915e-01, 7.39823091e-01, 4.41424800e-01, 4.29048289e-01, 3.34041839e+00, 1.25542616e+00, 4.57817916e-01, 8.80044727e-02, 1.06574419e+00, 1.76564681e+00, 2.27690976e+00, 1.89041265e-01, 8.88635101e-01, 1.33432244e+00, 7.80132690e-01, 1.95030689e+00, 8.10445679e-02, 2.24252401e+00, 1.91362347e+00, 3.29182182e+00, 1.05085213e+00, 2.59973995e+00, 1.63127745e+00, 6.20303474e-01, 2.49304481e+00, 8.57753406e-01, 3.20653855e+00, 6.30675444e-01, 6.20743332e-01, 1.11855341e+00, 2.62139702e+00, 5.34450725e-01, 1.64068248e+00, 3.31066988e-01, 1.57005887e+00, 2.17213393e-01, 9.41021038e-01, 1.81911249e+00, 3.38399559e+00, 1.51783639e+00, 2.61623802e+00, 4.87073540e+00, 1.63012547e+00, 4.09254852e+00, 7.52662003e+00, 7.35085773e-01, 3.59411170e-02, 2.80230892e+00, 8.94506226e-01, 1.24053879e+00, 1.82747678e+00, 2.09934339e+00, 7.88664727e-01, 4.26916806e-01, 1.14983676e+00, 1.23654745e+00, 1.17428296e-01, 7.81700139e-01, ... 2.13547357e-01, 1.01120588e+00, 1.30672122e+00, 4.49044393e+00, 1.91281676e+00, 4.96142187e+00, 4.98708791e-01, 1.07024576e-01, 4.17915453e-01, 3.81704808e+00, 1.16261074e+00, 7.22723755e-01, 1.86201283e+00, 1.86799111e-02, 1.24527650e+00, 5.46664954e-01, 3.26748238e-01, 1.92515845e+00, 1.01162172e+00, 2.18129768e+00, 2.79981680e+00, 1.95991477e+00, 1.54909445e+00, 6.11864595e-01, 1.86990892e+00, 2.51808836e+00, 2.07057185e+00, 1.60235293e-01, 3.25929283e-03, 2.93356848e+00, 2.30708361e+00, 2.96932167e+00, 1.99290693e-01, 1.66111871e+00, 1.47008512e-01, 9.69815960e-01, 4.34889374e-01, 1.23820793e+00, 4.86462445e-02, 2.93094018e+00, 3.78169300e+00, 3.82458453e-01, 3.08544739e+00, 2.47101876e+00, 1.48976925e+00, 8.72392926e-01, 4.12272884e+00, 1.16544319e+00, 1.41737129e+00, 2.02377787e+00, 2.06537114e+00, 1.51758571e+00, 1.67072208e+00, 3.36114202e-01, 1.48194524e+00, 1.40764682e+00, 2.67283019e+00, 1.01490958e+00, 2.27160853e+00, 1.78060168e+00, 4.87138762e-01, 2.75654088e+00, 1.56972219e+00, 1.71478319e+00, 3.13686584e-01, 2.69815336e+00, 3.14442335e+00, 2.64495073e+00, 1.87525957e-01, 1.95837070e+00, 5.28574288e-01, 1.10559983e+00, 1.44656343e+00, 5.90947836e-01, 2.50629194e+00, 1.50253993e+00, 2.56187286e-01, 8.14324408e-01, 1.61842954e+00, 2.13182998e+00]])
- chainPandasIndex
PandasIndex(Index([0], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 490, 491, 492, 493, 494, 495, 496, 497, 498, 499], dtype='int64', name='draw', length=500))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:54.644498
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (chain: 1, draw: 500, channel: 2, control: 3, fourier_mode: 4, date: 179) Coordinates: * chain (chain) int64 0 * draw (draw) int64 0 1 2 3 4 5 ... 495 496 497 498 499 * channel (channel) <U2 'x1' 'x2' * control (control) <U7 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) <U11 'sin_order_1' ... 'cos_ord... * date (date) <U10 '2018-04-02' ... '2021-08-30' Data variables: (12/13) beta_channel (chain, draw, channel) float64 1.418 ... 1.259 gamma_control (chain, draw, control) float64 3.665 ... -1.201 gamma_fourier (chain, draw, fourier_mode) float64 0.08728 ..... channel_contributions (chain, draw, date, channel) float64 0.2905 ..... intercept (chain, draw) float64 -3.807 -1.606 ... -0.598 lam (chain, draw, channel) float64 1.372 ... 1.678 ... ... mu (chain, draw, date) float64 -3.19 ... -212.0 alpha (chain, draw, channel) float64 0.0521 ... 0.7527 channel_adstock (chain, draw, date, channel) float64 0.303 ...... control_contributions (chain, draw, date, control) float64 0.0 ... -... channel_adstock_saturated (chain, draw, date, channel) float64 0.2049 ..... sigma (chain, draw) float64 2.361 0.3904 ... 2.132 Attributes: created_at: 2023-08-03T12:44:54.644498 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- chain: 1
- draw: 500
- date: 179
- chain(chain)int640
array([0])
- draw(draw)int640 1 2 3 4 5 ... 495 496 497 498 499
array([ 0, 1, 2, ..., 497, 498, 499])
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- likelihood(chain, draw, date)float64-7.646 -1.949 ... -210.5 -215.1
array([[[-7.64565815e+00, -1.94922477e+00, 2.89341956e+00, ..., 5.34868436e+02, 5.33969792e+02, 5.34099399e+02], [-6.65431494e-01, -1.43483037e-02, 2.20785878e-01, ..., 8.01458595e+01, 8.06515086e+01, 8.17227111e+01], [-3.15327903e+00, -2.96584709e+00, 1.77266105e+00, ..., 5.26393093e+00, 3.33724083e+00, 7.52370386e+00], ..., [ 4.21129384e+00, 1.18911955e-01, -1.45744539e+00, ..., -3.74089980e+02, -3.75432384e+02, -3.77886954e+02], [ 6.74795441e+00, 3.22809699e+00, 7.94359098e+00, ..., 3.75099611e+02, 3.76135254e+02, 3.81491191e+02], [-2.15351790e+00, -3.42633927e+00, -1.33782504e-01, ..., -2.09302513e+02, -2.10516049e+02, -2.15109385e+02]]])
- chainPandasIndex
PandasIndex(Index([0], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 490, 491, 492, 493, 494, 495, 496, 497, 498, 499], dtype='int64', name='draw', length=500))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:54.650068
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (chain: 1, draw: 500, date: 179) Coordinates: * chain (chain) int64 0 * draw (draw) int64 0 1 2 3 4 5 6 7 ... 492 493 494 495 496 497 498 499 * date (date) <U10 '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (chain, draw, date) float64 -7.646 -1.949 ... -210.5 -215.1 Attributes: created_at: 2023-08-03T12:44:54.650068 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- date: 179
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- likelihood(date)float640.4794 0.4527 ... 0.5388 0.5625
array([0.47936319, 0.45268134, 0.53738551, 0.46487367, 0.5343368 , 0.44239849, 0.60963642, 0.73132832, 0.59600126, 0.70565315, 0.61313561, 0.60049297, 0.56404656, 0.78633605, 0.60523784, 0.5580442 , 0.50641716, 0.586594 , 0.46992062, 0.42705583, 0.48426812, 0.38777049, 0.49079078, 0.50002789, 0.44113299, 0.46359002, 0.42484345, 0.42573496, 0.5126282 , 0.55393305, 0.7801562 , 0.61244259, 0.7820166 , 0.7741081 , 0.87677312, 0.63147717, 0.51546283, 0.71272935, 0.93485537, 0.79408756, 0.72228448, 0.68098843, 0.70650313, 0.73308506, 0.4805125 , 0.57954561, 0.93776656, 0.77561424, 0.66655529, 0.59547518, 0.76751515, 0.57312122, 0.50621332, 0.5308715 , 0.52295261, 0.79720566, 0.62933004, 0.46812934, 0.81995741, 0.60723799, 0.63667661, 0.63023046, 0.60081393, 0.54905189, 0.54151156, 0.67785878, 0.580275 , 0.48759392, 0.54008438, 0.7454827 , 0.4601459 , 0.47552149, 0.71676648, 0.72228072, 0.54215422, 0.85509146, 0.684061 , 0.56992491, 0.84343218, 0.58108193, 0.57400488, 0.7216718 , 0.70124307, 0.70769822, 0.89872242, 0.8115083 , 0.72059583, 0.57222288, 1. , 0.8366832 , 0.99252741, 0.70215306, 0.69084327, 0.71206105, 0.64219547, 0.81993542, 0.6896944 , 0.59591584, 0.6458122 , 0.50792736, 0.55944542, 0.63420523, 0.70343723, 0.7740387 , 0.70204359, 0.65253863, 0.81871625, 0.81911895, 0.68380948, 0.71220965, 0.54236837, 0.58017503, 0.6352586 , 0.62739849, 0.92329765, 0.85928619, 0.62316379, 0.56392299, 0.55616857, 0.4257328 , 0.43070972, 0.52466263, 0.54561821, 0.5264453 , 0.41628475, 0.49557027, 0.53537871, 0.53003824, 0.78154262, 0.53440301, 0.63876159, 0.59727845, 0.57348504, 0.72266752, 0.88323619, 0.67509495, 0.68280629, 0.76882837, 0.66983526, 0.70381398, 0.73990877, 0.6594292 , 0.83865735, 0.73595323, 0.56852858, 0.62116609, 0.52960021, 0.67721404, 0.65454618, 0.57113219, 0.55954835, 0.68309668, 0.64092662, 0.61267177, 0.58478466, 0.63905178, 0.56741971, 0.58706316, 0.63111636, 0.84924442, 0.89647751, 0.84943539, 0.68420327, 0.82623974, 0.70912897, 0.66280698, 0.60631287, 0.61775646, 0.54565334, 0.52431821, 0.77688667, 0.53243718, 0.45656482, 0.38411009, 0.42749903, 0.66954245, 0.49776812, 0.53883804, 0.56252938])
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:52.612711
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (date: 179) Coordinates: * date (date) <U10 '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (date) float64 0.4794 0.4527 0.5374 ... 0.4978 0.5388 0.5625 Attributes: created_at: 2023-08-03T12:44:52.612711 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- date: 179
- channel: 2
- control: 3
- fourier_mode: 4
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- channel(channel)<U2'x1' 'x2'
array(['x1', 'x2'], dtype='<U2')
- control(control)<U7'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype='<U7')
- fourier_mode(fourier_mode)<U11'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='<U11')
- channel_data(date, channel)float640.3196 0.0 0.1128 ... 0.4403 0.0
array([[3.19648241e-01, 0.00000000e+00], [1.12765324e-01, 0.00000000e+00], [2.93380707e-01, 0.00000000e+00], [7.16379574e-02, 0.00000000e+00], [3.88041940e-01, 0.00000000e+00], [4.73291540e-02, 0.00000000e+00], [4.25671645e-01, 0.00000000e+00], [3.35039834e-01, 8.84759215e-01], [2.53918849e-01, 0.00000000e+00], [9.41199784e-01, 0.00000000e+00], [4.26630818e-01, 0.00000000e+00], [3.64360623e-01, 0.00000000e+00], [4.42975062e-01, 0.00000000e+00], [4.24530352e-01, 9.69641782e-01], [3.29493374e-01, 0.00000000e+00], [9.25031639e-01, 0.00000000e+00], [3.09048312e-01, 0.00000000e+00], [9.12534800e-01, 0.00000000e+00], [2.51539259e-01, 0.00000000e+00], [2.37266186e-01, 0.00000000e+00], ... [1.70332079e-01, 9.38381372e-01], [4.29038699e-01, 9.20516422e-01], [9.13487319e-01, 0.00000000e+00], [1.42944657e-01, 0.00000000e+00], [1.86838303e-01, 8.55514629e-01], [3.14421972e-01, 0.00000000e+00], [4.00678428e-01, 0.00000000e+00], [1.45069138e-01, 0.00000000e+00], [1.48471239e-01, 0.00000000e+00], [7.00777532e-02, 0.00000000e+00], [1.99103276e-01, 0.00000000e+00], [3.62700587e-01, 9.14000224e-01], [2.41143360e-01, 0.00000000e+00], [4.05094103e-02, 0.00000000e+00], [6.76832332e-02, 0.00000000e+00], [3.31349195e-02, 0.00000000e+00], [1.66170470e-01, 8.68233263e-01], [1.72458556e-01, 0.00000000e+00], [2.81197012e-01, 0.00000000e+00], [4.40328682e-01, 0.00000000e+00]])
- target(date)float640.4794 0.4527 ... 0.5388 0.5625
array([0.47936319, 0.45268134, 0.53738551, 0.46487367, 0.5343368 , 0.44239849, 0.60963642, 0.73132832, 0.59600126, 0.70565315, 0.61313561, 0.60049297, 0.56404656, 0.78633605, 0.60523784, 0.5580442 , 0.50641716, 0.586594 , 0.46992062, 0.42705583, 0.48426812, 0.38777049, 0.49079078, 0.50002789, 0.44113299, 0.46359002, 0.42484345, 0.42573496, 0.5126282 , 0.55393305, 0.7801562 , 0.61244259, 0.7820166 , 0.7741081 , 0.87677312, 0.63147717, 0.51546283, 0.71272935, 0.93485537, 0.79408756, 0.72228448, 0.68098843, 0.70650313, 0.73308506, 0.4805125 , 0.57954561, 0.93776656, 0.77561424, 0.66655529, 0.59547518, 0.76751515, 0.57312122, 0.50621332, 0.5308715 , 0.52295261, 0.79720566, 0.62933004, 0.46812934, 0.81995741, 0.60723799, 0.63667661, 0.63023046, 0.60081393, 0.54905189, 0.54151156, 0.67785878, 0.580275 , 0.48759392, 0.54008438, 0.7454827 , 0.4601459 , 0.47552149, 0.71676648, 0.72228072, 0.54215422, 0.85509146, 0.684061 , 0.56992491, 0.84343218, 0.58108193, 0.57400488, 0.7216718 , 0.70124307, 0.70769822, 0.89872242, 0.8115083 , 0.72059583, 0.57222288, 1. , 0.8366832 , 0.99252741, 0.70215306, 0.69084327, 0.71206105, 0.64219547, 0.81993542, 0.6896944 , 0.59591584, 0.6458122 , 0.50792736, 0.55944542, 0.63420523, 0.70343723, 0.7740387 , 0.70204359, 0.65253863, 0.81871625, 0.81911895, 0.68380948, 0.71220965, 0.54236837, 0.58017503, 0.6352586 , 0.62739849, 0.92329765, 0.85928619, 0.62316379, 0.56392299, 0.55616857, 0.4257328 , 0.43070972, 0.52466263, 0.54561821, 0.5264453 , 0.41628475, 0.49557027, 0.53537871, 0.53003824, 0.78154262, 0.53440301, 0.63876159, 0.59727845, 0.57348504, 0.72266752, 0.88323619, 0.67509495, 0.68280629, 0.76882837, 0.66983526, 0.70381398, 0.73990877, 0.6594292 , 0.83865735, 0.73595323, 0.56852858, 0.62116609, 0.52960021, 0.67721404, 0.65454618, 0.57113219, 0.55954835, 0.68309668, 0.64092662, 0.61267177, 0.58478466, 0.63905178, 0.56741971, 0.58706316, 0.63111636, 0.84924442, 0.89647751, 0.84943539, 0.68420327, 0.82623974, 0.70912897, 0.66280698, 0.60631287, 0.61775646, 0.54565334, 0.52431821, 0.77688667, 0.53243718, 0.45656482, 0.38411009, 0.42749903, 0.66954245, 0.49776812, 0.53883804, 0.56252938])
- control_data(date, control)float640.0 0.0 0.0 0.0 ... 0.0 0.0 178.0
array([[ 0., 0., 0.], [ 0., 0., 1.], [ 0., 0., 2.], [ 0., 0., 3.], [ 0., 0., 4.], [ 0., 0., 5.], [ 0., 0., 6.], [ 0., 0., 7.], [ 0., 0., 8.], [ 0., 0., 9.], [ 0., 0., 10.], [ 0., 0., 11.], [ 0., 0., 12.], [ 0., 0., 13.], [ 0., 0., 14.], [ 0., 0., 15.], [ 0., 0., 16.], [ 0., 0., 17.], [ 0., 0., 18.], [ 0., 0., 19.], ... [ 0., 0., 159.], [ 0., 0., 160.], [ 0., 0., 161.], [ 0., 0., 162.], [ 0., 0., 163.], [ 0., 0., 164.], [ 0., 0., 165.], [ 0., 0., 166.], [ 0., 0., 167.], [ 0., 0., 168.], [ 0., 0., 169.], [ 0., 0., 170.], [ 0., 0., 171.], [ 0., 0., 172.], [ 0., 0., 173.], [ 0., 0., 174.], [ 0., 0., 175.], [ 0., 0., 176.], [ 0., 0., 177.], [ 0., 0., 178.]])
- fourier_data(date, fourier_mode)float640.9999 -0.01183 ... 0.8907 -0.4547
array([[ 0.99993007, -0.01182639, -0.02365113, -0.99972027], [ 0.99126855, -0.13185852, -0.2614144 , -0.96522666], [ 0.96825075, -0.24998097, -0.48408852, -0.87501903], [ 0.93121004, -0.36448301, -0.67882048, -0.73430426], [ 0.88068286, -0.47370635, -0.83437012, -0.55120459], [ 0.81740098, -0.57606912, -0.94175893, -0.33628873], [ 0.74228091, -0.67008884, -0.99478831, -0.10196189], [ 0.65641058, -0.75440384, -0.99039732, 0.13825031], [ 0.56103363, -0.82779301, -0.92883943, 0.37048254], [ 0.45753138, -0.88919348, -0.81366783, 0.58133008], [ 0.34740282, -0.93771599, -0.65153036, 0.75862256], [ 0.23224292, -0.97265781, -0.45178579, 0.89212645], [ 0.11371951, -0.99351289, -0.22596361, 0.97413574], [-0.00645086, -0.99997919, 0.01290146, 0.99991677], [-0.12652782, -0.99196306, 0.25102184, 0.96798142], [-0.2447723 , -0.96958059, 0.47465294, 0.88017304], [-0.35947181, -0.93315594, 0.67088652, 0.74156003], [-0.46896519, -0.88321665, 0.82839573, 0.5601433 ], [-0.57166667, -0.82048596, 0.93808896, 0.34639443], [-0.66608886, -0.7458724 , 0.99363459, 0.11265127], ... [ 0.95406655, -0.29959476, -0.57166667, -0.82048596], [ 0.91116864, -0.41203364, -0.75086425, -0.66045656], [ 0.85507451, -0.51850514, -0.88672106, -0.46230483], [ 0.78659657, -0.61746728, -0.97139528, -0.23746832], [ 0.70672656, -0.7074868 , -0.99999942, 0.00107515], [ 0.61662121, -0.78725998, -0.97088241, 0.23955656], [ 0.51758551, -0.85563149, -0.88572492, 0.46421048], [ 0.41105375, -0.91161111, -0.74944234, 0.66206962], [ 0.29856882, -0.95438811, -0.56990106, 0.82171332], [ 0.18175979, -0.98334296, -0.35746442, 0.93392676], [ 0.06231838, -0.99805632, -0.1243945 , 0.99223284], [-0.05802557, -0.9983151 , 0.1158556 , 0.99326607], [-0.17752915, -0.98411554, 0.34941839, 0.9369668 ], [-0.29446162, -0.95566331, 0.56281233, 0.82658471], [-0.40712949, -0.91337045, 0.74372008, 0.66849116], [-0.513901 , -0.8578495 , 0.88169943, 0.47181152], [-0.61322983, -0.78990454, 0.96878605, 0.24789836], [-0.70367741, -0.7105196 , 0.99995318, 0.00967621], [-0.78393382, -0.6208444 , 0.97340184, -0.22910446], [-0.85283672, -0.52217768, 0.8906646 , -0.45466095]])
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- created_at :
- 2023-08-03T12:44:52.614490
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (date: 179, channel: 2, control: 3, fourier_mode: 4) Coordinates: * date (date) <U10 '2018-04-02' '2018-04-09' ... '2021-08-30' * channel (channel) <U2 'x1' 'x2' * control (control) <U7 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) <U11 'sin_order_1' ... 'cos_order_2' Data variables: channel_data (date, channel) float64 0.3196 0.0 0.1128 ... 0.0 0.4403 0.0 target (date) float64 0.4794 0.4527 0.5374 ... 0.4978 0.5388 0.5625 control_data (date, control) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 178.0 fourier_data (date, fourier_mode) float64 0.9999 -0.01183 ... -0.4547 Attributes: created_at: 2023-08-03T12:44:52.614490 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- index: 179
- index(index)int640 1 2 3 4 5 ... 174 175 176 177 178
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153, 154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164, 165, 166, 167, 168, 169, 170, 171, 172, 173, 174, 175, 176, 177, 178])
- date_week(index)object'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', ... '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype=object)
- x1(index)float640.3186 0.1124 ... 0.2803 0.4389
array([3.18580018e-01, 1.12388476e-01, 2.92400266e-01, 7.13985526e-02, 3.86745154e-01, 4.71709861e-02, 4.24249105e-01, 3.33920175e-01, 2.53070285e-01, 9.38054416e-01, 4.25205073e-01, 3.63142977e-01, 4.41494696e-01, 4.23111626e-01, 3.28392249e-01, 9.21940303e-01, 3.08015512e-01, 9.09485226e-01, 2.50698647e-01, 2.36473273e-01, 4.03137932e-01, 1.47177719e-01, 3.63041014e-01, 1.47066490e-01, 9.46704311e-02, 2.59245305e-01, 5.74838249e-02, 2.06465328e-02, 1.65636049e-01, 2.14007141e-01, 3.60517692e-01, 1.22368556e-01, 9.58683953e-01, 3.21972344e-01, 2.53234055e-01, 6.40119796e-02, 2.36553200e-02, 1.83164569e-01, 9.25302245e-01, 9.96658129e-01, 4.01689634e-01, 1.73745461e-01, 9.55372645e-01, 3.55889419e-01, 9.29181335e-04, 4.37815667e-01, 9.73976316e-01, 9.77903945e-01, 2.48141704e-01, 4.05620409e-01, 2.49040963e-01, 5.30524844e-02, 6.63217207e-02, 9.19314691e-02, 2.84652565e-01, 3.73845327e-01, 3.11033721e-01, 1.08200596e-02, 3.25854693e-01, 4.30160827e-01, 4.42189999e-01, 2.68919050e-01, 3.84406892e-01, 3.03780772e-01, 2.22092463e-01, 9.84087017e-01, 1.78161753e-01, 1.52862615e-01, 4.32812209e-01, 3.80735892e-01, 1.33810881e-01, 2.18715931e-01, 3.24034681e-01, 3.66676692e-01, 1.50298760e-01, 9.31123777e-01, 2.99199062e-01, 1.59348190e-01, 4.49282836e-01, 9.18307179e-03, ... 2.83962083e-01, 4.29271667e-01, 1.55574946e-01, 1.07068213e-01, 4.46958034e-01, 3.53012159e-01, 4.05501510e-01, 9.95101996e-01, 1.95552073e-01, 4.21105629e-01, 3.89127721e-02, 2.74762370e-01, 3.88551689e-01, 3.98177912e-01, 9.30166751e-01, 2.59848823e-01, 1.94820914e-01, 2.58231310e-01, 3.02189662e-01, 1.03497234e-01, 8.01700983e-02, 4.15976836e-01, 3.96173495e-01, 4.43288434e-01, 6.38882192e-02, 2.56961240e-01, 4.16716500e-01, 1.89344301e-01, 1.21168779e-02, 3.07204390e-01, 2.79346139e-01, 1.55859670e-01, 2.51259804e-01, 4.15636348e-01, 1.50413447e-01, 4.18457229e-02, 2.92710243e-01, 3.91623929e-01, 9.89705226e-02, 2.68473040e-01, 3.63484578e-01, 1.85363200e-01, 6.59774982e-02, 3.54568453e-01, 1.59422721e-01, 1.81976239e-01, 1.16747054e-01, 3.23780216e-01, 4.34122877e-01, 1.08988007e-01, 1.61353829e-01, 9.42322052e-01, 8.52032642e-02, 3.25819647e-01, 1.67913280e-01, 3.39621958e-01, 2.52901858e-01, 8.64855399e-02, 3.37226955e-01, 1.69762852e-01, 4.27604907e-01, 9.10434562e-01, 1.42466955e-01, 1.86213914e-01, 3.13371214e-01, 3.99339412e-01, 1.44584335e-01, 1.47975068e-01, 6.98435624e-02, 1.98437898e-01, 3.61488489e-01, 2.40337490e-01, 4.03740331e-02, 6.74570446e-02, 3.30241869e-02, 1.65615150e-01, 1.71882222e-01, 2.80257288e-01, 4.38857161e-01])
- x2(index)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0
array([0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.87978184, 0. , 0. , 0. , 0. , 0. , 0.96418688, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.80062317, 0. , 0. , 0. , 0.8535269 , 0. , 0. , 0.98859712, 0.87047511, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.9748662 , 0. , 0. , 0. , 0.90257287, 0. , 0. , 0. , 0. , 0.99437431, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.95798229, 0. , 0. , 0.88604706, 0.84193305, 0. , 0.91253659, 0. , 0. , 0.99123254, 0. , 0. , 0.95581799, 0.91704461, 0. , 0.80904352, 0. , 0. , 0. , 0.86960149, 0. , 0.9208528 , 0. , 0. , 0. , 0. , 0.99390622, 0. , 0. , 0. , 0. , 0. , 0. , 0.93665676, 0.9093652 , 0. , 0. , 0.80688306, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.89397651, 0.86774976, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.83998152, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.96018382, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.93310233, 0.91533788, 0. , 0. , 0.85070177, 0. , 0. , 0. , 0. , 0. , 0. , 0.90885834, 0. , 0. , 0. , 0. , 0.86334885, 0. , 0. , 0. ])
- event_1(index)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 1., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- event_2(index)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 1., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- dayofyear(index)int6492 99 106 113 ... 221 228 235 242
array([ 92, 99, 106, 113, 120, 127, 134, 141, 148, 155, 162, 169, 176, 183, 190, 197, 204, 211, 218, 225, 232, 239, 246, 253, 260, 267, 274, 281, 288, 295, 302, 309, 316, 323, 330, 337, 344, 351, 358, 365, 7, 14, 21, 28, 35, 42, 49, 56, 63, 70, 77, 84, 91, 98, 105, 112, 119, 126, 133, 140, 147, 154, 161, 168, 175, 182, 189, 196, 203, 210, 217, 224, 231, 238, 245, 252, 259, 266, 273, 280, 287, 294, 301, 308, 315, 322, 329, 336, 343, 350, 357, 364, 6, 13, 20, 27, 34, 41, 48, 55, 62, 69, 76, 83, 90, 97, 104, 111, 118, 125, 132, 139, 146, 153, 160, 167, 174, 181, 188, 195, 202, 209, 216, 223, 230, 237, 244, 251, 258, 265, 272, 279, 286, 293, 300, 307, 314, 321, 328, 335, 342, 349, 356, 363, 4, 11, 18, 25, 32, 39, 46, 53, 60, 67, 74, 81, 88, 95, 102, 109, 116, 123, 130, 137, 144, 151, 158, 165, 172, 179, 186, 193, 200, 207, 214, 221, 228, 235, 242])
- t(index)int640 1 2 3 4 5 ... 174 175 176 177 178
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153, 154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164, 165, 166, 167, 168, 169, 170, 171, 172, 173, 174, 175, 176, 177, 178])
- y(index)float643.985e+03 3.763e+03 ... 4.676e+03
array([3984.66223734, 3762.87179412, 4466.96738844, 3864.21937266, 4441.62527775, 3677.39655018, 5067.54633687, 6079.09904219, 4954.20536859, 5865.67657627, 5096.63305051, 4991.54228314, 4688.5848447 , 6536.34574052, 5030.98358508, 4638.69083472, 4209.54579837, 4876.00838907, 3906.171689 , 3549.86212243, 4025.43395537, 3223.30633321, 4079.65295872, 4156.43557336, 3666.8772267 , 3853.54920598, 3531.47191787, 3538.8824745 , 4261.17449177, 4604.51723377, 6484.97624603, 5090.8724376 , 6500.44070675, 6434.7020032 , 7288.09549867, 5249.09562308, 4284.73710406, 5924.4967947 , 7770.89886433, 6600.77946045, 6003.92292284, 5660.65332884, 5872.74193171, 6093.70178134, 3994.21575864, 4817.41932661, 7795.0978095 , 6447.22166693, 5540.6792271 , 4949.83235925, 6379.8986888 , 4764.01718599, 4207.85140173, 4412.82026656, 4346.99520328, 6626.69831268, 5231.24779003, 3891.28186583, 6815.82014683, 5047.60961099, 5292.31541818, 5238.73239384, 4994.21021861, 4563.9431011 , 4501.26480448, 5634.63844969, 4823.48226687, 4053.07938257, 4489.40147834, 6196.75603817, 3824.9202315 , 3952.72844853, 5958.05509279, 6003.89166699, 4506.60683165, 7107.8687139 , 5686.19380778, 4737.44808553, 7010.95204787, 4830.18985388, ... 4650.33832006, 5271.77236249, 5847.25691642, 6434.12509649, 5835.67243843, 5424.16703016, 6805.503155 , 6808.85054468, 5684.10310247, 5920.17689413, 4508.38691899, 4822.65128872, 5280.52839424, 5215.19196501, 7674.82634251, 7142.73704187, 5179.99140594, 4687.55775271, 4623.09983189, 3538.8645019 , 3580.23476566, 4361.20963926, 4535.40095043, 4376.02791122, 3460.32851473, 4119.38208881, 4450.28600415, 4405.89387854, 6496.50077682, 4442.17560168, 5309.6466714 , 4964.82187487, 4767.04140224, 6007.10694272, 7341.81915474, 5611.66436779, 5675.76416319, 6390.81472343, 5567.94366983, 5850.38866634, 6150.42324303, 5481.44428281, 6971.26166534, 6117.54314155, 4725.84123847, 5163.38572629, 4402.2528145 , 5629.27905386, 5440.85463743, 4747.48354262, 4651.19391143, 5678.17799695, 5327.64323813, 5092.77743153, 4860.96843213, 5312.05880983, 4716.62387739, 4879.90820541, 5246.0963942 , 7059.26575537, 7451.88641636, 7060.8531078 , 5687.37638691, 6868.04144218, 5894.56896391, 5509.52170002, 5039.91969411, 5135.04343508, 4535.6929473 , 4358.34664039, 6457.79858878, 4425.83485865, 3795.15282441, 3192.87959337, 3553.54614781, 5565.50968216, 4137.65148493, 4479.04135141, 4675.97343867])
- indexPandasIndex
PandasIndex(RangeIndex(start=0, stop=179, step=1, name='index'))
<xarray.Dataset> Dimensions: (index: 179) Coordinates: * index (index) int64 0 1 2 3 4 5 6 7 ... 171 172 173 174 175 176 177 178 Data variables: date_week (index) object '2018-04-02' '2018-04-09' ... '2021-08-30' x1 (index) float64 0.3186 0.1124 0.2924 ... 0.1719 0.2803 0.4389 x2 (index) float64 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.8633 0.0 0.0 0.0 event_1 (index) float64 0.0 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0 0.0 event_2 (index) float64 0.0 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0 0.0 dayofyear (index) int64 92 99 106 113 120 127 ... 207 214 221 228 235 242 t (index) int64 0 1 2 3 4 5 6 7 ... 171 172 173 174 175 176 177 178 y (index) float64 3.985e+03 3.763e+03 ... 4.479e+03 4.676e+03
xarray.Dataset
The fit()
method automatically builds the model using the priors from model_config
, and assigns the created model to our instance. You can access it as a normal attribute.
type(mmm.model)
pymc.model.Model
mmm.graphviz()
posterior trace can be accessed by fit_result
attribute
mmm.fit_result
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000, control: 3, fourier_mode: 4, channel: 2, date: 179) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 ... 995 996 997 998 999 * control (control) <U7 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) <U11 'sin_order_1' ... 'cos_ord... * channel (channel) <U2 'x1' 'x2' * date (date) <U10 '2018-04-02' ... '2021-08-30' Data variables: (12/13) intercept (chain, draw) float64 0.3852 0.3278 ... 0.311 gamma_control (chain, draw, control) float64 0.2353 ... 0.00... gamma_fourier (chain, draw, fourier_mode) float64 0.004958 .... beta_channel (chain, draw, channel) float64 0.3975 ... 0.3032 alpha (chain, draw, channel) float64 0.4327 ... 0.2364 lam (chain, draw, channel) float64 2.864 ... 2.357 ... ... channel_adstock (chain, draw, date, channel) float64 0.1816 ..... channel_adstock_saturated (chain, draw, date, channel) float64 0.2543 ..... channel_contributions (chain, draw, date, channel) float64 0.1011 ..... control_contributions (chain, draw, date, control) float64 0.0 ... 0... fourier_contributions (chain, draw, date, fourier_mode) float64 0.00... mu (chain, draw, date) float64 0.4922 ... 0.6067 Attributes: created_at: 2023-08-03T12:44:52.592010 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0 sampling_time: 71.22048568725586 tuning_steps: 1000
- chain: 4
- draw: 1000
- control: 3
- fourier_mode: 4
- channel: 2
- date: 179
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- control(control)<U7'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype='<U7')
- fourier_mode(fourier_mode)<U11'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='<U11')
- channel(channel)<U2'x1' 'x2'
array(['x1', 'x2'], dtype='<U2')
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- intercept(chain, draw)float640.3852 0.3278 ... 0.3526 0.311
array([[0.3851927 , 0.3278435 , 0.36165071, ..., 0.34388561, 0.35443253, 0.35253033], [0.34981775, 0.34584928, 0.35525882, ..., 0.35523169, 0.35908122, 0.34575935], [0.35563579, 0.3451598 , 0.35325558, ..., 0.34084824, 0.3358194 , 0.34582752], [0.35233725, 0.34990925, 0.33671441, ..., 0.33613902, 0.35255676, 0.31100003]])
- gamma_control(chain, draw, control)float640.2353 0.335 ... 0.3376 0.0006672
array([[[0.23532676, 0.33500538, 0.0005746 ], [0.25416368, 0.32078771, 0.00061704], [0.25503385, 0.34825216, 0.00063763], ..., [0.27940618, 0.36824443, 0.00063042], [0.20458129, 0.29476204, 0.0005926 ], [0.20935657, 0.30461187, 0.00064154]], [[0.24481066, 0.38185725, 0.00065845], [0.22396253, 0.31905371, 0.00063923], [0.2644513 , 0.29687398, 0.00059563], ..., [0.29669276, 0.32514522, 0.00065721], [0.28999838, 0.34466694, 0.00065541], [0.23563995, 0.32485093, 0.00053246]], [[0.25429729, 0.34677501, 0.00058459], [0.22986879, 0.33982102, 0.00061778], [0.22342951, 0.29270982, 0.00066354], ..., [0.25601694, 0.34311046, 0.00065063], [0.25895209, 0.29189666, 0.00062944], [0.22955645, 0.32483543, 0.00064999]], [[0.24109677, 0.31853461, 0.00058352], [0.29313553, 0.34149225, 0.00061645], [0.22163006, 0.31875355, 0.00068093], ..., [0.24132451, 0.354876 , 0.00061514], [0.25669935, 0.33493294, 0.0006427 ], [0.25828094, 0.33763074, 0.00066721]]])
- gamma_fourier(chain, draw, fourier_mode)float640.004958 0.06203 ... 0.002304
array([[[ 4.95809628e-03, 6.20279726e-02, -5.71308839e-02, -3.61014002e-04], [-1.91843410e-03, 6.48588368e-02, -5.87826187e-02, 3.60838917e-03], [ 4.35995936e-03, 6.47872116e-02, -5.65168053e-02, 4.71093336e-03], ..., [ 7.94672426e-03, 6.75807164e-02, -5.63313282e-02, -1.47784829e-04], [-1.31529224e-03, 5.92130264e-02, -5.81009382e-02, 3.96237167e-03], [ 5.55080638e-03, 6.23952161e-02, -5.76088140e-02, -3.76878678e-03]], [[ 4.13643501e-03, 5.47443780e-02, -5.97690662e-02, -3.06882849e-03], [ 3.19892639e-03, 6.29689960e-02, -6.04330480e-02, 4.04539302e-03], [ 7.62099127e-03, 6.33083802e-02, -5.69458811e-02, 3.34054624e-03], ... [ 5.11807677e-03, 6.28919145e-02, -5.29486257e-02, -6.17973039e-05], [ 1.68063824e-04, 6.49121813e-02, -6.20664321e-02, 2.88811104e-03], [-2.74165987e-03, 6.18243942e-02, -5.72046509e-02, 2.77271404e-03]], [[ 4.66838199e-03, 6.50293320e-02, -5.53405183e-02, 4.70843165e-03], [ 3.58246737e-03, 5.88003555e-02, -5.74237099e-02, -2.67996208e-03], [-1.17035656e-03, 6.63343357e-02, -5.81246770e-02, 2.34420384e-03], ..., [ 7.64189080e-03, 6.75806276e-02, -5.22560665e-02, 2.83681101e-03], [-3.01968156e-03, 6.03621191e-02, -6.62141618e-02, -1.15545314e-03], [ 2.54997426e-03, 6.67754216e-02, -5.51418500e-02, 2.30388176e-03]]])
- beta_channel(chain, draw, channel)float640.3975 0.2199 ... 0.3708 0.3032
array([[[0.39747966, 0.21988804], [0.34515166, 0.25144203], [0.38923905, 0.27966718], ..., [0.36613939, 0.22342244], [0.36084128, 0.21863902], [0.35797294, 0.29516133]], [[0.35420288, 0.22517493], [0.38252049, 0.24562958], [0.36322659, 0.26906047], ..., [0.354326 , 0.23809452], [0.34863784, 0.23125968], [0.32616385, 0.26794348]], [[0.35108301, 0.25902999], [0.36757113, 0.24140852], [0.37625117, 0.25125183], ..., [0.35758592, 0.23179333], [0.37958889, 0.26137228], [0.36196509, 0.2821071 ]], [[0.33416923, 0.23833189], [0.35337092, 0.26069343], [0.38017521, 0.42298183], ..., [0.36616889, 0.37683898], [0.37366401, 0.23341168], [0.37077652, 0.30324672]]])
- alpha(chain, draw, channel)float640.4327 0.1476 ... 0.4033 0.2364
array([[[0.43272042, 0.14763962], [0.37628828, 0.21490056], [0.44952034, 0.19114875], ..., [0.3918313 , 0.2143133 ], [0.39587832, 0.18641637], [0.39023918, 0.22904142]], [[0.40099726, 0.18609191], [0.41830397, 0.19922713], [0.40902319, 0.19465306], ..., [0.38225161, 0.18357016], [0.37474468, 0.22267201], [0.35744327, 0.20237434]], [[0.37937136, 0.1810354 ], [0.39701279, 0.22641711], [0.40693796, 0.20299626], ..., [0.43417953, 0.21038369], [0.38818336, 0.22937401], [0.40052059, 0.1968152 ]], [[0.34478513, 0.20297456], [0.39019533, 0.20159526], [0.45334093, 0.19681061], ..., [0.41071744, 0.25705175], [0.43922073, 0.17603632], [0.40334685, 0.23643458]]])
- lam(chain, draw, channel)float642.864 3.748 5.017 ... 4.928 2.357
array([[[2.86425215, 3.7483983 ], [5.01728387, 3.22465384], [3.38145664, 2.46475436], ..., [4.03547871, 4.2791672 ], [3.99487994, 4.09929228], [4.01818239, 2.10356613]], [[4.20189298, 3.23864771], [3.78380769, 3.06663053], [3.85040114, 2.72983397], ..., [3.82269294, 3.73977378], [3.80472013, 3.48590908], [5.33750927, 2.37939828]], [[4.16650807, 2.84458786], [3.97025846, 3.38015519], [3.69400167, 2.86456307], ..., [4.18250848, 3.68758412], [4.08004477, 2.7849874 ], [4.01796744, 2.42955159]], [[4.52726296, 3.73440388], [4.12171083, 2.8086678 ], [3.98776329, 1.37034525], ..., [4.3643265 , 1.68699974], [3.68499589, 3.46548145], [4.92799961, 2.35656969]]])
- sigma(chain, draw)float640.0297 0.02959 ... 0.03126 0.02854
array([[0.02970057, 0.02959437, 0.03038651, ..., 0.02992881, 0.02988514, 0.03156086], [0.02910947, 0.03040008, 0.03222584, ..., 0.02925145, 0.02916137, 0.02987333], [0.03256473, 0.03259282, 0.03395681, ..., 0.02995864, 0.02846489, 0.02906484], [0.03165629, 0.03038313, 0.02800252, ..., 0.0307474 , 0.03125682, 0.0285407 ]])
- channel_adstock(chain, draw, date, channel)float640.1816 0.0 ... 0.3552 0.008762
array([[[[0.1815531 , 0. ], [0.14260994, 0. ], [0.22834396, 0. ], ..., [0.1499905 , 0.10926844], [0.22447859, 0.01613235], [0.34698014, 0.0023816 ]], [[0.19944852, 0. ], [0.14541148, 0. ], [0.2377752 , 0. ], ..., [0.15421733, 0.1465582 ], [0.23343663, 0.03149544], [0.3624971 , 0.00676512]], [[0.17625371, 0. ], [0.14140831, 0. ], [0.22533573, 0. ], ..., ... ..., [0.15162208, 0.16601091], [0.22801753, 0.04267339], [0.35316586, 0.01095633]], [[0.17950072, 0. ], [0.1421646 , 0. ], [0.22719164, 0. ], ..., [0.14951595, 0.12595762], [0.22342381, 0.02217312], [0.34511994, 0.00390258]], [[0.19085283, 0. ], [0.14430884, 0. ], [0.23337575, 0. ], ..., [0.15217504, 0.15686844], [0.22919074, 0.03708912], [0.35519933, 0.00876234]]]])
- channel_adstock_saturated(chain, draw, date, channel)float640.2543 0.0 0.2014 ... 0.704 0.01032
array([[[[0.25430202, 0. ], [0.2014423 , 0. ], [0.31583818, 0. ], ..., [0.21156136, 0.20197513], [0.31084602, 0.03022603], [0.45969091, 0.00446356]], [[0.46238838, 0. ], [0.3494221 , 0. ], [0.53454925, 0. ], ..., [0.36866399, 0.23199765], [0.52673016, 0.05073734], [0.72083234, 0.01090716]], [[0.28947865, 0. ], [0.23462945, 0. ], [0.36355954, 0. ], ..., ... ..., [0.31929703, 0.13912204], [0.46020511, 0.03597946], [0.6473163 , 0.0092414 ]], [[0.3191763 , 0. ], [0.2561073 , 0. ], [0.39575047, 0. ], ..., [0.26871921, 0.21485129], [0.38987949, 0.03840137], [0.56208967, 0.00676206]], [[0.43841044, 0. ], [0.34131216, 0. ], [0.51904945, 0. ], ..., [0.35832189, 0.18275915], [0.51147546, 0.04367375], [0.70401208, 0.01032416]]]])
- channel_contributions(chain, draw, date, channel)float640.1011 0.0 ... 0.261 0.003131
array([[[[0.10107988, 0. ], [0.08006922, 0. ], [0.12553925, 0. ], ..., [0.08409134, 0.04441191], [0.12355497, 0.00664634], [0.18271778, 0.00098148]], [[0.15959412, 0. ], [0.12060362, 0. ], [0.18450056, 0. ], ..., [0.12724499, 0.05833396], [0.18180179, 0.0127575 ], [0.24879648, 0.00274252]], [[0.1126764 , 0. ], [0.09132694, 0. ], [0.14151157, 0. ], ..., ... ..., [0.11691664, 0.05242661], [0.1685128 , 0.01355846], [0.23702709, 0.00348252]], [[0.1192647 , 0. ], [0.09569808, 0. ], [0.14787771, 0. ], ..., [0.1004107 , 0.0501488 ], [0.14568393, 0.00896333], [0.21003268, 0.00157834]], [[0.1625523 , 0. ], [0.12655054, 0. ], [0.19245135, 0. ], ..., [0.13285734, 0.05542111], [0.18964309, 0.01324392], [0.26103115, 0.00313077]]]])
- control_contributions(chain, draw, date, control)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.1188
array([[[[0. , 0. , 0. ], [0. , 0. , 0.0005746 ], [0. , 0. , 0.00114919], ..., [0. , 0. , 0.10112895], [0. , 0. , 0.10170355], [0. , 0. , 0.10227814]], [[0. , 0. , 0. ], [0. , 0. , 0.00061704], [0. , 0. , 0.00123407], ..., [0. , 0. , 0.10859852], [0. , 0. , 0.10921556], [0. , 0. , 0.1098326 ]], [[0. , 0. , 0. ], [0. , 0. , 0.00063763], [0. , 0. , 0.00127526], ..., ... ..., [0. , 0. , 0.10826448], [0. , 0. , 0.10887962], [0. , 0. , 0.10949475]], [[0. , 0. , 0. ], [0. , 0. , 0.0006427 ], [0. , 0. , 0.00128541], ..., [0. , 0. , 0.11311578], [0. , 0. , 0.11375849], [0. , 0. , 0.11440119]], [[0. , 0. , 0. ], [0. , 0. , 0.00066721], [0. , 0. , 0.00133443], ..., [0. , 0. , 0.11742978], [0. , 0. , 0.118097 ], [0. , 0. , 0.11876421]]]])
- fourier_contributions(chain, draw, date, fourier_mode)float640.004958 -0.0007336 ... -0.001047
array([[[[ 4.95774954e-03, -7.33567038e-04, 1.35120980e-03, 3.60913017e-04], [ 4.91480489e-03, -8.17891640e-03, 1.49348357e-02, 3.48460341e-04], [ 4.80068044e-03, -1.55058127e-02, 2.76564051e-02, 3.15894122e-04], ..., [-3.48890034e-03, -4.40720905e-02, -5.71282093e-02, -3.49324817e-06], [-3.88681934e-03, -3.85097195e-02, -5.56113077e-02, 8.27099173e-05], [-4.22844658e-03, -3.23896226e-02, -5.08844556e-02, 1.64138968e-04]], [[-1.91829994e-03, -7.67045944e-04, 1.39027520e-03, -3.60737980e-03], [-1.90168339e-03, -8.55218996e-03, 1.53666229e-02, -3.48291344e-03], [-1.85752526e-03, -1.62134749e-02, 2.84559910e-02, -3.15740919e-03], ... [ 2.12488169e-03, -4.28884689e-02, -6.62110620e-02, -1.11804100e-05], [ 2.36723049e-03, -3.74754837e-02, -6.44529872e-02, 2.64719466e-04], [ 2.57529532e-03, -3.15197511e-02, -5.89746097e-02, 5.25339421e-04]], [[ 2.54979593e-03, -7.89712225e-04, 1.30416691e-03, -2.30323730e-03], [ 2.52770928e-03, -8.80490798e-03, 1.44148736e-02, -2.22376811e-03], [ 2.46901449e-03, -1.66925846e-02, 2.66935367e-02, -2.01594039e-03], ..., [-1.79435928e-03, -4.74452461e-02, -5.51392685e-02, 2.22928493e-05], [-1.99901106e-03, -4.14571467e-02, -5.36751784e-02, -5.27829582e-04], [-2.17471169e-03, -3.48686345e-02, -4.91128936e-02, -1.04748507e-03]]]])
- mu(chain, draw, date)float640.4922 0.4779 ... 0.5343 0.6067
array([[[0.49220889, 0.4778557 , 0.52914831, ..., 0.51013221, 0.51917242, 0.58383173], [0.48253517, 0.45049399, 0.52080572, ..., 0.51854251, 0.53480922, 0.60298718], [0.47454763, 0.45962161, 0.51570048, ..., 0.51005826, 0.52307717, 0.58981955], ..., [0.48926008, 0.46240409, 0.52518171, ..., 0.52222545, 0.52673797, 0.59369961], [0.4825707 , 0.45873296, 0.52191945, ..., 0.52394809, 0.53235925, 0.59977951], [0.4959653 , 0.4705083 , 0.53256606, ..., 0.51358231, 0.53398808, 0.6033219 ]], [[0.49313542, 0.47023699, 0.53427596, ..., 0.52100609, 0.53929052, 0.60919536], [0.47507318, 0.45462685, 0.51739271, ..., 0.50882137, 0.52278221, 0.5927206 ], [0.48679539, 0.4649309 , 0.52513421, ..., 0.50546247, 0.51903428, 0.58642755], ... [0.4758345 , 0.45570457, 0.51577561, ..., 0.52293027, 0.53077526, 0.59669912], [0.47775355, 0.45060018, 0.51827874, ..., 0.50838255, 0.5256123 , 0.59737514], [0.47386164, 0.45007095, 0.5128358 , ..., 0.51514693, 0.53365051, 0.60339939]], [[0.50039882, 0.46556837, 0.53151247, ..., 0.52511132, 0.53388285, 0.59841661], [0.49167767, 0.4664165 , 0.52927345, ..., 0.51517028, 0.53146507, 0.60022269], [0.46134556, 0.44465078, 0.50618606, ..., 0.50121153, 0.52522484, 0.59785904], ..., [0.48405405, 0.45770919, 0.52178695, ..., 0.50812578, 0.52762602, 0.59650458], [0.47080929, 0.45636958, 0.51677125, ..., 0.50924621, 0.52166599, 0.59117525], [0.47431334, 0.44413168, 0.51523983, ..., 0.51235169, 0.53432487, 0.60672243]]])
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:52.592010
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
- sampling_time :
- 71.22048568725586
- tuning_steps :
- 1000
If you wish to inspect the entire inference data, use the idata
attribute. Within idata
, you can find the entire dataset passed to the model under fit_data
.
mmm.idata
-
- chain: 4
- draw: 1000
- control: 3
- fourier_mode: 4
- channel: 2
- date: 179
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- control(control)<U7'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype='<U7')
- fourier_mode(fourier_mode)<U11'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='<U11')
- channel(channel)<U2'x1' 'x2'
array(['x1', 'x2'], dtype='<U2')
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- intercept(chain, draw)float640.3852 0.3278 ... 0.3526 0.311
array([[0.3851927 , 0.3278435 , 0.36165071, ..., 0.34388561, 0.35443253, 0.35253033], [0.34981775, 0.34584928, 0.35525882, ..., 0.35523169, 0.35908122, 0.34575935], [0.35563579, 0.3451598 , 0.35325558, ..., 0.34084824, 0.3358194 , 0.34582752], [0.35233725, 0.34990925, 0.33671441, ..., 0.33613902, 0.35255676, 0.31100003]])
- gamma_control(chain, draw, control)float640.2353 0.335 ... 0.3376 0.0006672
array([[[0.23532676, 0.33500538, 0.0005746 ], [0.25416368, 0.32078771, 0.00061704], [0.25503385, 0.34825216, 0.00063763], ..., [0.27940618, 0.36824443, 0.00063042], [0.20458129, 0.29476204, 0.0005926 ], [0.20935657, 0.30461187, 0.00064154]], [[0.24481066, 0.38185725, 0.00065845], [0.22396253, 0.31905371, 0.00063923], [0.2644513 , 0.29687398, 0.00059563], ..., [0.29669276, 0.32514522, 0.00065721], [0.28999838, 0.34466694, 0.00065541], [0.23563995, 0.32485093, 0.00053246]], [[0.25429729, 0.34677501, 0.00058459], [0.22986879, 0.33982102, 0.00061778], [0.22342951, 0.29270982, 0.00066354], ..., [0.25601694, 0.34311046, 0.00065063], [0.25895209, 0.29189666, 0.00062944], [0.22955645, 0.32483543, 0.00064999]], [[0.24109677, 0.31853461, 0.00058352], [0.29313553, 0.34149225, 0.00061645], [0.22163006, 0.31875355, 0.00068093], ..., [0.24132451, 0.354876 , 0.00061514], [0.25669935, 0.33493294, 0.0006427 ], [0.25828094, 0.33763074, 0.00066721]]])
- gamma_fourier(chain, draw, fourier_mode)float640.004958 0.06203 ... 0.002304
array([[[ 4.95809628e-03, 6.20279726e-02, -5.71308839e-02, -3.61014002e-04], [-1.91843410e-03, 6.48588368e-02, -5.87826187e-02, 3.60838917e-03], [ 4.35995936e-03, 6.47872116e-02, -5.65168053e-02, 4.71093336e-03], ..., [ 7.94672426e-03, 6.75807164e-02, -5.63313282e-02, -1.47784829e-04], [-1.31529224e-03, 5.92130264e-02, -5.81009382e-02, 3.96237167e-03], [ 5.55080638e-03, 6.23952161e-02, -5.76088140e-02, -3.76878678e-03]], [[ 4.13643501e-03, 5.47443780e-02, -5.97690662e-02, -3.06882849e-03], [ 3.19892639e-03, 6.29689960e-02, -6.04330480e-02, 4.04539302e-03], [ 7.62099127e-03, 6.33083802e-02, -5.69458811e-02, 3.34054624e-03], ... [ 5.11807677e-03, 6.28919145e-02, -5.29486257e-02, -6.17973039e-05], [ 1.68063824e-04, 6.49121813e-02, -6.20664321e-02, 2.88811104e-03], [-2.74165987e-03, 6.18243942e-02, -5.72046509e-02, 2.77271404e-03]], [[ 4.66838199e-03, 6.50293320e-02, -5.53405183e-02, 4.70843165e-03], [ 3.58246737e-03, 5.88003555e-02, -5.74237099e-02, -2.67996208e-03], [-1.17035656e-03, 6.63343357e-02, -5.81246770e-02, 2.34420384e-03], ..., [ 7.64189080e-03, 6.75806276e-02, -5.22560665e-02, 2.83681101e-03], [-3.01968156e-03, 6.03621191e-02, -6.62141618e-02, -1.15545314e-03], [ 2.54997426e-03, 6.67754216e-02, -5.51418500e-02, 2.30388176e-03]]])
- beta_channel(chain, draw, channel)float640.3975 0.2199 ... 0.3708 0.3032
array([[[0.39747966, 0.21988804], [0.34515166, 0.25144203], [0.38923905, 0.27966718], ..., [0.36613939, 0.22342244], [0.36084128, 0.21863902], [0.35797294, 0.29516133]], [[0.35420288, 0.22517493], [0.38252049, 0.24562958], [0.36322659, 0.26906047], ..., [0.354326 , 0.23809452], [0.34863784, 0.23125968], [0.32616385, 0.26794348]], [[0.35108301, 0.25902999], [0.36757113, 0.24140852], [0.37625117, 0.25125183], ..., [0.35758592, 0.23179333], [0.37958889, 0.26137228], [0.36196509, 0.2821071 ]], [[0.33416923, 0.23833189], [0.35337092, 0.26069343], [0.38017521, 0.42298183], ..., [0.36616889, 0.37683898], [0.37366401, 0.23341168], [0.37077652, 0.30324672]]])
- alpha(chain, draw, channel)float640.4327 0.1476 ... 0.4033 0.2364
array([[[0.43272042, 0.14763962], [0.37628828, 0.21490056], [0.44952034, 0.19114875], ..., [0.3918313 , 0.2143133 ], [0.39587832, 0.18641637], [0.39023918, 0.22904142]], [[0.40099726, 0.18609191], [0.41830397, 0.19922713], [0.40902319, 0.19465306], ..., [0.38225161, 0.18357016], [0.37474468, 0.22267201], [0.35744327, 0.20237434]], [[0.37937136, 0.1810354 ], [0.39701279, 0.22641711], [0.40693796, 0.20299626], ..., [0.43417953, 0.21038369], [0.38818336, 0.22937401], [0.40052059, 0.1968152 ]], [[0.34478513, 0.20297456], [0.39019533, 0.20159526], [0.45334093, 0.19681061], ..., [0.41071744, 0.25705175], [0.43922073, 0.17603632], [0.40334685, 0.23643458]]])
- lam(chain, draw, channel)float642.864 3.748 5.017 ... 4.928 2.357
array([[[2.86425215, 3.7483983 ], [5.01728387, 3.22465384], [3.38145664, 2.46475436], ..., [4.03547871, 4.2791672 ], [3.99487994, 4.09929228], [4.01818239, 2.10356613]], [[4.20189298, 3.23864771], [3.78380769, 3.06663053], [3.85040114, 2.72983397], ..., [3.82269294, 3.73977378], [3.80472013, 3.48590908], [5.33750927, 2.37939828]], [[4.16650807, 2.84458786], [3.97025846, 3.38015519], [3.69400167, 2.86456307], ..., [4.18250848, 3.68758412], [4.08004477, 2.7849874 ], [4.01796744, 2.42955159]], [[4.52726296, 3.73440388], [4.12171083, 2.8086678 ], [3.98776329, 1.37034525], ..., [4.3643265 , 1.68699974], [3.68499589, 3.46548145], [4.92799961, 2.35656969]]])
- sigma(chain, draw)float640.0297 0.02959 ... 0.03126 0.02854
array([[0.02970057, 0.02959437, 0.03038651, ..., 0.02992881, 0.02988514, 0.03156086], [0.02910947, 0.03040008, 0.03222584, ..., 0.02925145, 0.02916137, 0.02987333], [0.03256473, 0.03259282, 0.03395681, ..., 0.02995864, 0.02846489, 0.02906484], [0.03165629, 0.03038313, 0.02800252, ..., 0.0307474 , 0.03125682, 0.0285407 ]])
- channel_adstock(chain, draw, date, channel)float640.1816 0.0 ... 0.3552 0.008762
array([[[[0.1815531 , 0. ], [0.14260994, 0. ], [0.22834396, 0. ], ..., [0.1499905 , 0.10926844], [0.22447859, 0.01613235], [0.34698014, 0.0023816 ]], [[0.19944852, 0. ], [0.14541148, 0. ], [0.2377752 , 0. ], ..., [0.15421733, 0.1465582 ], [0.23343663, 0.03149544], [0.3624971 , 0.00676512]], [[0.17625371, 0. ], [0.14140831, 0. ], [0.22533573, 0. ], ..., ... ..., [0.15162208, 0.16601091], [0.22801753, 0.04267339], [0.35316586, 0.01095633]], [[0.17950072, 0. ], [0.1421646 , 0. ], [0.22719164, 0. ], ..., [0.14951595, 0.12595762], [0.22342381, 0.02217312], [0.34511994, 0.00390258]], [[0.19085283, 0. ], [0.14430884, 0. ], [0.23337575, 0. ], ..., [0.15217504, 0.15686844], [0.22919074, 0.03708912], [0.35519933, 0.00876234]]]])
- channel_adstock_saturated(chain, draw, date, channel)float640.2543 0.0 0.2014 ... 0.704 0.01032
array([[[[0.25430202, 0. ], [0.2014423 , 0. ], [0.31583818, 0. ], ..., [0.21156136, 0.20197513], [0.31084602, 0.03022603], [0.45969091, 0.00446356]], [[0.46238838, 0. ], [0.3494221 , 0. ], [0.53454925, 0. ], ..., [0.36866399, 0.23199765], [0.52673016, 0.05073734], [0.72083234, 0.01090716]], [[0.28947865, 0. ], [0.23462945, 0. ], [0.36355954, 0. ], ..., ... ..., [0.31929703, 0.13912204], [0.46020511, 0.03597946], [0.6473163 , 0.0092414 ]], [[0.3191763 , 0. ], [0.2561073 , 0. ], [0.39575047, 0. ], ..., [0.26871921, 0.21485129], [0.38987949, 0.03840137], [0.56208967, 0.00676206]], [[0.43841044, 0. ], [0.34131216, 0. ], [0.51904945, 0. ], ..., [0.35832189, 0.18275915], [0.51147546, 0.04367375], [0.70401208, 0.01032416]]]])
- channel_contributions(chain, draw, date, channel)float640.1011 0.0 ... 0.261 0.003131
array([[[[0.10107988, 0. ], [0.08006922, 0. ], [0.12553925, 0. ], ..., [0.08409134, 0.04441191], [0.12355497, 0.00664634], [0.18271778, 0.00098148]], [[0.15959412, 0. ], [0.12060362, 0. ], [0.18450056, 0. ], ..., [0.12724499, 0.05833396], [0.18180179, 0.0127575 ], [0.24879648, 0.00274252]], [[0.1126764 , 0. ], [0.09132694, 0. ], [0.14151157, 0. ], ..., ... ..., [0.11691664, 0.05242661], [0.1685128 , 0.01355846], [0.23702709, 0.00348252]], [[0.1192647 , 0. ], [0.09569808, 0. ], [0.14787771, 0. ], ..., [0.1004107 , 0.0501488 ], [0.14568393, 0.00896333], [0.21003268, 0.00157834]], [[0.1625523 , 0. ], [0.12655054, 0. ], [0.19245135, 0. ], ..., [0.13285734, 0.05542111], [0.18964309, 0.01324392], [0.26103115, 0.00313077]]]])
- control_contributions(chain, draw, date, control)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.1188
array([[[[0. , 0. , 0. ], [0. , 0. , 0.0005746 ], [0. , 0. , 0.00114919], ..., [0. , 0. , 0.10112895], [0. , 0. , 0.10170355], [0. , 0. , 0.10227814]], [[0. , 0. , 0. ], [0. , 0. , 0.00061704], [0. , 0. , 0.00123407], ..., [0. , 0. , 0.10859852], [0. , 0. , 0.10921556], [0. , 0. , 0.1098326 ]], [[0. , 0. , 0. ], [0. , 0. , 0.00063763], [0. , 0. , 0.00127526], ..., ... ..., [0. , 0. , 0.10826448], [0. , 0. , 0.10887962], [0. , 0. , 0.10949475]], [[0. , 0. , 0. ], [0. , 0. , 0.0006427 ], [0. , 0. , 0.00128541], ..., [0. , 0. , 0.11311578], [0. , 0. , 0.11375849], [0. , 0. , 0.11440119]], [[0. , 0. , 0. ], [0. , 0. , 0.00066721], [0. , 0. , 0.00133443], ..., [0. , 0. , 0.11742978], [0. , 0. , 0.118097 ], [0. , 0. , 0.11876421]]]])
- fourier_contributions(chain, draw, date, fourier_mode)float640.004958 -0.0007336 ... -0.001047
array([[[[ 4.95774954e-03, -7.33567038e-04, 1.35120980e-03, 3.60913017e-04], [ 4.91480489e-03, -8.17891640e-03, 1.49348357e-02, 3.48460341e-04], [ 4.80068044e-03, -1.55058127e-02, 2.76564051e-02, 3.15894122e-04], ..., [-3.48890034e-03, -4.40720905e-02, -5.71282093e-02, -3.49324817e-06], [-3.88681934e-03, -3.85097195e-02, -5.56113077e-02, 8.27099173e-05], [-4.22844658e-03, -3.23896226e-02, -5.08844556e-02, 1.64138968e-04]], [[-1.91829994e-03, -7.67045944e-04, 1.39027520e-03, -3.60737980e-03], [-1.90168339e-03, -8.55218996e-03, 1.53666229e-02, -3.48291344e-03], [-1.85752526e-03, -1.62134749e-02, 2.84559910e-02, -3.15740919e-03], ... [ 2.12488169e-03, -4.28884689e-02, -6.62110620e-02, -1.11804100e-05], [ 2.36723049e-03, -3.74754837e-02, -6.44529872e-02, 2.64719466e-04], [ 2.57529532e-03, -3.15197511e-02, -5.89746097e-02, 5.25339421e-04]], [[ 2.54979593e-03, -7.89712225e-04, 1.30416691e-03, -2.30323730e-03], [ 2.52770928e-03, -8.80490798e-03, 1.44148736e-02, -2.22376811e-03], [ 2.46901449e-03, -1.66925846e-02, 2.66935367e-02, -2.01594039e-03], ..., [-1.79435928e-03, -4.74452461e-02, -5.51392685e-02, 2.22928493e-05], [-1.99901106e-03, -4.14571467e-02, -5.36751784e-02, -5.27829582e-04], [-2.17471169e-03, -3.48686345e-02, -4.91128936e-02, -1.04748507e-03]]]])
- mu(chain, draw, date)float640.4922 0.4779 ... 0.5343 0.6067
array([[[0.49220889, 0.4778557 , 0.52914831, ..., 0.51013221, 0.51917242, 0.58383173], [0.48253517, 0.45049399, 0.52080572, ..., 0.51854251, 0.53480922, 0.60298718], [0.47454763, 0.45962161, 0.51570048, ..., 0.51005826, 0.52307717, 0.58981955], ..., [0.48926008, 0.46240409, 0.52518171, ..., 0.52222545, 0.52673797, 0.59369961], [0.4825707 , 0.45873296, 0.52191945, ..., 0.52394809, 0.53235925, 0.59977951], [0.4959653 , 0.4705083 , 0.53256606, ..., 0.51358231, 0.53398808, 0.6033219 ]], [[0.49313542, 0.47023699, 0.53427596, ..., 0.52100609, 0.53929052, 0.60919536], [0.47507318, 0.45462685, 0.51739271, ..., 0.50882137, 0.52278221, 0.5927206 ], [0.48679539, 0.4649309 , 0.52513421, ..., 0.50546247, 0.51903428, 0.58642755], ... [0.4758345 , 0.45570457, 0.51577561, ..., 0.52293027, 0.53077526, 0.59669912], [0.47775355, 0.45060018, 0.51827874, ..., 0.50838255, 0.5256123 , 0.59737514], [0.47386164, 0.45007095, 0.5128358 , ..., 0.51514693, 0.53365051, 0.60339939]], [[0.50039882, 0.46556837, 0.53151247, ..., 0.52511132, 0.53388285, 0.59841661], [0.49167767, 0.4664165 , 0.52927345, ..., 0.51517028, 0.53146507, 0.60022269], [0.46134556, 0.44465078, 0.50618606, ..., 0.50121153, 0.52522484, 0.59785904], ..., [0.48405405, 0.45770919, 0.52178695, ..., 0.50812578, 0.52762602, 0.59650458], [0.47080929, 0.45636958, 0.51677125, ..., 0.50924621, 0.52166599, 0.59117525], [0.47431334, 0.44413168, 0.51523983, ..., 0.51235169, 0.53432487, 0.60672243]]])
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:52.592010
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
- sampling_time :
- 71.22048568725586
- tuning_steps :
- 1000
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000, control: 3, fourier_mode: 4, channel: 2, date: 179) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 ... 995 996 997 998 999 * control (control) <U7 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) <U11 'sin_order_1' ... 'cos_ord... * channel (channel) <U2 'x1' 'x2' * date (date) <U10 '2018-04-02' ... '2021-08-30' Data variables: (12/13) intercept (chain, draw) float64 0.3852 0.3278 ... 0.311 gamma_control (chain, draw, control) float64 0.2353 ... 0.00... gamma_fourier (chain, draw, fourier_mode) float64 0.004958 .... beta_channel (chain, draw, channel) float64 0.3975 ... 0.3032 alpha (chain, draw, channel) float64 0.4327 ... 0.2364 lam (chain, draw, channel) float64 2.864 ... 2.357 ... ... channel_adstock (chain, draw, date, channel) float64 0.1816 ..... channel_adstock_saturated (chain, draw, date, channel) float64 0.2543 ..... channel_contributions (chain, draw, date, channel) float64 0.1011 ..... control_contributions (chain, draw, date, control) float64 0.0 ... 0... fourier_contributions (chain, draw, date, fourier_mode) float64 0.00... mu (chain, draw, date) float64 0.4922 ... 0.6067 Attributes: created_at: 2023-08-03T12:44:52.592010 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0 sampling_time: 71.22048568725586 tuning_steps: 1000
xarray.Dataset -
- chain: 4
- draw: 1000
- date: 179
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- likelihood(chain, draw, date)float640.4729 0.4781 ... 0.5132 0.5917
array([[[0.47287942, 0.47813078, 0.51465129, ..., 0.52427118, 0.48808257, 0.64502033], [0.45271729, 0.43090349, 0.52388816, ..., 0.50669698, 0.56831357, 0.6213914 ], [0.42171949, 0.43005445, 0.51474497, ..., 0.47139577, 0.52505306, 0.5843854 ], ..., [0.48602618, 0.46158674, 0.48214092, ..., 0.52001007, 0.5588568 , 0.64542187], [0.47392491, 0.48522603, 0.57290291, ..., 0.5108139 , 0.51097266, 0.57536919], [0.52295468, 0.47749806, 0.5184336 , ..., 0.56059989, 0.50194709, 0.5448219 ]], [[0.44534133, 0.49686391, 0.57126524, ..., 0.51129144, 0.51708924, 0.61947504], [0.38988604, 0.48435177, 0.54436286, ..., 0.49925045, 0.52653 , 0.64186543], [0.54005746, 0.48779086, 0.59584443, ..., 0.49281092, 0.51468331, 0.56969741], ... [0.45774182, 0.4412412 , 0.50929802, ..., 0.52677074, 0.53297208, 0.57374545], [0.42837812, 0.44675722, 0.59275659, ..., 0.50487571, 0.50630096, 0.55256169], [0.47478711, 0.43370699, 0.5517417 , ..., 0.48946988, 0.55556668, 0.60029659]], [[0.50040094, 0.48237177, 0.48872577, ..., 0.57913077, 0.57688092, 0.63184387], [0.46921747, 0.50867525, 0.53736652, ..., 0.53987552, 0.54848383, 0.62268496], [0.47374027, 0.43857999, 0.52922524, ..., 0.42368666, 0.54641232, 0.60036874], ..., [0.51725923, 0.46773254, 0.54444294, ..., 0.50322911, 0.51189872, 0.59232263], [0.50870318, 0.40132392, 0.51047115, ..., 0.50286732, 0.5106579 , 0.5727394 ], [0.44793272, 0.41722096, 0.51152289, ..., 0.54099287, 0.51319513, 0.59169714]]])
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:55.266426
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000, date: 179) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 6 7 ... 992 993 994 995 996 997 998 999 * date (date) <U10 '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (chain, draw, date) float64 0.4729 0.4781 ... 0.5132 0.5917 Attributes: created_at: 2023-08-03T12:44:55.266426 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- chain: 4
- draw: 1000
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- process_time_diff(chain, draw)float640.02484 0.03027 ... 0.02287 0.04276
array([[0.02484465, 0.03026824, 0.0275498 , ..., 0.02033344, 0.0246395 , 0.01992772], [0.03949065, 0.01371447, 0.02974776, ..., 0.01909351, 0.01244942, 0.02200291], [0.02418727, 0.02139096, 0.02423729, ..., 0.02853269, 0.02975177, 0.01429801], [0.04396229, 0.04085172, 0.03761578, ..., 0.03177199, 0.02286654, 0.04275852]])
- lp(chain, draw)float64351.2 354.5 353.6 ... 353.0 353.4
array([[351.15588188, 354.49917291, 353.62608315, ..., 352.73204341, 355.68605299, 354.62048964], [348.97647245, 358.33147522, 356.24118053, ..., 355.5669823 , 353.41596179, 349.40172304], [355.33330248, 354.11476965, 353.02275297, ..., 356.37076335, 355.23282234, 357.27840173], [355.46829925, 356.75307698, 352.46180604, ..., 354.23307794, 352.95911501, 353.3783564 ]])
- max_energy_error(chain, draw)float64-0.03674 0.03495 ... 0.2547 0.1954
array([[-0.03674255, 0.03494822, 0.3392237 , ..., 0.18039873, 0.26088542, 0.49621841], [ 0.13823553, -0.18089455, 0.03477711, ..., -0.14652744, -0.09281548, 0.35230766], [ 0.03755447, -0.0662405 , 0.03674591, ..., 0.1285427 , 0.01519062, 0.13618743], [ 0.30428744, -0.2446454 , -0.02112695, ..., -0.02632085, 0.25474352, 0.19543155]])
- step_size(chain, draw)float640.07388 0.07388 ... 0.06563 0.06563
array([[0.07388311, 0.07388311, 0.07388311, ..., 0.07388311, 0.07388311, 0.07388311], [0.0714658 , 0.0714658 , 0.0714658 , ..., 0.0714658 , 0.0714658 , 0.0714658 ], [0.08111642, 0.08111642, 0.08111642, ..., 0.08111642, 0.08111642, 0.08111642], [0.06562828, 0.06562828, 0.06562828, ..., 0.06562828, 0.06562828, 0.06562828]])
- reached_max_treedepth(chain, draw)boolFalse False False ... False False
array([[False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False]])
- perf_counter_diff(chain, draw)float640.02507 0.03056 ... 0.02366 0.04421
array([[0.02507298, 0.03056349, 0.02787485, ..., 0.02106091, 0.02582147, 0.02055384], [0.04002584, 0.01379189, 0.03068306, ..., 0.01909887, 0.0124605 , 0.02201315], [0.02420191, 0.02140715, 0.02440291, ..., 0.03021452, 0.03137594, 0.01493147], [0.05295574, 0.0466779 , 0.03982227, ..., 0.03293008, 0.02366477, 0.04420793]])
- step_size_bar(chain, draw)float640.07122 0.07122 ... 0.07193 0.07193
array([[0.07121798, 0.07121798, 0.07121798, ..., 0.07121798, 0.07121798, 0.07121798], [0.07661654, 0.07661654, 0.07661654, ..., 0.07661654, 0.07661654, 0.07661654], [0.06641153, 0.06641153, 0.06641153, ..., 0.06641153, 0.06641153, 0.06641153], [0.071931 , 0.071931 , 0.071931 , ..., 0.071931 , 0.071931 , 0.071931 ]])
- smallest_eigval(chain, draw)float64nan nan nan nan ... nan nan nan nan
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]])
- acceptance_rate(chain, draw)float640.9998 0.9916 ... 0.8754 0.9391
array([[0.99981323, 0.99156617, 0.86087927, ..., 0.9423506 , 0.9186242 , 0.83478816], [0.9490059 , 0.99982621, 0.98453782, ..., 0.98754873, 0.97686967, 0.86907628], [0.98896688, 0.99065932, 0.99177597, ..., 0.95280949, 0.99488913, 0.91630383], [0.88759452, 0.990825 , 0.99666295, ..., 0.99622014, 0.87535508, 0.93914408]])
- perf_counter_start(chain, draw)float641.442e+04 1.442e+04 ... 1.444e+04
array([[14416.2873664 , 14416.31274647, 14416.34360081, ..., 14441.42891612, 14441.45019966, 14441.47626545], [14410.82677097, 14410.86706909, 14410.88139978, ..., 14436.66757347, 14436.68689378, 14436.69960516], [14409.35345573, 14409.37791282, 14409.39967388, ..., 14435.89103034, 14435.92153093, 14435.95342922], [14412.12049024, 14412.173873 , 14412.22084097, ..., 14437.55382856, 14437.58701967, 14437.61093389]])
- energy_error(chain, draw)float64-0.01344 0.02138 ... 0.09381 0.1034
array([[-0.01343999, 0.02138095, 0.19201605, ..., 0.10314587, -0.12546418, -0.01132211], [ 0.13080946, -0.18089455, 0.02378239, ..., -0.01192367, -0.03935264, 0.15098095], [ 0.01433681, -0.00304629, 0.00828812, ..., -0.0148316 , 0.01148575, 0.04128621], [ 0.11345492, -0.20100975, 0.00464202, ..., -0.0210118 , 0.09381406, 0.10337544]])
- index_in_trajectory(chain, draw)int64-31 33 32 -28 43 ... 44 -9 38 29
array([[-31, 33, 32, ..., -37, -41, -32], [-12, 18, -10, ..., 33, -11, -23], [-15, -59, -10, ..., -38, 35, 14], [ 48, -28, -34, ..., -9, 38, 29]])
- energy(chain, draw)float64-344.9 -345.2 ... -345.0 -346.9
array([[-344.93870218, -345.16252633, -346.5464796 , ..., -345.62667989, -345.34434866, -345.90163116], [-333.85438495, -345.87424529, -349.06552382, ..., -352.20633888, -349.62907148, -341.99915893], [-344.87714537, -350.74551005, -345.76644887, ..., -348.45764188, -347.33084329, -349.89438043], [-349.3319774 , -344.49423483, -346.52038173, ..., -348.01416215, -344.95524551, -346.92667977]])
- largest_eigval(chain, draw)float64nan nan nan nan ... nan nan nan nan
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]])
- n_steps(chain, draw)float6463.0 63.0 63.0 ... 63.0 63.0 127.0
array([[ 63., 63., 63., ..., 63., 63., 63.], [ 63., 31., 63., ..., 63., 31., 63.], [ 63., 63., 63., ..., 63., 63., 31.], [ 63., 63., 63., ..., 63., 63., 127.]])
- tree_depth(chain, draw)int646 6 6 6 6 6 6 6 ... 6 6 6 6 6 6 6 7
array([[6, 6, 6, ..., 6, 6, 6], [6, 5, 6, ..., 6, 5, 6], [6, 6, 6, ..., 6, 6, 5], [6, 6, 6, ..., 6, 6, 7]])
- diverging(chain, draw)boolFalse False False ... False False
array([[False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False], [False, False, False, ..., False, False, False]])
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- created_at :
- 2023-08-03T12:44:52.608151
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
- sampling_time :
- 71.22048568725586
- tuning_steps :
- 1000
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 ... 994 995 996 997 998 999 Data variables: (12/17) process_time_diff (chain, draw) float64 0.02484 0.03027 ... 0.04276 lp (chain, draw) float64 351.2 354.5 ... 353.0 353.4 max_energy_error (chain, draw) float64 -0.03674 0.03495 ... 0.1954 step_size (chain, draw) float64 0.07388 0.07388 ... 0.06563 reached_max_treedepth (chain, draw) bool False False False ... False False perf_counter_diff (chain, draw) float64 0.02507 0.03056 ... 0.04421 ... ... index_in_trajectory (chain, draw) int64 -31 33 32 -28 43 ... 44 -9 38 29 energy (chain, draw) float64 -344.9 -345.2 ... -345.0 -346.9 largest_eigval (chain, draw) float64 nan nan nan nan ... nan nan nan n_steps (chain, draw) float64 63.0 63.0 63.0 ... 63.0 127.0 tree_depth (chain, draw) int64 6 6 6 6 6 6 6 6 ... 6 6 6 6 6 6 7 diverging (chain, draw) bool False False False ... False False Attributes: created_at: 2023-08-03T12:44:52.608151 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0 sampling_time: 71.22048568725586 tuning_steps: 1000
xarray.Dataset -
- chain: 1
- draw: 500
- channel: 2
- control: 3
- fourier_mode: 4
- date: 179
- chain(chain)int640
array([0])
- draw(draw)int640 1 2 3 4 5 ... 495 496 497 498 499
array([ 0, 1, 2, ..., 497, 498, 499])
- channel(channel)<U2'x1' 'x2'
array(['x1', 'x2'], dtype='<U2')
- control(control)<U7'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype='<U7')
- fourier_mode(fourier_mode)<U11'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='<U11')
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- beta_channel(chain, draw, channel)float641.418 1.277 0.1118 ... 4.541 1.259
array([[[1.41771409e+00, 1.27651456e+00], [1.11759230e-01, 1.00391763e+00], [1.66078493e+00, 3.20596683e+00], [3.20419089e+00, 9.87694132e-01], [3.55738705e+00, 1.17779302e+00], [1.57480200e+00, 2.96252368e-01], [3.26279685e+00, 1.56043278e+00], [1.27876003e+00, 1.10874401e-01], [3.54175229e-01, 7.43369192e-04], [2.38913960e+00, 1.67625422e+00], [1.75669724e+00, 5.02332389e-02], [2.38221787e-01, 2.21589489e+00], [1.15402728e+00, 1.28912514e+00], [9.01387641e-01, 2.27254179e+00], [1.97424890e+00, 7.10213090e-01], [3.01153104e+00, 1.13393205e-01], [1.80193915e+00, 2.84006410e-01], [1.94642714e+00, 9.86562999e-01], [2.61711365e-01, 2.05874501e+00], [6.98596308e-01, 2.07954266e-01], ... [1.95652787e+00, 3.39117422e-01], [2.91587674e+00, 3.44216753e-02], [3.36193824e+00, 1.02603402e-01], [8.08479560e-02, 1.91336022e+00], [2.23524656e+00, 1.10874283e+00], [1.07506579e+00, 7.46518481e-03], [2.78408481e+00, 5.37883336e-02], [2.21400711e+00, 4.95268830e-01], [6.58985171e-01, 2.47089791e+00], [3.60840473e-01, 1.27136115e-01], [1.39541597e+00, 7.09987659e-01], [2.12174075e-01, 1.75399689e+00], [4.06291582e+00, 9.94070662e-01], [6.66311684e-01, 9.11884110e-01], [1.71819776e+00, 6.33663220e-01], [4.54245357e+00, 1.15071969e+00], [1.07630282e+00, 2.20479058e+00], [1.81437141e+00, 1.11845209e+00], [4.54819610e+00, 7.58313974e-01], [4.54092801e+00, 1.25927449e+00]]])
- gamma_control(chain, draw, control)float643.665 4.476 ... -0.5954 -1.201
array([[[ 3.66482459, 4.47564534, 3.04612384], [ 1.8484942 , 2.98944803, 0.45814775], [-1.28487187, 0.87898241, 0.0175121 ], ..., [-0.08253424, 1.42715996, -2.11053585], [-0.33669504, 1.06259901, 2.12543441], [ 0.84511814, -0.59539134, -1.20094649]]])
- gamma_fourier(chain, draw, fourier_mode)float640.08728 -0.1613 ... -0.1337 2.467
array([[[ 0.08727895, -0.16125849, -0.71021385, -0.22046244], [-0.98480157, -0.09054815, -0.17627153, -2.0710989 ], [ 0.90703613, -0.41613027, 4.51566395, -0.68117658], ..., [ 3.34541511, -2.06247692, 0.15811203, 0.74631286], [ 2.17838564, -0.08109065, 0.59851762, -0.07118254], [-0.32304809, -0.14205784, -0.13366498, 2.46669554]]])
- channel_contributions(chain, draw, date, channel)float640.2905 0.0 0.119 ... 3.186 0.1076
array([[[[2.90516504e-01, 0.00000000e+00], [1.19033514e-01, 0.00000000e+00], [2.73228572e-01, 0.00000000e+00], ..., [1.66297245e-01, 6.33972700e-03], [2.64799160e-01, 4.44643693e-05], [4.08043786e-01, 3.11853158e-07]], [[5.97122984e-02, 0.00000000e+00], [3.73626697e-02, 0.00000000e+00], [6.21567495e-02, 0.00000000e+00], ..., [4.23183280e-02, 2.28024037e-01], [6.13012219e-02, 5.21924459e-02], [8.33391527e-02, 1.17514998e-02]], [[6.69743994e-01, 0.00000000e+00], [2.91719758e-01, 0.00000000e+00], [6.35954640e-01, 0.00000000e+00], ..., ... ..., [2.32507655e-01, 1.30642798e-02], [3.74909454e-01, 1.20254676e-04], [5.75214477e-01, 1.10687536e-06]], [[1.19848505e+00, 0.00000000e+00], [1.03161115e+00, 0.00000000e+00], [1.58491311e+00, 0.00000000e+00], ..., [1.09437874e+00, 4.19086610e-02], [1.57037339e+00, 2.10121606e-03], [2.28147106e+00, 1.05243910e-04]], [[2.26777159e+00, 0.00000000e+00], [1.35144884e+00, 0.00000000e+00], [2.32981154e+00, 0.00000000e+00], ..., [1.56231581e+00, 2.35940757e-01], [2.29549604e+00, 1.78506809e-01], [3.18647033e+00, 1.07580873e-01]]]])
- intercept(chain, draw)float64-3.807 -1.606 ... 0.5065 -0.598
array([[-3.80664915e+00, -1.60632722e+00, -2.23287081e+00, 1.09787805e+00, 1.76016341e+00, -1.88967081e+00, 6.07935349e-01, -3.79703233e+00, -1.15201037e+00, -1.18584205e+00, -8.74344532e-01, 2.56337702e+00, 3.46090683e+00, -3.77754708e-01, 2.04130032e-01, 1.17706005e+00, 1.74583787e+00, 7.49616077e-01, -4.60983997e-01, -1.81504417e+00, 5.85722649e+00, 8.86564381e-01, -1.20215513e+00, 2.94730415e-01, 6.09652104e-01, 9.11875687e-01, -4.38947169e+00, 6.89550084e-01, 2.06504837e+00, 2.74654396e+00, 2.38194873e+00, -8.94219085e-01, 3.91248163e+00, -3.29180346e+00, -1.34649410e+00, 8.96551388e-02, -2.90473580e+00, 9.72659269e-02, -1.92257214e+00, -1.11137240e+00, -2.81659882e+00, -5.70027859e+00, 3.62964271e-01, -2.80605209e-01, -2.22468885e+00, 1.98732785e+00, 2.84508165e+00, 2.96739935e+00, -3.48394576e+00, -2.02887307e-01, -1.34791202e+00, -1.83266609e+00, -1.85205186e+00, 1.95568463e-01, -1.17267467e+00, -1.03010248e+00, 7.57360596e-01, -2.01141799e-01, 5.62960478e-01, 2.60324891e+00, ... 1.12283454e+00, -3.26569052e+00, 1.53617785e+00, -2.39620849e+00, 1.59846579e+00, -5.76747003e-01, -1.46739063e+00, -5.24181191e-02, 1.40837723e+00, -6.04833598e-01, 1.59690714e+00, 1.64638048e+00, -1.00528212e+00, 2.28411724e-01, -4.10244097e-01, 4.84134507e-01, 1.93437760e+00, 7.88297884e-01, -1.61553946e+00, 3.04686958e-01, -2.70082064e+00, -6.14513806e+00, -7.08004311e-01, -3.02819776e+00, 1.23298734e+00, -4.19611758e+00, 4.87400474e-01, 6.07163435e+00, 1.68453167e+00, 3.98394979e+00, 2.15590303e+00, 7.25736371e-01, -4.07869462e+00, 3.34821766e+00, 2.54238707e+00, -2.22477173e+00, -3.74008812e-01, 8.12533823e-01, 1.49192715e+00, 5.33468705e+00, 1.00686622e+00, 4.50202281e+00, -6.06565848e-01, -2.36020627e-02, 1.97849581e+00, 1.93642563e+00, -3.92982412e-01, 1.78024985e+00, -2.67678489e+00, 9.31340283e-01, 1.95256632e+00, -1.49401961e+00, 3.05449438e-01, 1.20708956e+00, -1.03227911e+00, -1.60946736e+00, -5.37938207e-01, 5.06498336e-01, -5.98010433e-01]])
- lam(chain, draw, channel)float641.372 1.643 4.847 ... 4.326 1.678
array([[[ 1.3720548 , 1.64270887], [ 4.846812 , 3.05089387], [ 2.85303097, 5.08265535], [ 3.90491712, 3.31338982], [ 4.45846384, 5.23646078], [ 1.69843734, 0.76837954], [ 8.02190759, 1.38004493], [ 3.27879988, 6.93637645], [ 3.28594503, 3.58567522], [ 1.98321149, 1.22753861], [ 9.93484412, 1.20314043], [ 1.62539436, 4.38944859], [ 5.96540237, 3.92421575], [ 8.17722329, 3.87321514], [ 5.12222528, 0.70012007], [ 3.37302323, 3.22603354], [ 2.19312691, 2.65267639], [ 2.86273852, 1.68693716], [ 1.64905395, 1.4875959 ], [ 4.58132884, 0.89593771], ... [ 1.51059352, 5.06906401], [ 3.8870985 , 0.71907266], [ 1.14851796, 2.68981586], [ 1.93168394, 8.08156595], [ 3.2294571 , 2.62666351], [ 4.51877697, 10.45226595], [ 2.69223401, 1.05351076], [ 1.32245143, 4.03977294], [ 1.46040357, 5.91052485], [ 1.99781008, 2.19104807], [ 3.46922155, 1.32888381], [ 5.46855487, 2.52903811], [ 1.56943531, 3.5625068 ], [ 0.58488156, 5.23058655], [ 0.67068795, 1.35500863], [ 3.02724692, 0.96446992], [ 4.70293848, 2.71689166], [ 1.49472697, 2.9505339 ], [ 3.38065588, 2.67843913], [ 4.32638843, 1.67784475]]])
- fourier_contributions(chain, draw, date, fourier_mode)float640.08727 0.001907 ... -0.1191 -1.122
array([[[[ 8.72728473e-02, 1.90710586e-03, 1.67973582e-02, 2.20400771e-01], [ 8.65168790e-02, 2.12633047e-02, 1.85660127e-01, 2.12796226e-01], [ 8.45079099e-02, 4.03115527e-02, 3.43806375e-01, 1.92908831e-01], ..., [-6.14162260e-02, 1.14577316e-01, -7.10180605e-01, -2.13324140e-03], [-6.84209213e-02, 1.00116429e-01, -6.91323474e-01, 5.05089279e-02], [-7.44346945e-02, 8.42055818e-02, -6.32562335e-01, 1.00235662e-01]], [[-9.84732695e-01, 1.07085783e-03, 4.16902030e-03, 2.07051955e+00], [-9.76202818e-01, 1.19395450e-02, 4.60799150e-02, 1.99907988e+00], [-9.53534856e-01, 2.26353150e-02, 8.53310226e-02, 1.81225095e+00], ... [-1.53288076e+00, 5.76164940e-02, 5.98489602e-01, -6.88777354e-04], [-1.70771017e+00, 5.03446739e-02, 5.82598156e-01, 1.63082367e-02], [-1.85780727e+00, 4.23437254e-02, 5.33078456e-01, 3.23639201e-02]], [[-3.23025497e-01, 1.68003148e-03, 3.16132753e-03, -2.46600554e+00], [-3.20227410e-01, 1.87315356e-02, 3.49419512e-02, -2.38092031e+00], [-3.12791555e-01, 3.55117558e-02, 6.47056842e-02, -2.15840554e+00], ..., [ 2.27321642e-01, 1.00934878e-01, -1.33658726e-01, 2.38682700e-02], [ 2.53248322e-01, 8.81958130e-02, -1.30109741e-01, -5.65130945e-01], [ 2.75507273e-01, 7.41794314e-02, -1.19050668e-01, -1.12151013e+00]]]])
- mu(chain, draw, date)float64-3.19 -0.1353 ... -211.0 -212.0
array([[[-3.18975456e+00, -1.35255259e-01, 3.22036177e+00, ..., 5.31824631e+02, 5.35012995e+02, 5.38288883e+02], [-4.55588189e-01, -2.99202865e-02, 3.38807451e-01, ..., 7.98590339e+01, 8.07304683e+01, 8.17108687e+01], [-7.70473012e-02, -1.49262202e+00, -2.16956752e+00, ..., 6.02611008e+00, 5.64451201e+00, 5.57311356e+00], ..., [ 2.50462802e+00, 3.32252116e-01, -1.34367874e+00, ..., -3.72470012e+02, -3.75086938e+02, -3.77612712e+02], [ 3.94118271e+00, 5.74584772e+00, 8.24432548e+00, ..., 3.74841779e+02, 3.77222405e+02, 3.79865379e+02], [-1.11442852e+00, -3.09498232e+00, -3.04107153e+00, ..., -2.09947870e+02, -2.11045333e+02, -2.11963308e+02]]])
- alpha(chain, draw, channel)float640.0521 0.007014 ... 0.2067 0.7527
array([[[5.21036437e-02, 7.01355180e-03], [2.30438776e-01, 2.25101248e-01], [6.23615608e-02, 9.70097144e-02], [1.32889237e-01, 5.00559550e-02], [2.86399158e-01, 4.86702102e-01], [1.12476609e-01, 1.38874353e-01], [4.38830006e-02, 2.27186361e-01], [2.17255274e-02, 1.14369354e-01], [3.13171807e-01, 3.25342124e-01], [8.69289147e-02, 3.13357452e-01], [4.80242501e-01, 5.57627343e-02], [2.99680856e-01, 3.66054083e-01], [4.38263867e-01, 4.03405202e-01], [3.77285663e-01, 3.77672564e-01], [6.12790820e-01, 1.64399192e-01], [6.03679618e-01, 2.86299055e-01], [4.38487606e-02, 2.33547181e-01], [5.70323120e-01, 4.12761879e-01], [3.35988660e-01, 8.92219801e-02], [2.01030313e-01, 3.47716797e-01], ... [3.96006546e-01, 2.27202810e-03], [2.61246602e-01, 3.87195477e-01], [1.65564394e-01, 1.71122640e-01], [1.46161941e-01, 5.36758595e-02], [3.77553319e-01, 5.30462189e-01], [5.67577800e-01, 5.01400248e-01], [2.31180070e-01, 2.93754129e-01], [1.48999380e-01, 2.05110752e-01], [2.66807613e-01, 1.60311428e-01], [6.70239481e-02, 2.47008160e-01], [2.38660236e-01, 4.32334615e-01], [3.91907264e-01, 5.43144348e-01], [3.86505130e-01, 5.99591575e-01], [1.16866568e-01, 2.04606808e-01], [4.63992803e-02, 3.68704752e-01], [6.13431324e-02, 5.71710076e-01], [2.74840902e-01, 3.33923134e-01], [6.20565186e-03, 9.20442678e-03], [5.02552224e-01, 5.00870321e-02], [2.06731998e-01, 7.52706120e-01]]])
- channel_adstock(chain, draw, date, channel)float640.303 0.0 0.1227 ... 0.4025 0.1021
array([[[[3.02993403e-01, 0.00000000e+00], [1.22676900e-01, 0.00000000e+00], [2.84486416e-01, 0.00000000e+00], ..., [1.71774527e-01, 6.04669065e-03], [2.75495702e-01, 4.24087781e-05], [4.31740283e-01, 2.97436162e-07]], [[2.45990848e-01, 0.00000000e+00], [1.43466340e-01, 0.00000000e+00], [2.58836419e-01, 0.00000000e+00], ..., [1.64433866e-01, 1.51539656e-01], [2.54290758e-01, 3.41117657e-02], [3.97458806e-01, 7.67393208e-03]], [[2.99714478e-01, 0.00000000e+00], [1.24423765e-01, 0.00000000e+00], [2.82844288e-01, 0.00000000e+00], ..., ... ..., [1.72414420e-01, 7.91803150e-03], [2.80521945e-01, 7.28809412e-05], [4.39336977e-01, 6.70827287e-07]], [[1.59657898e-01, 0.00000000e+00], [1.36560453e-01, 0.00000000e+00], [2.15166550e-01, 0.00000000e+00], ..., [1.45196794e-01, 4.13090949e-02], [2.13016631e-01, 2.06904996e-03], [3.26250262e-01, 1.03632537e-04]], [[2.53567568e-01, 0.00000000e+00], [1.41873951e-01, 0.00000000e+00], [2.62060189e-01, 0.00000000e+00], ..., [1.65811029e-01, 2.26006913e-01], [2.57343254e-01, 1.70116787e-01], [4.02499945e-01, 1.02082852e-01]]]])
- control_contributions(chain, draw, date, control)float640.0 0.0 0.0 0.0 ... 0.0 -0.0 -213.8
array([[[[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 3.04612384e+00], [ 0.00000000e+00, 0.00000000e+00, 6.09224768e+00], ..., [ 0.00000000e+00, 0.00000000e+00, 5.36117796e+02], [ 0.00000000e+00, 0.00000000e+00, 5.39163920e+02], [ 0.00000000e+00, 0.00000000e+00, 5.42210044e+02]], [[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [ 0.00000000e+00, 0.00000000e+00, 4.58147748e-01], [ 0.00000000e+00, 0.00000000e+00, 9.16295495e-01], ..., [ 0.00000000e+00, 0.00000000e+00, 8.06340036e+01], [ 0.00000000e+00, 0.00000000e+00, 8.10921513e+01], [ 0.00000000e+00, 0.00000000e+00, 8.15502991e+01]], [[-0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [-0.00000000e+00, 0.00000000e+00, 1.75121026e-02], [-0.00000000e+00, 0.00000000e+00, 3.50242052e-02], ..., ... ..., [-0.00000000e+00, 0.00000000e+00, -3.71454309e+02], [-0.00000000e+00, 0.00000000e+00, -3.73564845e+02], [-0.00000000e+00, 0.00000000e+00, -3.75675381e+02]], [[-0.00000000e+00, 0.00000000e+00, 0.00000000e+00], [-0.00000000e+00, 0.00000000e+00, 2.12543441e+00], [-0.00000000e+00, 0.00000000e+00, 4.25086882e+00], ..., [-0.00000000e+00, 0.00000000e+00, 3.74076456e+02], [-0.00000000e+00, 0.00000000e+00, 3.76201891e+02], [-0.00000000e+00, 0.00000000e+00, 3.78327325e+02]], [[ 0.00000000e+00, -0.00000000e+00, -0.00000000e+00], [ 0.00000000e+00, -0.00000000e+00, -1.20094649e+00], [ 0.00000000e+00, -0.00000000e+00, -2.40189298e+00], ..., [ 0.00000000e+00, -0.00000000e+00, -2.11366582e+02], [ 0.00000000e+00, -0.00000000e+00, -2.12567529e+02], [ 0.00000000e+00, -0.00000000e+00, -2.13768475e+02]]]])
- channel_adstock_saturated(chain, draw, date, channel)float640.2049 0.0 ... 0.7017 0.08543
array([[[[2.04918965e-01, 0.00000000e+00], [8.39615789e-02, 0.00000000e+00], [1.92724734e-01, 0.00000000e+00], ..., [1.17299564e-01, 4.96643534e-03], [1.86778958e-01, 3.48326379e-05], [2.87818107e-01, 2.44300510e-07]], [[5.34294109e-01, 0.00000000e+00], [3.34313950e-01, 0.00000000e+00], [5.56166586e-01, 0.00000000e+00], ..., [3.78656223e-01, 2.27134210e-01], [5.48511490e-01, 5.19887733e-02], [7.45702637e-01, 1.17056415e-02]], [[4.03269552e-01, 0.00000000e+00], [1.75651737e-01, 0.00000000e+00], [3.82924140e-01, 0.00000000e+00], ..., ... ..., [1.28147773e-01, 1.16806789e-02], [2.06633247e-01, 1.07518843e-04], [3.17032376e-01, 9.89649326e-07]], [[2.63507779e-01, 0.00000000e+00], [2.26817650e-01, 0.00000000e+00], [3.48470707e-01, 0.00000000e+00], ..., [2.40618196e-01, 5.52655791e-02], [3.45273896e-01, 2.77090510e-03], [5.01621085e-01, 1.38786721e-04]], [[4.99407079e-01, 0.00000000e+00], [2.97615120e-01, 0.00000000e+00], [5.13069472e-01, 0.00000000e+00], ..., [3.44052098e-01, 1.87362453e-01], [5.05512537e-01, 1.41753693e-01], [7.01722274e-01, 8.54308364e-02]]]])
- sigma(chain, draw)float642.361 0.3904 3.818 ... 1.618 2.132
array([[2.36139233e+00, 3.90383666e-01, 3.81810433e+00, 1.59228477e+00, 2.43287675e+00, 5.48688025e-01, 1.56404487e+00, 1.31355316e+00, 1.28073124e+00, 4.40476811e-01, 1.78472661e+00, 3.15452436e-01, 1.27223844e+00, 2.75881580e+00, 1.84174204e+00, 1.04928691e+00, 8.87666065e-01, 2.76698391e+00, 3.93845643e-01, 1.63368287e+00, 7.61037943e-02, 4.12874596e+00, 5.21084915e-01, 7.39823091e-01, 4.41424800e-01, 4.29048289e-01, 3.34041839e+00, 1.25542616e+00, 4.57817916e-01, 8.80044727e-02, 1.06574419e+00, 1.76564681e+00, 2.27690976e+00, 1.89041265e-01, 8.88635101e-01, 1.33432244e+00, 7.80132690e-01, 1.95030689e+00, 8.10445679e-02, 2.24252401e+00, 1.91362347e+00, 3.29182182e+00, 1.05085213e+00, 2.59973995e+00, 1.63127745e+00, 6.20303474e-01, 2.49304481e+00, 8.57753406e-01, 3.20653855e+00, 6.30675444e-01, 6.20743332e-01, 1.11855341e+00, 2.62139702e+00, 5.34450725e-01, 1.64068248e+00, 3.31066988e-01, 1.57005887e+00, 2.17213393e-01, 9.41021038e-01, 1.81911249e+00, 3.38399559e+00, 1.51783639e+00, 2.61623802e+00, 4.87073540e+00, 1.63012547e+00, 4.09254852e+00, 7.52662003e+00, 7.35085773e-01, 3.59411170e-02, 2.80230892e+00, 8.94506226e-01, 1.24053879e+00, 1.82747678e+00, 2.09934339e+00, 7.88664727e-01, 4.26916806e-01, 1.14983676e+00, 1.23654745e+00, 1.17428296e-01, 7.81700139e-01, ... 2.13547357e-01, 1.01120588e+00, 1.30672122e+00, 4.49044393e+00, 1.91281676e+00, 4.96142187e+00, 4.98708791e-01, 1.07024576e-01, 4.17915453e-01, 3.81704808e+00, 1.16261074e+00, 7.22723755e-01, 1.86201283e+00, 1.86799111e-02, 1.24527650e+00, 5.46664954e-01, 3.26748238e-01, 1.92515845e+00, 1.01162172e+00, 2.18129768e+00, 2.79981680e+00, 1.95991477e+00, 1.54909445e+00, 6.11864595e-01, 1.86990892e+00, 2.51808836e+00, 2.07057185e+00, 1.60235293e-01, 3.25929283e-03, 2.93356848e+00, 2.30708361e+00, 2.96932167e+00, 1.99290693e-01, 1.66111871e+00, 1.47008512e-01, 9.69815960e-01, 4.34889374e-01, 1.23820793e+00, 4.86462445e-02, 2.93094018e+00, 3.78169300e+00, 3.82458453e-01, 3.08544739e+00, 2.47101876e+00, 1.48976925e+00, 8.72392926e-01, 4.12272884e+00, 1.16544319e+00, 1.41737129e+00, 2.02377787e+00, 2.06537114e+00, 1.51758571e+00, 1.67072208e+00, 3.36114202e-01, 1.48194524e+00, 1.40764682e+00, 2.67283019e+00, 1.01490958e+00, 2.27160853e+00, 1.78060168e+00, 4.87138762e-01, 2.75654088e+00, 1.56972219e+00, 1.71478319e+00, 3.13686584e-01, 2.69815336e+00, 3.14442335e+00, 2.64495073e+00, 1.87525957e-01, 1.95837070e+00, 5.28574288e-01, 1.10559983e+00, 1.44656343e+00, 5.90947836e-01, 2.50629194e+00, 1.50253993e+00, 2.56187286e-01, 8.14324408e-01, 1.61842954e+00, 2.13182998e+00]])
- chainPandasIndex
PandasIndex(Index([0], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 490, 491, 492, 493, 494, 495, 496, 497, 498, 499], dtype='int64', name='draw', length=500))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:54.644498
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (chain: 1, draw: 500, channel: 2, control: 3, fourier_mode: 4, date: 179) Coordinates: * chain (chain) int64 0 * draw (draw) int64 0 1 2 3 4 5 ... 495 496 497 498 499 * channel (channel) <U2 'x1' 'x2' * control (control) <U7 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) <U11 'sin_order_1' ... 'cos_ord... * date (date) <U10 '2018-04-02' ... '2021-08-30' Data variables: (12/13) beta_channel (chain, draw, channel) float64 1.418 ... 1.259 gamma_control (chain, draw, control) float64 3.665 ... -1.201 gamma_fourier (chain, draw, fourier_mode) float64 0.08728 ..... channel_contributions (chain, draw, date, channel) float64 0.2905 ..... intercept (chain, draw) float64 -3.807 -1.606 ... -0.598 lam (chain, draw, channel) float64 1.372 ... 1.678 ... ... mu (chain, draw, date) float64 -3.19 ... -212.0 alpha (chain, draw, channel) float64 0.0521 ... 0.7527 channel_adstock (chain, draw, date, channel) float64 0.303 ...... control_contributions (chain, draw, date, control) float64 0.0 ... -... channel_adstock_saturated (chain, draw, date, channel) float64 0.2049 ..... sigma (chain, draw) float64 2.361 0.3904 ... 2.132 Attributes: created_at: 2023-08-03T12:44:54.644498 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- chain: 1
- draw: 500
- date: 179
- chain(chain)int640
array([0])
- draw(draw)int640 1 2 3 4 5 ... 495 496 497 498 499
array([ 0, 1, 2, ..., 497, 498, 499])
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- likelihood(chain, draw, date)float64-7.646 -1.949 ... -210.5 -215.1
array([[[-7.64565815e+00, -1.94922477e+00, 2.89341956e+00, ..., 5.34868436e+02, 5.33969792e+02, 5.34099399e+02], [-6.65431494e-01, -1.43483037e-02, 2.20785878e-01, ..., 8.01458595e+01, 8.06515086e+01, 8.17227111e+01], [-3.15327903e+00, -2.96584709e+00, 1.77266105e+00, ..., 5.26393093e+00, 3.33724083e+00, 7.52370386e+00], ..., [ 4.21129384e+00, 1.18911955e-01, -1.45744539e+00, ..., -3.74089980e+02, -3.75432384e+02, -3.77886954e+02], [ 6.74795441e+00, 3.22809699e+00, 7.94359098e+00, ..., 3.75099611e+02, 3.76135254e+02, 3.81491191e+02], [-2.15351790e+00, -3.42633927e+00, -1.33782504e-01, ..., -2.09302513e+02, -2.10516049e+02, -2.15109385e+02]]])
- chainPandasIndex
PandasIndex(Index([0], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 490, 491, 492, 493, 494, 495, 496, 497, 498, 499], dtype='int64', name='draw', length=500))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:54.650068
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (chain: 1, draw: 500, date: 179) Coordinates: * chain (chain) int64 0 * draw (draw) int64 0 1 2 3 4 5 6 7 ... 492 493 494 495 496 497 498 499 * date (date) <U10 '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (chain, draw, date) float64 -7.646 -1.949 ... -210.5 -215.1 Attributes: created_at: 2023-08-03T12:44:54.650068 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- date: 179
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- likelihood(date)float640.4794 0.4527 ... 0.5388 0.5625
array([0.47936319, 0.45268134, 0.53738551, 0.46487367, 0.5343368 , 0.44239849, 0.60963642, 0.73132832, 0.59600126, 0.70565315, 0.61313561, 0.60049297, 0.56404656, 0.78633605, 0.60523784, 0.5580442 , 0.50641716, 0.586594 , 0.46992062, 0.42705583, 0.48426812, 0.38777049, 0.49079078, 0.50002789, 0.44113299, 0.46359002, 0.42484345, 0.42573496, 0.5126282 , 0.55393305, 0.7801562 , 0.61244259, 0.7820166 , 0.7741081 , 0.87677312, 0.63147717, 0.51546283, 0.71272935, 0.93485537, 0.79408756, 0.72228448, 0.68098843, 0.70650313, 0.73308506, 0.4805125 , 0.57954561, 0.93776656, 0.77561424, 0.66655529, 0.59547518, 0.76751515, 0.57312122, 0.50621332, 0.5308715 , 0.52295261, 0.79720566, 0.62933004, 0.46812934, 0.81995741, 0.60723799, 0.63667661, 0.63023046, 0.60081393, 0.54905189, 0.54151156, 0.67785878, 0.580275 , 0.48759392, 0.54008438, 0.7454827 , 0.4601459 , 0.47552149, 0.71676648, 0.72228072, 0.54215422, 0.85509146, 0.684061 , 0.56992491, 0.84343218, 0.58108193, 0.57400488, 0.7216718 , 0.70124307, 0.70769822, 0.89872242, 0.8115083 , 0.72059583, 0.57222288, 1. , 0.8366832 , 0.99252741, 0.70215306, 0.69084327, 0.71206105, 0.64219547, 0.81993542, 0.6896944 , 0.59591584, 0.6458122 , 0.50792736, 0.55944542, 0.63420523, 0.70343723, 0.7740387 , 0.70204359, 0.65253863, 0.81871625, 0.81911895, 0.68380948, 0.71220965, 0.54236837, 0.58017503, 0.6352586 , 0.62739849, 0.92329765, 0.85928619, 0.62316379, 0.56392299, 0.55616857, 0.4257328 , 0.43070972, 0.52466263, 0.54561821, 0.5264453 , 0.41628475, 0.49557027, 0.53537871, 0.53003824, 0.78154262, 0.53440301, 0.63876159, 0.59727845, 0.57348504, 0.72266752, 0.88323619, 0.67509495, 0.68280629, 0.76882837, 0.66983526, 0.70381398, 0.73990877, 0.6594292 , 0.83865735, 0.73595323, 0.56852858, 0.62116609, 0.52960021, 0.67721404, 0.65454618, 0.57113219, 0.55954835, 0.68309668, 0.64092662, 0.61267177, 0.58478466, 0.63905178, 0.56741971, 0.58706316, 0.63111636, 0.84924442, 0.89647751, 0.84943539, 0.68420327, 0.82623974, 0.70912897, 0.66280698, 0.60631287, 0.61775646, 0.54565334, 0.52431821, 0.77688667, 0.53243718, 0.45656482, 0.38411009, 0.42749903, 0.66954245, 0.49776812, 0.53883804, 0.56252938])
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:52.612711
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (date: 179) Coordinates: * date (date) <U10 '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (date) float64 0.4794 0.4527 0.5374 ... 0.4978 0.5388 0.5625 Attributes: created_at: 2023-08-03T12:44:52.612711 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- date: 179
- channel: 2
- control: 3
- fourier_mode: 4
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- channel(channel)<U2'x1' 'x2'
array(['x1', 'x2'], dtype='<U2')
- control(control)<U7'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype='<U7')
- fourier_mode(fourier_mode)<U11'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='<U11')
- channel_data(date, channel)float640.3196 0.0 0.1128 ... 0.4403 0.0
array([[3.19648241e-01, 0.00000000e+00], [1.12765324e-01, 0.00000000e+00], [2.93380707e-01, 0.00000000e+00], [7.16379574e-02, 0.00000000e+00], [3.88041940e-01, 0.00000000e+00], [4.73291540e-02, 0.00000000e+00], [4.25671645e-01, 0.00000000e+00], [3.35039834e-01, 8.84759215e-01], [2.53918849e-01, 0.00000000e+00], [9.41199784e-01, 0.00000000e+00], [4.26630818e-01, 0.00000000e+00], [3.64360623e-01, 0.00000000e+00], [4.42975062e-01, 0.00000000e+00], [4.24530352e-01, 9.69641782e-01], [3.29493374e-01, 0.00000000e+00], [9.25031639e-01, 0.00000000e+00], [3.09048312e-01, 0.00000000e+00], [9.12534800e-01, 0.00000000e+00], [2.51539259e-01, 0.00000000e+00], [2.37266186e-01, 0.00000000e+00], ... [1.70332079e-01, 9.38381372e-01], [4.29038699e-01, 9.20516422e-01], [9.13487319e-01, 0.00000000e+00], [1.42944657e-01, 0.00000000e+00], [1.86838303e-01, 8.55514629e-01], [3.14421972e-01, 0.00000000e+00], [4.00678428e-01, 0.00000000e+00], [1.45069138e-01, 0.00000000e+00], [1.48471239e-01, 0.00000000e+00], [7.00777532e-02, 0.00000000e+00], [1.99103276e-01, 0.00000000e+00], [3.62700587e-01, 9.14000224e-01], [2.41143360e-01, 0.00000000e+00], [4.05094103e-02, 0.00000000e+00], [6.76832332e-02, 0.00000000e+00], [3.31349195e-02, 0.00000000e+00], [1.66170470e-01, 8.68233263e-01], [1.72458556e-01, 0.00000000e+00], [2.81197012e-01, 0.00000000e+00], [4.40328682e-01, 0.00000000e+00]])
- target(date)float640.4794 0.4527 ... 0.5388 0.5625
array([0.47936319, 0.45268134, 0.53738551, 0.46487367, 0.5343368 , 0.44239849, 0.60963642, 0.73132832, 0.59600126, 0.70565315, 0.61313561, 0.60049297, 0.56404656, 0.78633605, 0.60523784, 0.5580442 , 0.50641716, 0.586594 , 0.46992062, 0.42705583, 0.48426812, 0.38777049, 0.49079078, 0.50002789, 0.44113299, 0.46359002, 0.42484345, 0.42573496, 0.5126282 , 0.55393305, 0.7801562 , 0.61244259, 0.7820166 , 0.7741081 , 0.87677312, 0.63147717, 0.51546283, 0.71272935, 0.93485537, 0.79408756, 0.72228448, 0.68098843, 0.70650313, 0.73308506, 0.4805125 , 0.57954561, 0.93776656, 0.77561424, 0.66655529, 0.59547518, 0.76751515, 0.57312122, 0.50621332, 0.5308715 , 0.52295261, 0.79720566, 0.62933004, 0.46812934, 0.81995741, 0.60723799, 0.63667661, 0.63023046, 0.60081393, 0.54905189, 0.54151156, 0.67785878, 0.580275 , 0.48759392, 0.54008438, 0.7454827 , 0.4601459 , 0.47552149, 0.71676648, 0.72228072, 0.54215422, 0.85509146, 0.684061 , 0.56992491, 0.84343218, 0.58108193, 0.57400488, 0.7216718 , 0.70124307, 0.70769822, 0.89872242, 0.8115083 , 0.72059583, 0.57222288, 1. , 0.8366832 , 0.99252741, 0.70215306, 0.69084327, 0.71206105, 0.64219547, 0.81993542, 0.6896944 , 0.59591584, 0.6458122 , 0.50792736, 0.55944542, 0.63420523, 0.70343723, 0.7740387 , 0.70204359, 0.65253863, 0.81871625, 0.81911895, 0.68380948, 0.71220965, 0.54236837, 0.58017503, 0.6352586 , 0.62739849, 0.92329765, 0.85928619, 0.62316379, 0.56392299, 0.55616857, 0.4257328 , 0.43070972, 0.52466263, 0.54561821, 0.5264453 , 0.41628475, 0.49557027, 0.53537871, 0.53003824, 0.78154262, 0.53440301, 0.63876159, 0.59727845, 0.57348504, 0.72266752, 0.88323619, 0.67509495, 0.68280629, 0.76882837, 0.66983526, 0.70381398, 0.73990877, 0.6594292 , 0.83865735, 0.73595323, 0.56852858, 0.62116609, 0.52960021, 0.67721404, 0.65454618, 0.57113219, 0.55954835, 0.68309668, 0.64092662, 0.61267177, 0.58478466, 0.63905178, 0.56741971, 0.58706316, 0.63111636, 0.84924442, 0.89647751, 0.84943539, 0.68420327, 0.82623974, 0.70912897, 0.66280698, 0.60631287, 0.61775646, 0.54565334, 0.52431821, 0.77688667, 0.53243718, 0.45656482, 0.38411009, 0.42749903, 0.66954245, 0.49776812, 0.53883804, 0.56252938])
- control_data(date, control)float640.0 0.0 0.0 0.0 ... 0.0 0.0 178.0
array([[ 0., 0., 0.], [ 0., 0., 1.], [ 0., 0., 2.], [ 0., 0., 3.], [ 0., 0., 4.], [ 0., 0., 5.], [ 0., 0., 6.], [ 0., 0., 7.], [ 0., 0., 8.], [ 0., 0., 9.], [ 0., 0., 10.], [ 0., 0., 11.], [ 0., 0., 12.], [ 0., 0., 13.], [ 0., 0., 14.], [ 0., 0., 15.], [ 0., 0., 16.], [ 0., 0., 17.], [ 0., 0., 18.], [ 0., 0., 19.], ... [ 0., 0., 159.], [ 0., 0., 160.], [ 0., 0., 161.], [ 0., 0., 162.], [ 0., 0., 163.], [ 0., 0., 164.], [ 0., 0., 165.], [ 0., 0., 166.], [ 0., 0., 167.], [ 0., 0., 168.], [ 0., 0., 169.], [ 0., 0., 170.], [ 0., 0., 171.], [ 0., 0., 172.], [ 0., 0., 173.], [ 0., 0., 174.], [ 0., 0., 175.], [ 0., 0., 176.], [ 0., 0., 177.], [ 0., 0., 178.]])
- fourier_data(date, fourier_mode)float640.9999 -0.01183 ... 0.8907 -0.4547
array([[ 0.99993007, -0.01182639, -0.02365113, -0.99972027], [ 0.99126855, -0.13185852, -0.2614144 , -0.96522666], [ 0.96825075, -0.24998097, -0.48408852, -0.87501903], [ 0.93121004, -0.36448301, -0.67882048, -0.73430426], [ 0.88068286, -0.47370635, -0.83437012, -0.55120459], [ 0.81740098, -0.57606912, -0.94175893, -0.33628873], [ 0.74228091, -0.67008884, -0.99478831, -0.10196189], [ 0.65641058, -0.75440384, -0.99039732, 0.13825031], [ 0.56103363, -0.82779301, -0.92883943, 0.37048254], [ 0.45753138, -0.88919348, -0.81366783, 0.58133008], [ 0.34740282, -0.93771599, -0.65153036, 0.75862256], [ 0.23224292, -0.97265781, -0.45178579, 0.89212645], [ 0.11371951, -0.99351289, -0.22596361, 0.97413574], [-0.00645086, -0.99997919, 0.01290146, 0.99991677], [-0.12652782, -0.99196306, 0.25102184, 0.96798142], [-0.2447723 , -0.96958059, 0.47465294, 0.88017304], [-0.35947181, -0.93315594, 0.67088652, 0.74156003], [-0.46896519, -0.88321665, 0.82839573, 0.5601433 ], [-0.57166667, -0.82048596, 0.93808896, 0.34639443], [-0.66608886, -0.7458724 , 0.99363459, 0.11265127], ... [ 0.95406655, -0.29959476, -0.57166667, -0.82048596], [ 0.91116864, -0.41203364, -0.75086425, -0.66045656], [ 0.85507451, -0.51850514, -0.88672106, -0.46230483], [ 0.78659657, -0.61746728, -0.97139528, -0.23746832], [ 0.70672656, -0.7074868 , -0.99999942, 0.00107515], [ 0.61662121, -0.78725998, -0.97088241, 0.23955656], [ 0.51758551, -0.85563149, -0.88572492, 0.46421048], [ 0.41105375, -0.91161111, -0.74944234, 0.66206962], [ 0.29856882, -0.95438811, -0.56990106, 0.82171332], [ 0.18175979, -0.98334296, -0.35746442, 0.93392676], [ 0.06231838, -0.99805632, -0.1243945 , 0.99223284], [-0.05802557, -0.9983151 , 0.1158556 , 0.99326607], [-0.17752915, -0.98411554, 0.34941839, 0.9369668 ], [-0.29446162, -0.95566331, 0.56281233, 0.82658471], [-0.40712949, -0.91337045, 0.74372008, 0.66849116], [-0.513901 , -0.8578495 , 0.88169943, 0.47181152], [-0.61322983, -0.78990454, 0.96878605, 0.24789836], [-0.70367741, -0.7105196 , 0.99995318, 0.00967621], [-0.78393382, -0.6208444 , 0.97340184, -0.22910446], [-0.85283672, -0.52217768, 0.8906646 , -0.45466095]])
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- created_at :
- 2023-08-03T12:44:52.614490
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (date: 179, channel: 2, control: 3, fourier_mode: 4) Coordinates: * date (date) <U10 '2018-04-02' '2018-04-09' ... '2021-08-30' * channel (channel) <U2 'x1' 'x2' * control (control) <U7 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) <U11 'sin_order_1' ... 'cos_order_2' Data variables: channel_data (date, channel) float64 0.3196 0.0 0.1128 ... 0.0 0.4403 0.0 target (date) float64 0.4794 0.4527 0.5374 ... 0.4978 0.5388 0.5625 control_data (date, control) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 178.0 fourier_data (date, fourier_mode) float64 0.9999 -0.01183 ... -0.4547 Attributes: created_at: 2023-08-03T12:44:52.614490 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- index: 179
- index(index)int640 1 2 3 4 5 ... 174 175 176 177 178
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153, 154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164, 165, 166, 167, 168, 169, 170, 171, 172, 173, 174, 175, 176, 177, 178])
- date_week(index)object'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', ... '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype=object)
- x1(index)float640.3186 0.1124 ... 0.2803 0.4389
array([3.18580018e-01, 1.12388476e-01, 2.92400266e-01, 7.13985526e-02, 3.86745154e-01, 4.71709861e-02, 4.24249105e-01, 3.33920175e-01, 2.53070285e-01, 9.38054416e-01, 4.25205073e-01, 3.63142977e-01, 4.41494696e-01, 4.23111626e-01, 3.28392249e-01, 9.21940303e-01, 3.08015512e-01, 9.09485226e-01, 2.50698647e-01, 2.36473273e-01, 4.03137932e-01, 1.47177719e-01, 3.63041014e-01, 1.47066490e-01, 9.46704311e-02, 2.59245305e-01, 5.74838249e-02, 2.06465328e-02, 1.65636049e-01, 2.14007141e-01, 3.60517692e-01, 1.22368556e-01, 9.58683953e-01, 3.21972344e-01, 2.53234055e-01, 6.40119796e-02, 2.36553200e-02, 1.83164569e-01, 9.25302245e-01, 9.96658129e-01, 4.01689634e-01, 1.73745461e-01, 9.55372645e-01, 3.55889419e-01, 9.29181335e-04, 4.37815667e-01, 9.73976316e-01, 9.77903945e-01, 2.48141704e-01, 4.05620409e-01, 2.49040963e-01, 5.30524844e-02, 6.63217207e-02, 9.19314691e-02, 2.84652565e-01, 3.73845327e-01, 3.11033721e-01, 1.08200596e-02, 3.25854693e-01, 4.30160827e-01, 4.42189999e-01, 2.68919050e-01, 3.84406892e-01, 3.03780772e-01, 2.22092463e-01, 9.84087017e-01, 1.78161753e-01, 1.52862615e-01, 4.32812209e-01, 3.80735892e-01, 1.33810881e-01, 2.18715931e-01, 3.24034681e-01, 3.66676692e-01, 1.50298760e-01, 9.31123777e-01, 2.99199062e-01, 1.59348190e-01, 4.49282836e-01, 9.18307179e-03, ... 2.83962083e-01, 4.29271667e-01, 1.55574946e-01, 1.07068213e-01, 4.46958034e-01, 3.53012159e-01, 4.05501510e-01, 9.95101996e-01, 1.95552073e-01, 4.21105629e-01, 3.89127721e-02, 2.74762370e-01, 3.88551689e-01, 3.98177912e-01, 9.30166751e-01, 2.59848823e-01, 1.94820914e-01, 2.58231310e-01, 3.02189662e-01, 1.03497234e-01, 8.01700983e-02, 4.15976836e-01, 3.96173495e-01, 4.43288434e-01, 6.38882192e-02, 2.56961240e-01, 4.16716500e-01, 1.89344301e-01, 1.21168779e-02, 3.07204390e-01, 2.79346139e-01, 1.55859670e-01, 2.51259804e-01, 4.15636348e-01, 1.50413447e-01, 4.18457229e-02, 2.92710243e-01, 3.91623929e-01, 9.89705226e-02, 2.68473040e-01, 3.63484578e-01, 1.85363200e-01, 6.59774982e-02, 3.54568453e-01, 1.59422721e-01, 1.81976239e-01, 1.16747054e-01, 3.23780216e-01, 4.34122877e-01, 1.08988007e-01, 1.61353829e-01, 9.42322052e-01, 8.52032642e-02, 3.25819647e-01, 1.67913280e-01, 3.39621958e-01, 2.52901858e-01, 8.64855399e-02, 3.37226955e-01, 1.69762852e-01, 4.27604907e-01, 9.10434562e-01, 1.42466955e-01, 1.86213914e-01, 3.13371214e-01, 3.99339412e-01, 1.44584335e-01, 1.47975068e-01, 6.98435624e-02, 1.98437898e-01, 3.61488489e-01, 2.40337490e-01, 4.03740331e-02, 6.74570446e-02, 3.30241869e-02, 1.65615150e-01, 1.71882222e-01, 2.80257288e-01, 4.38857161e-01])
- x2(index)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0
array([0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.87978184, 0. , 0. , 0. , 0. , 0. , 0.96418688, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.80062317, 0. , 0. , 0. , 0.8535269 , 0. , 0. , 0.98859712, 0.87047511, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.9748662 , 0. , 0. , 0. , 0.90257287, 0. , 0. , 0. , 0. , 0.99437431, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.95798229, 0. , 0. , 0.88604706, 0.84193305, 0. , 0.91253659, 0. , 0. , 0.99123254, 0. , 0. , 0.95581799, 0.91704461, 0. , 0.80904352, 0. , 0. , 0. , 0.86960149, 0. , 0.9208528 , 0. , 0. , 0. , 0. , 0.99390622, 0. , 0. , 0. , 0. , 0. , 0. , 0.93665676, 0.9093652 , 0. , 0. , 0.80688306, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.89397651, 0.86774976, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.83998152, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.96018382, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.93310233, 0.91533788, 0. , 0. , 0.85070177, 0. , 0. , 0. , 0. , 0. , 0. , 0.90885834, 0. , 0. , 0. , 0. , 0.86334885, 0. , 0. , 0. ])
- event_1(index)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 1., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- event_2(index)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 1., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- dayofyear(index)int6492 99 106 113 ... 221 228 235 242
array([ 92, 99, 106, 113, 120, 127, 134, 141, 148, 155, 162, 169, 176, 183, 190, 197, 204, 211, 218, 225, 232, 239, 246, 253, 260, 267, 274, 281, 288, 295, 302, 309, 316, 323, 330, 337, 344, 351, 358, 365, 7, 14, 21, 28, 35, 42, 49, 56, 63, 70, 77, 84, 91, 98, 105, 112, 119, 126, 133, 140, 147, 154, 161, 168, 175, 182, 189, 196, 203, 210, 217, 224, 231, 238, 245, 252, 259, 266, 273, 280, 287, 294, 301, 308, 315, 322, 329, 336, 343, 350, 357, 364, 6, 13, 20, 27, 34, 41, 48, 55, 62, 69, 76, 83, 90, 97, 104, 111, 118, 125, 132, 139, 146, 153, 160, 167, 174, 181, 188, 195, 202, 209, 216, 223, 230, 237, 244, 251, 258, 265, 272, 279, 286, 293, 300, 307, 314, 321, 328, 335, 342, 349, 356, 363, 4, 11, 18, 25, 32, 39, 46, 53, 60, 67, 74, 81, 88, 95, 102, 109, 116, 123, 130, 137, 144, 151, 158, 165, 172, 179, 186, 193, 200, 207, 214, 221, 228, 235, 242])
- t(index)int640 1 2 3 4 5 ... 174 175 176 177 178
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153, 154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164, 165, 166, 167, 168, 169, 170, 171, 172, 173, 174, 175, 176, 177, 178])
- y(index)float643.985e+03 3.763e+03 ... 4.676e+03
array([3984.66223734, 3762.87179412, 4466.96738844, 3864.21937266, 4441.62527775, 3677.39655018, 5067.54633687, 6079.09904219, 4954.20536859, 5865.67657627, 5096.63305051, 4991.54228314, 4688.5848447 , 6536.34574052, 5030.98358508, 4638.69083472, 4209.54579837, 4876.00838907, 3906.171689 , 3549.86212243, 4025.43395537, 3223.30633321, 4079.65295872, 4156.43557336, 3666.8772267 , 3853.54920598, 3531.47191787, 3538.8824745 , 4261.17449177, 4604.51723377, 6484.97624603, 5090.8724376 , 6500.44070675, 6434.7020032 , 7288.09549867, 5249.09562308, 4284.73710406, 5924.4967947 , 7770.89886433, 6600.77946045, 6003.92292284, 5660.65332884, 5872.74193171, 6093.70178134, 3994.21575864, 4817.41932661, 7795.0978095 , 6447.22166693, 5540.6792271 , 4949.83235925, 6379.8986888 , 4764.01718599, 4207.85140173, 4412.82026656, 4346.99520328, 6626.69831268, 5231.24779003, 3891.28186583, 6815.82014683, 5047.60961099, 5292.31541818, 5238.73239384, 4994.21021861, 4563.9431011 , 4501.26480448, 5634.63844969, 4823.48226687, 4053.07938257, 4489.40147834, 6196.75603817, 3824.9202315 , 3952.72844853, 5958.05509279, 6003.89166699, 4506.60683165, 7107.8687139 , 5686.19380778, 4737.44808553, 7010.95204787, 4830.18985388, ... 4650.33832006, 5271.77236249, 5847.25691642, 6434.12509649, 5835.67243843, 5424.16703016, 6805.503155 , 6808.85054468, 5684.10310247, 5920.17689413, 4508.38691899, 4822.65128872, 5280.52839424, 5215.19196501, 7674.82634251, 7142.73704187, 5179.99140594, 4687.55775271, 4623.09983189, 3538.8645019 , 3580.23476566, 4361.20963926, 4535.40095043, 4376.02791122, 3460.32851473, 4119.38208881, 4450.28600415, 4405.89387854, 6496.50077682, 4442.17560168, 5309.6466714 , 4964.82187487, 4767.04140224, 6007.10694272, 7341.81915474, 5611.66436779, 5675.76416319, 6390.81472343, 5567.94366983, 5850.38866634, 6150.42324303, 5481.44428281, 6971.26166534, 6117.54314155, 4725.84123847, 5163.38572629, 4402.2528145 , 5629.27905386, 5440.85463743, 4747.48354262, 4651.19391143, 5678.17799695, 5327.64323813, 5092.77743153, 4860.96843213, 5312.05880983, 4716.62387739, 4879.90820541, 5246.0963942 , 7059.26575537, 7451.88641636, 7060.8531078 , 5687.37638691, 6868.04144218, 5894.56896391, 5509.52170002, 5039.91969411, 5135.04343508, 4535.6929473 , 4358.34664039, 6457.79858878, 4425.83485865, 3795.15282441, 3192.87959337, 3553.54614781, 5565.50968216, 4137.65148493, 4479.04135141, 4675.97343867])
- indexPandasIndex
PandasIndex(RangeIndex(start=0, stop=179, step=1, name='index'))
<xarray.Dataset> Dimensions: (index: 179) Coordinates: * index (index) int64 0 1 2 3 4 5 6 7 ... 171 172 173 174 175 176 177 178 Data variables: date_week (index) object '2018-04-02' '2018-04-09' ... '2021-08-30' x1 (index) float64 0.3186 0.1124 0.2924 ... 0.1719 0.2803 0.4389 x2 (index) float64 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.8633 0.0 0.0 0.0 event_1 (index) float64 0.0 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0 0.0 event_2 (index) float64 0.0 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0 0.0 dayofyear (index) int64 92 99 106 113 120 127 ... 207 214 221 228 235 242 t (index) int64 0 1 2 3 4 5 6 7 ... 171 172 173 174 175 176 177 178 y (index) float64 3.985e+03 3.763e+03 ... 4.479e+03 4.676e+03
xarray.Dataset
Saving and loading a fitted model#
All the data passed to the model on initialization is stored in idata.attrs
. This will be used later in the save()
method to convert both this data and all the fit data into the netCDF format. You can read more about this format here.
The save
and load
method only require a path to inform where the model should be saved and loaded from.
mmm.save('my_saved_model.nc')
loaded_model = DelayedSaturatedMMM.load('my_saved_model.nc')
/home/ricardo/miniconda3/envs/pymc-marketing/lib/python3.11/site-packages/arviz/data/inference_data.py:152: UserWarning: fit_data group is not defined in the InferenceData scheme
warnings.warn(
loaded_model.model_config["beta_channel"]
{'sigma': array([2.1775326 , 1.14026088]), 'dims': ('channel',)}
loaded_model.graphviz()
loaded_model.idata
-
- chain: 4
- draw: 1000
- control: 3
- fourier_mode: 4
- channel: 2
- date: 179
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- control(control)object'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype=object)
- fourier_mode(fourier_mode)object'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype=object)
- channel(channel)object'x1' 'x2'
array(['x1', 'x2'], dtype=object)
- date(date)object'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype=object)
- intercept(chain, draw)float64...
[4000 values with dtype=float64]
- gamma_control(chain, draw, control)float64...
[12000 values with dtype=float64]
- gamma_fourier(chain, draw, fourier_mode)float64...
[16000 values with dtype=float64]
- beta_channel(chain, draw, channel)float64...
[8000 values with dtype=float64]
- alpha(chain, draw, channel)float64...
[8000 values with dtype=float64]
- lam(chain, draw, channel)float64...
[8000 values with dtype=float64]
- sigma(chain, draw)float64...
[4000 values with dtype=float64]
- channel_adstock(chain, draw, date, channel)float64...
[1432000 values with dtype=float64]
- channel_adstock_saturated(chain, draw, date, channel)float64...
[1432000 values with dtype=float64]
- channel_contributions(chain, draw, date, channel)float64...
[1432000 values with dtype=float64]
- control_contributions(chain, draw, date, control)float64...
[2148000 values with dtype=float64]
- fourier_contributions(chain, draw, date, fourier_mode)float64...
[2864000 values with dtype=float64]
- mu(chain, draw, date)float64...
[716000 values with dtype=float64]
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:52.592010
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
- sampling_time :
- 71.22048568725586
- tuning_steps :
- 1000
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000, control: 3, fourier_mode: 4, channel: 2, date: 179) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 ... 995 996 997 998 999 * control (control) object 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) object 'sin_order_1' ... 'cos_o... * channel (channel) object 'x1' 'x2' * date (date) object '2018-04-02' ... '2021-08-30' Data variables: (12/13) intercept (chain, draw) float64 ... gamma_control (chain, draw, control) float64 ... gamma_fourier (chain, draw, fourier_mode) float64 ... beta_channel (chain, draw, channel) float64 ... alpha (chain, draw, channel) float64 ... lam (chain, draw, channel) float64 ... ... ... channel_adstock (chain, draw, date, channel) float64 ... channel_adstock_saturated (chain, draw, date, channel) float64 ... channel_contributions (chain, draw, date, channel) float64 ... control_contributions (chain, draw, date, control) float64 ... fourier_contributions (chain, draw, date, fourier_mode) float64 ... mu (chain, draw, date) float64 ... Attributes: created_at: 2023-08-03T12:44:52.592010 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0 sampling_time: 71.22048568725586 tuning_steps: 1000
xarray.Dataset -
- chain: 4
- draw: 1000
- date: 179
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- date(date)object'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype=object)
- likelihood(chain, draw, date)float64...
[716000 values with dtype=float64]
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:55.266426
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000, date: 179) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 6 7 ... 992 993 994 995 996 997 998 999 * date (date) object '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (chain, draw, date) float64 ... Attributes: created_at: 2023-08-03T12:44:55.266426 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- chain: 4
- draw: 1000
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- process_time_diff(chain, draw)float64...
[4000 values with dtype=float64]
- lp(chain, draw)float64...
[4000 values with dtype=float64]
- max_energy_error(chain, draw)float64...
[4000 values with dtype=float64]
- step_size(chain, draw)float64...
[4000 values with dtype=float64]
- reached_max_treedepth(chain, draw)bool...
[4000 values with dtype=bool]
- perf_counter_diff(chain, draw)float64...
[4000 values with dtype=float64]
- step_size_bar(chain, draw)float64...
[4000 values with dtype=float64]
- smallest_eigval(chain, draw)float64...
[4000 values with dtype=float64]
- acceptance_rate(chain, draw)float64...
[4000 values with dtype=float64]
- perf_counter_start(chain, draw)float64...
[4000 values with dtype=float64]
- energy_error(chain, draw)float64...
[4000 values with dtype=float64]
- index_in_trajectory(chain, draw)int64...
[4000 values with dtype=int64]
- energy(chain, draw)float64...
[4000 values with dtype=float64]
- largest_eigval(chain, draw)float64...
[4000 values with dtype=float64]
- n_steps(chain, draw)float64...
[4000 values with dtype=float64]
- tree_depth(chain, draw)int64...
[4000 values with dtype=int64]
- diverging(chain, draw)bool...
[4000 values with dtype=bool]
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- created_at :
- 2023-08-03T12:44:52.608151
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
- sampling_time :
- 71.22048568725586
- tuning_steps :
- 1000
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 ... 994 995 996 997 998 999 Data variables: (12/17) process_time_diff (chain, draw) float64 ... lp (chain, draw) float64 ... max_energy_error (chain, draw) float64 ... step_size (chain, draw) float64 ... reached_max_treedepth (chain, draw) bool ... perf_counter_diff (chain, draw) float64 ... ... ... index_in_trajectory (chain, draw) int64 ... energy (chain, draw) float64 ... largest_eigval (chain, draw) float64 ... n_steps (chain, draw) float64 ... tree_depth (chain, draw) int64 ... diverging (chain, draw) bool ... Attributes: created_at: 2023-08-03T12:44:52.608151 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0 sampling_time: 71.22048568725586 tuning_steps: 1000
xarray.Dataset -
- chain: 1
- draw: 500
- channel: 2
- control: 3
- fourier_mode: 4
- date: 179
- chain(chain)int640
array([0])
- draw(draw)int640 1 2 3 4 5 ... 495 496 497 498 499
array([ 0, 1, 2, ..., 497, 498, 499])
- channel(channel)object'x1' 'x2'
array(['x1', 'x2'], dtype=object)
- control(control)object'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype=object)
- fourier_mode(fourier_mode)object'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype=object)
- date(date)object'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype=object)
- beta_channel(chain, draw, channel)float64...
[1000 values with dtype=float64]
- gamma_control(chain, draw, control)float64...
[1500 values with dtype=float64]
- gamma_fourier(chain, draw, fourier_mode)float64...
[2000 values with dtype=float64]
- channel_contributions(chain, draw, date, channel)float64...
[179000 values with dtype=float64]
- intercept(chain, draw)float64...
[500 values with dtype=float64]
- lam(chain, draw, channel)float64...
[1000 values with dtype=float64]
- fourier_contributions(chain, draw, date, fourier_mode)float64...
[358000 values with dtype=float64]
- mu(chain, draw, date)float64...
[89500 values with dtype=float64]
- alpha(chain, draw, channel)float64...
[1000 values with dtype=float64]
- channel_adstock(chain, draw, date, channel)float64...
[179000 values with dtype=float64]
- control_contributions(chain, draw, date, control)float64...
[268500 values with dtype=float64]
- channel_adstock_saturated(chain, draw, date, channel)float64...
[179000 values with dtype=float64]
- sigma(chain, draw)float64...
[500 values with dtype=float64]
- chainPandasIndex
PandasIndex(Index([0], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 490, 491, 492, 493, 494, 495, 496, 497, 498, 499], dtype='int64', name='draw', length=500))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:54.644498
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (chain: 1, draw: 500, channel: 2, control: 3, fourier_mode: 4, date: 179) Coordinates: * chain (chain) int64 0 * draw (draw) int64 0 1 2 3 4 5 ... 495 496 497 498 499 * channel (channel) object 'x1' 'x2' * control (control) object 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) object 'sin_order_1' ... 'cos_o... * date (date) object '2018-04-02' ... '2021-08-30' Data variables: (12/13) beta_channel (chain, draw, channel) float64 ... gamma_control (chain, draw, control) float64 ... gamma_fourier (chain, draw, fourier_mode) float64 ... channel_contributions (chain, draw, date, channel) float64 ... intercept (chain, draw) float64 ... lam (chain, draw, channel) float64 ... ... ... mu (chain, draw, date) float64 ... alpha (chain, draw, channel) float64 ... channel_adstock (chain, draw, date, channel) float64 ... control_contributions (chain, draw, date, control) float64 ... channel_adstock_saturated (chain, draw, date, channel) float64 ... sigma (chain, draw) float64 ... Attributes: created_at: 2023-08-03T12:44:54.644498 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- chain: 1
- draw: 500
- date: 179
- chain(chain)int640
array([0])
- draw(draw)int640 1 2 3 4 5 ... 495 496 497 498 499
array([ 0, 1, 2, ..., 497, 498, 499])
- date(date)object'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype=object)
- likelihood(chain, draw, date)float64...
[89500 values with dtype=float64]
- chainPandasIndex
PandasIndex(Index([0], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 490, 491, 492, 493, 494, 495, 496, 497, 498, 499], dtype='int64', name='draw', length=500))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:54.650068
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (chain: 1, draw: 500, date: 179) Coordinates: * chain (chain) int64 0 * draw (draw) int64 0 1 2 3 4 5 6 7 ... 492 493 494 495 496 497 498 499 * date (date) object '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (chain, draw, date) float64 ... Attributes: created_at: 2023-08-03T12:44:54.650068 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- date: 179
- date(date)object'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype=object)
- likelihood(date)float64...
[179 values with dtype=float64]
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:44:52.612711
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (date: 179) Coordinates: * date (date) object '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (date) float64 ... Attributes: created_at: 2023-08-03T12:44:52.612711 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- date: 179
- channel: 2
- control: 3
- fourier_mode: 4
- date(date)object'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype=object)
- channel(channel)object'x1' 'x2'
array(['x1', 'x2'], dtype=object)
- control(control)object'event_1' 'event_2' 't'
array(['event_1', 'event_2', 't'], dtype=object)
- fourier_mode(fourier_mode)object'sin_order_1' ... 'cos_order_2'
array(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype=object)
- channel_data(date, channel)float64...
[358 values with dtype=float64]
- target(date)float64...
[179 values with dtype=float64]
- control_data(date, control)float64...
[537 values with dtype=float64]
- fourier_data(date, fourier_mode)float64...
[716 values with dtype=float64]
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- channelPandasIndex
PandasIndex(Index(['x1', 'x2'], dtype='object', name='channel'))
- controlPandasIndex
PandasIndex(Index(['event_1', 'event_2', 't'], dtype='object', name='control'))
- fourier_modePandasIndex
PandasIndex(Index(['sin_order_1', 'cos_order_1', 'sin_order_2', 'cos_order_2'], dtype='object', name='fourier_mode'))
- created_at :
- 2023-08-03T12:44:52.614490
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
<xarray.Dataset> Dimensions: (date: 179, channel: 2, control: 3, fourier_mode: 4) Coordinates: * date (date) object '2018-04-02' '2018-04-09' ... '2021-08-30' * channel (channel) object 'x1' 'x2' * control (control) object 'event_1' 'event_2' 't' * fourier_mode (fourier_mode) object 'sin_order_1' ... 'cos_order_2' Data variables: channel_data (date, channel) float64 ... target (date) float64 ... control_data (date, control) float64 ... fourier_data (date, fourier_mode) float64 ... Attributes: created_at: 2023-08-03T12:44:52.614490 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
xarray.Dataset -
- index: 179
- index(index)int640 1 2 3 4 5 ... 174 175 176 177 178
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153, 154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164, 165, 166, 167, 168, 169, 170, 171, 172, 173, 174, 175, 176, 177, 178])
- date_week(index)object'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype=object)
- x1(index)float640.3186 0.1124 ... 0.2803 0.4389
array([3.185800e-01, 1.123885e-01, 2.924003e-01, 7.139855e-02, 3.867452e-01, 4.717099e-02, 4.242491e-01, 3.339202e-01, 2.530703e-01, 9.380544e-01, 4.252051e-01, 3.631430e-01, 4.414947e-01, 4.231116e-01, 3.283922e-01, 9.219403e-01, 3.080155e-01, 9.094852e-01, 2.506986e-01, 2.364733e-01, 4.031379e-01, 1.471777e-01, 3.630410e-01, 1.470665e-01, 9.467043e-02, 2.592453e-01, 5.748382e-02, 2.064653e-02, 1.656360e-01, 2.140071e-01, 3.605177e-01, 1.223686e-01, 9.586840e-01, 3.219723e-01, 2.532341e-01, 6.401198e-02, 2.365532e-02, 1.831646e-01, 9.253022e-01, 9.966581e-01, 4.016896e-01, 1.737455e-01, 9.553726e-01, 3.558894e-01, 9.291813e-04, 4.378157e-01, 9.739763e-01, 9.779039e-01, 2.481417e-01, 4.056204e-01, 2.490410e-01, 5.305248e-02, 6.632172e-02, 9.193147e-02, 2.846526e-01, 3.738453e-01, 3.110337e-01, 1.082006e-02, 3.258547e-01, 4.301608e-01, 4.421900e-01, 2.689191e-01, 3.844069e-01, 3.037808e-01, 2.220925e-01, 9.840870e-01, 1.781618e-01, 1.528626e-01, 4.328122e-01, 3.807359e-01, 1.338109e-01, 2.187159e-01, 3.240347e-01, 3.666767e-01, 1.502988e-01, 9.311238e-01, 2.991991e-01, 1.593482e-01, 4.492828e-01, 9.183072e-03, 2.406173e-01, 3.965522e-02, 6.828151e-02, 2.051752e-01, 4.046401e-01, 4.391195e-01, 2.083842e-01, 3.543588e-02, 9.664747e-01, 3.240292e-01, 4.006693e-01, 1.644249e-01, 2.552044e-01, 3.868456e-01, 4.340460e-01, 2.885776e-01, 3.196821e-01, 2.036868e-01, 3.595476e-01, 2.111223e-02, 2.839621e-01, 4.292717e-01, 1.555749e-01, 1.070682e-01, 4.469580e-01, 3.530122e-01, 4.055015e-01, 9.951020e-01, 1.955521e-01, 4.211056e-01, 3.891277e-02, 2.747624e-01, 3.885517e-01, 3.981779e-01, 9.301668e-01, 2.598488e-01, 1.948209e-01, 2.582313e-01, 3.021897e-01, 1.034972e-01, 8.017010e-02, 4.159768e-01, 3.961735e-01, 4.432884e-01, 6.388822e-02, 2.569612e-01, 4.167165e-01, 1.893443e-01, 1.211688e-02, 3.072044e-01, 2.793461e-01, 1.558597e-01, 2.512598e-01, 4.156363e-01, 1.504134e-01, 4.184572e-02, 2.927102e-01, 3.916239e-01, 9.897052e-02, 2.684730e-01, 3.634846e-01, 1.853632e-01, 6.597750e-02, 3.545685e-01, 1.594227e-01, 1.819762e-01, 1.167471e-01, 3.237802e-01, 4.341229e-01, 1.089880e-01, 1.613538e-01, 9.423221e-01, 8.520326e-02, 3.258196e-01, 1.679133e-01, 3.396220e-01, 2.529019e-01, 8.648554e-02, 3.372270e-01, 1.697629e-01, 4.276049e-01, 9.104346e-01, 1.424670e-01, 1.862139e-01, 3.133712e-01, 3.993394e-01, 1.445843e-01, 1.479751e-01, 6.984356e-02, 1.984379e-01, 3.614885e-01, 2.403375e-01, 4.037403e-02, 6.745704e-02, 3.302419e-02, 1.656152e-01, 1.718822e-01, 2.802573e-01, 4.388572e-01])
- x2(index)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0
array([0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.879782, 0. , 0. , 0. , 0. , 0. , 0.964187, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.800623, 0. , 0. , 0. , 0.853527, 0. , 0. , 0.988597, 0.870475, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.974866, 0. , 0. , 0. , 0.902573, 0. , 0. , 0. , 0. , 0.994374, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.957982, 0. , 0. , 0.886047, 0.841933, 0. , 0.912537, 0. , 0. , 0.991233, 0. , 0. , 0.955818, 0.917045, 0. , 0.809044, 0. , 0. , 0. , 0.869601, 0. , 0.920853, 0. , 0. , 0. , 0. , 0.993906, 0. , 0. , 0. , 0. , 0. , 0. , 0.936657, 0.909365, 0. , 0. , 0.806883, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.893977, 0.86775 , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.839982, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.960184, 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0.933102, 0.915338, 0. , 0. , 0.850702, 0. , 0. , 0. , 0. , 0. , 0. , 0.908858, 0. , 0. , 0. , 0. , 0.863349, 0. , 0. , 0. ])
- event_1(index)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 1., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- event_2(index)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 1., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
- dayofyear(index)int6492 99 106 113 ... 221 228 235 242
array([ 92, 99, 106, 113, 120, 127, 134, 141, 148, 155, 162, 169, 176, 183, 190, 197, 204, 211, 218, 225, 232, 239, 246, 253, 260, 267, 274, 281, 288, 295, 302, 309, 316, 323, 330, 337, 344, 351, 358, 365, 7, 14, 21, 28, 35, 42, 49, 56, 63, 70, 77, 84, 91, 98, 105, 112, 119, 126, 133, 140, 147, 154, 161, 168, 175, 182, 189, 196, 203, 210, 217, 224, 231, 238, 245, 252, 259, 266, 273, 280, 287, 294, 301, 308, 315, 322, 329, 336, 343, 350, 357, 364, 6, 13, 20, 27, 34, 41, 48, 55, 62, 69, 76, 83, 90, 97, 104, 111, 118, 125, 132, 139, 146, 153, 160, 167, 174, 181, 188, 195, 202, 209, 216, 223, 230, 237, 244, 251, 258, 265, 272, 279, 286, 293, 300, 307, 314, 321, 328, 335, 342, 349, 356, 363, 4, 11, 18, 25, 32, 39, 46, 53, 60, 67, 74, 81, 88, 95, 102, 109, 116, 123, 130, 137, 144, 151, 158, 165, 172, 179, 186, 193, 200, 207, 214, 221, 228, 235, 242])
- t(index)int640 1 2 3 4 5 ... 174 175 176 177 178
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153, 154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164, 165, 166, 167, 168, 169, 170, 171, 172, 173, 174, 175, 176, 177, 178])
- y(index)float643.985e+03 3.763e+03 ... 4.676e+03
array([3984.662237, 3762.871794, 4466.967388, 3864.219373, 4441.625278, 3677.39655 , 5067.546337, 6079.099042, 4954.205369, 5865.676576, 5096.633051, 4991.542283, 4688.584845, 6536.345741, 5030.983585, 4638.690835, 4209.545798, 4876.008389, 3906.171689, 3549.862122, 4025.433955, 3223.306333, 4079.652959, 4156.435573, 3666.877227, 3853.549206, 3531.471918, 3538.882474, 4261.174492, 4604.517234, 6484.976246, 5090.872438, 6500.440707, 6434.702003, 7288.095499, 5249.095623, 4284.737104, 5924.496795, 7770.898864, 6600.77946 , 6003.922923, 5660.653329, 5872.741932, 6093.701781, 3994.215759, 4817.419327, 7795.097809, 6447.221667, 5540.679227, 4949.832359, 6379.898689, 4764.017186, 4207.851402, 4412.820267, 4346.995203, 6626.698313, 5231.24779 , 3891.281866, 6815.820147, 5047.609611, 5292.315418, 5238.732394, 4994.210219, 4563.943101, 4501.264804, 5634.63845 , 4823.482267, 4053.079383, 4489.401478, 6196.756038, 3824.920232, 3952.728449, 5958.055093, 6003.891667, 4506.606832, 7107.868714, 5686.193808, 4737.448086, 7010.952048, 4830.189854, 4771.36246 , 5998.830148, 5829.018189, 5882.675999, 7470.547013, 6745.587701, 5989.886239, 4756.549762, 8312.407544, 6954.851763, 8250.292341, 5836.582431, 5742.57082 , 5918.941634, 5338.1905 , 6815.637385, 5733.020944, 4953.495336, 5368.254186, 4222.099205, 4650.33832 , 5271.772362, 5847.256916, 6434.125096, 5835.672438, 5424.16703 , 6805.503155, 6808.850545, 5684.103102, 5920.176894, 4508.386919, 4822.651289, 5280.528394, 5215.191965, 7674.826343, 7142.737042, 5179.991406, 4687.557753, 4623.099832, 3538.864502, 3580.234766, 4361.209639, 4535.40095 , 4376.027911, 3460.328515, 4119.382089, 4450.286004, 4405.893879, 6496.500777, 4442.175602, 5309.646671, 4964.821875, 4767.041402, 6007.106943, 7341.819155, 5611.664368, 5675.764163, 6390.814723, 5567.94367 , 5850.388666, 6150.423243, 5481.444283, 6971.261665, 6117.543142, 4725.841238, 5163.385726, 4402.252814, 5629.279054, 5440.854637, 4747.483543, 4651.193911, 5678.177997, 5327.643238, 5092.777432, 4860.968432, 5312.05881 , 4716.623877, 4879.908205, 5246.096394, 7059.265755, 7451.886416, 7060.853108, 5687.376387, 6868.041442, 5894.568964, 5509.5217 , 5039.919694, 5135.043435, 4535.692947, 4358.34664 , 6457.798589, 4425.834859, 3795.152824, 3192.879593, 3553.546148, 5565.509682, 4137.651485, 4479.041351, 4675.973439])
- indexPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 169, 170, 171, 172, 173, 174, 175, 176, 177, 178], dtype='int64', name='index', length=179))
<xarray.Dataset> Dimensions: (index: 179) Coordinates: * index (index) int64 0 1 2 3 4 5 6 7 ... 171 172 173 174 175 176 177 178 Data variables: date_week (index) object '2018-04-02' '2018-04-09' ... '2021-08-30' x1 (index) float64 0.3186 0.1124 0.2924 ... 0.1719 0.2803 0.4389 x2 (index) float64 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.8633 0.0 0.0 0.0 event_1 (index) float64 0.0 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0 0.0 event_2 (index) float64 0.0 0.0 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0 0.0 dayofyear (index) int64 92 99 106 113 120 127 ... 207 214 221 228 235 242 t (index) int64 0 1 2 3 4 5 6 7 ... 171 172 173 174 175 176 177 178 y (index) float64 3.985e+03 3.763e+03 ... 4.479e+03 4.676e+03
xarray.Dataset
A loaded model is ready to be used for sampling and prediction, making use of the previous fitting results and data if needed.
loaded_model.sample_posterior_predictive(X, extend_idata=True, combined=False, random_seed=rng)
Sampling: [likelihood]
<xarray.Dataset> Dimensions: (chain: 4, draw: 1000, date: 179) Coordinates: * chain (chain) int64 0 1 2 3 * draw (draw) int64 0 1 2 3 4 5 6 7 ... 992 993 994 995 996 997 998 999 * date (date) <U10 '2018-04-02' '2018-04-09' ... '2021-08-30' Data variables: likelihood (chain, draw, date) float64 0.5057 0.4536 ... 0.5621 0.5581 Attributes: created_at: 2023-08-03T12:45:03.555645 arviz_version: 0.15.1 inference_library: pymc inference_library_version: 5.7.0
- chain: 4
- draw: 1000
- date: 179
- chain(chain)int640 1 2 3
array([0, 1, 2, 3])
- draw(draw)int640 1 2 3 4 5 ... 995 996 997 998 999
array([ 0, 1, 2, ..., 997, 998, 999])
- date(date)<U10'2018-04-02' ... '2021-08-30'
array(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', '2018-06-11', '2018-06-18', '2018-06-25', '2018-07-02', '2018-07-09', '2018-07-16', '2018-07-23', '2018-07-30', '2018-08-06', '2018-08-13', '2018-08-20', '2018-08-27', '2018-09-03', '2018-09-10', '2018-09-17', '2018-09-24', '2018-10-01', '2018-10-08', '2018-10-15', '2018-10-22', '2018-10-29', '2018-11-05', '2018-11-12', '2018-11-19', '2018-11-26', '2018-12-03', '2018-12-10', '2018-12-17', '2018-12-24', '2018-12-31', '2019-01-07', '2019-01-14', '2019-01-21', '2019-01-28', '2019-02-04', '2019-02-11', '2019-02-18', '2019-02-25', '2019-03-04', '2019-03-11', '2019-03-18', '2019-03-25', '2019-04-01', '2019-04-08', '2019-04-15', '2019-04-22', '2019-04-29', '2019-05-06', '2019-05-13', '2019-05-20', '2019-05-27', '2019-06-03', '2019-06-10', '2019-06-17', '2019-06-24', '2019-07-01', '2019-07-08', '2019-07-15', '2019-07-22', '2019-07-29', '2019-08-05', '2019-08-12', '2019-08-19', '2019-08-26', '2019-09-02', '2019-09-09', '2019-09-16', '2019-09-23', '2019-09-30', '2019-10-07', '2019-10-14', '2019-10-21', '2019-10-28', '2019-11-04', '2019-11-11', '2019-11-18', '2019-11-25', '2019-12-02', '2019-12-09', '2019-12-16', '2019-12-23', '2019-12-30', '2020-01-06', '2020-01-13', '2020-01-20', '2020-01-27', '2020-02-03', '2020-02-10', '2020-02-17', '2020-02-24', '2020-03-02', '2020-03-09', '2020-03-16', '2020-03-23', '2020-03-30', '2020-04-06', '2020-04-13', '2020-04-20', '2020-04-27', '2020-05-04', '2020-05-11', '2020-05-18', '2020-05-25', '2020-06-01', '2020-06-08', '2020-06-15', '2020-06-22', '2020-06-29', '2020-07-06', '2020-07-13', '2020-07-20', '2020-07-27', '2020-08-03', '2020-08-10', '2020-08-17', '2020-08-24', '2020-08-31', '2020-09-07', '2020-09-14', '2020-09-21', '2020-09-28', '2020-10-05', '2020-10-12', '2020-10-19', '2020-10-26', '2020-11-02', '2020-11-09', '2020-11-16', '2020-11-23', '2020-11-30', '2020-12-07', '2020-12-14', '2020-12-21', '2020-12-28', '2021-01-04', '2021-01-11', '2021-01-18', '2021-01-25', '2021-02-01', '2021-02-08', '2021-02-15', '2021-02-22', '2021-03-01', '2021-03-08', '2021-03-15', '2021-03-22', '2021-03-29', '2021-04-05', '2021-04-12', '2021-04-19', '2021-04-26', '2021-05-03', '2021-05-10', '2021-05-17', '2021-05-24', '2021-05-31', '2021-06-07', '2021-06-14', '2021-06-21', '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='<U10')
- likelihood(chain, draw, date)float640.5057 0.4536 ... 0.5621 0.5581
array([[[0.50566516, 0.45356648, 0.5389822 , ..., 0.54054904, 0.57418503, 0.57218314], [0.47340083, 0.38812494, 0.445333 , ..., 0.54783002, 0.57678985, 0.55433614], [0.45078425, 0.47481834, 0.52336795, ..., 0.48583437, 0.54402159, 0.62762365], ..., [0.49933768, 0.419704 , 0.47986884, ..., 0.49402004, 0.50404859, 0.61688099], [0.49787153, 0.41531368, 0.50999043, ..., 0.53433009, 0.5436098 , 0.66190546], [0.52300674, 0.44989236, 0.54093442, ..., 0.4705328 , 0.56401553, 0.59699231]], [[0.57565075, 0.48360582, 0.55592461, ..., 0.53085245, 0.52804175, 0.59641743], [0.50900123, 0.46273479, 0.48426426, ..., 0.52823336, 0.55964165, 0.63119077], [0.48094354, 0.43684993, 0.53733421, ..., 0.53346568, 0.51910642, 0.57334469], ... [0.45029206, 0.42426587, 0.47254827, ..., 0.53466605, 0.49604829, 0.63250128], [0.47452993, 0.39640331, 0.54026889, ..., 0.45675391, 0.53829855, 0.56891897], [0.47855382, 0.43722599, 0.50371013, ..., 0.56272473, 0.54400911, 0.66146202]], [[0.52665784, 0.52447698, 0.5537308 , ..., 0.5504315 , 0.52033537, 0.5769569 ], [0.46491778, 0.48539251, 0.54424338, ..., 0.54833781, 0.48352203, 0.64304626], [0.4949092 , 0.4592712 , 0.52830432, ..., 0.47177167, 0.48004932, 0.5826424 ], ..., [0.45062791, 0.49008256, 0.51398036, ..., 0.49803019, 0.54941016, 0.61265547], [0.46602225, 0.50207309, 0.51809171, ..., 0.56849337, 0.53895919, 0.61986521], [0.43838947, 0.42215891, 0.52399232, ..., 0.5389761 , 0.56214423, 0.5580903 ]]])
- chainPandasIndex
PandasIndex(Index([0, 1, 2, 3], dtype='int64', name='chain'))
- drawPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 990, 991, 992, 993, 994, 995, 996, 997, 998, 999], dtype='int64', name='draw', length=1000))
- datePandasIndex
PandasIndex(Index(['2018-04-02', '2018-04-09', '2018-04-16', '2018-04-23', '2018-04-30', '2018-05-07', '2018-05-14', '2018-05-21', '2018-05-28', '2018-06-04', ... '2021-06-28', '2021-07-05', '2021-07-12', '2021-07-19', '2021-07-26', '2021-08-02', '2021-08-09', '2021-08-16', '2021-08-23', '2021-08-30'], dtype='object', name='date', length=179))
- created_at :
- 2023-08-03T12:45:03.555645
- arviz_version :
- 0.15.1
- inference_library :
- pymc
- inference_library_version :
- 5.7.0
az.plot_ppc(loaded_model.idata);
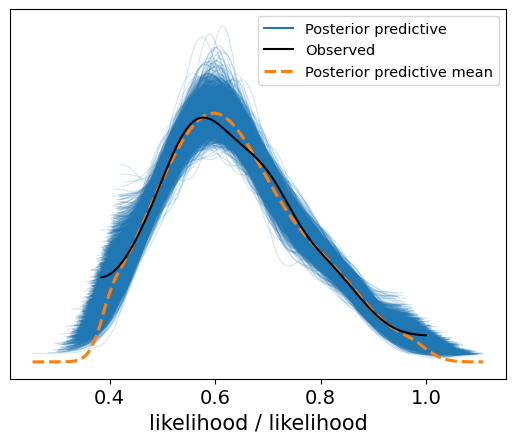
Other models#
Even though this introduction is using DelayedSaturatedMMM
, all other PyMC-Marketing models (MMM and CLV) provide these functionalities as well.
Summary#
The PyMC-Marketing functionalities described here are intended to facilitate model sharing among data science teams without demanding extensive modelling technical knowledge for everyone involved. We are still iterating on our API and would love to hear more feedback from our users!